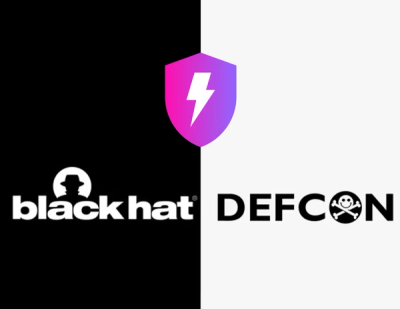
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
jsonpointer.js
Advanced tools
The jsonpointer.js npm package is a utility for handling JSON Pointers, which are a way to reference specific parts of a JSON document. It allows you to get, set, and delete values within a JSON object using a string syntax defined by RFC 6901.
Get Value
This feature allows you to retrieve a value from a JSON object using a JSON Pointer string.
const jsonpointer = require('jsonpointer.js');
const obj = { foo: { bar: 'baz' } };
const value = jsonpointer.get(obj, '/foo/bar');
console.log(value); // 'baz'
Set Value
This feature allows you to set a value in a JSON object at the location specified by a JSON Pointer string.
const jsonpointer = require('jsonpointer.js');
const obj = { foo: { bar: 'baz' } };
jsonpointer.set(obj, '/foo/bar', 'qux');
console.log(obj.foo.bar); // 'qux'
Delete Value
This feature allows you to delete a value from a JSON object at the location specified by a JSON Pointer string.
const jsonpointer = require('jsonpointer.js');
const obj = { foo: { bar: 'baz' } };
jsonpointer.remove(obj, '/foo/bar');
console.log(obj.foo.bar); // undefined
The jsonpointer package provides similar functionality for working with JSON Pointers. It allows you to get, set, and delete values within a JSON object using JSON Pointer syntax. It is widely used and has a similar API to jsonpointer.js.
The json-ptr package is another alternative for handling JSON Pointers. It offers additional features such as resolving pointers against a base document and working with URI fragments. It provides a more comprehensive API compared to jsonpointer.js.
The jsonpath package provides a more powerful querying language for JSON documents, similar to XPath for XML. While it is not limited to JSON Pointers, it offers more advanced querying capabilities, making it suitable for more complex use cases.
FAQs
JavaScript implementation of JSON Pointer
We found that jsonpointer.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.