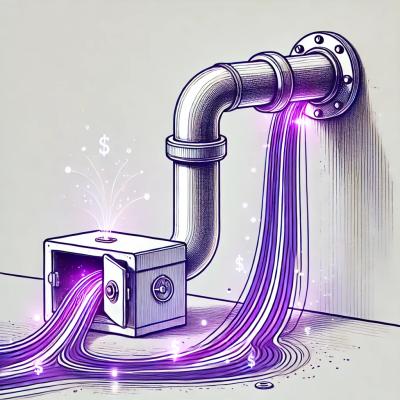
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
jumbo-template
Advanced tools
Jumplate is one of the fastest JS template engine (faster than Jade, Handlebars,...) with precompilation which allows you to cache your template. Execution of precompiled templates is fast as possble because it's clear JavaScript.
Jumplate is one of the fastest (faster than Jade, EJS, Handlebars, Underscore) Node.js template engine with cacheable precompilation. Jumplate is able to compile 16 000 templates in one second and render 400 000 precompiled templates in one second upon one 2,33 GHz core.
Template code is compiled to native JavaScript (can be cached), than it can be rendered with given set of data into final HTML.
Writen in ES6 for Node.js. Babeled for client-side execution. Should run in most recent browsers and IE11 (older should work too but it's not guaranteed).
Example usages of Jumplate.
Creating instance of Jumplate with given template code.
Layout example
<!DOCTYPE html>
<html lang="{{$lang}}">
<head>
<meta charset="UTF-8">
<meta name="description" content="Task manager for managing project development. Work with backlog and tasks in multiple project. Created as DEMO for JumboJS framework.">
<meta name="keywords" content="JumboJS, task, project, backlog, manager">
<meta name="author" content="Roman Jámbor">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="icon" href="/public/favicon.ico">
<link rel="stylesheet" href="/public/styles/default.css">
<script src="/public/uJumbo/ujumbo.js"></script>
<script src="/public/scripts/ujtest.js"></script>
<title>Node Task Manager | JumboJS DEMO</title>
{{include head}}
</head>
<body>
<div class='header{{if $homepage}} homepage{{/if}}'>
<header>
<h1>Node Task Manager</h1>
<h2>Welcome, thanks for giving it a try</h2>
</header>
</div>
<main data-j-snippet="content">
{{include content}}
</main>
</body>
</html>
Partial template example
{{block content}}
<h1>Homepage</h1>
<h2>{{$subtitle}}</h2>
{{if $user.age < 18}}
{{include "../not-allowed.jshtml"}}
{{/if}}
{{* Declare variable inside template *}}
{{var $date = new Date()}}
Current time: <b>{{$date}}</b>
{{* Log $date into browser console *}}
{{debug $date}}
<ul>
{{for $item of $items}}
<li>
{{$item.name}} (My key is: {{$itemKey}})
{{last}}
and I'm the last one
{{/last}}
</li>
{{/for}}
</ul>
<section>
{{!$htmlStyledContent}}
</section>
{{/block}}
Jumplate.registerLocalizator(function(key) {
if (key == "Home.index.text") {
return "Example localized text";
}
return key;
});
var tpl = new Jumplate(
"<body><h1>{{$title}}</h1>{{loc Home.index.text}}</body>");
tpl.compile((error, compiledTemplate) => {
if (error) {
console.error(error.message);
return;
}
// compiledTemplate can be saved to cache here
tpl.render(/* Data */{
title: "Example usage"
}, (error, htmlOutput) => {
if (error) {
console.error(error.message);
return;
}
// You can return htmlOutput to client
console.log(htmlOutput);
})
});
var template = "{{block content}}<h1>{{define title}}{{$title}} with layout{{/define}}</h1>"
+ "{{loc Home.index.text}}{{/block}}";
var layout = "<html><head><title>Example{{defined title}} | {{use title}}{{/defined}}</title>"
+ "</head><body>{{include content}}</body></html>";
var tpl = new Jumplate(template, null, layout);
tpl.compile((error, compiledTemplate) => {
// ... same ...
});
var template = '{{block content}}<h1>{{$title}}</h1>'
+ '{{loc Home.index.text}}{{include "footer.tpl"}}{{/block}}';
var tpl = new Jumplate(template, "path/to/template.tpl");
// Path is used as base dir for resolving include
// and file name is used in errors to specify in which file error occurs
tpl.compile((error, compiledTemplate) => {
// ... same ...
});
var tpl = new Jumplate(null, "path/to/template.tpl", null, "path/to/layout.tpl");
// Path is used as base dir for resolving include
// and file name is used in errors to specify in which file error occurs
tpl.compile((error, compiledTemplate) => {
// ... same ...
});
var tpl = Jumplate.fromCache("compiled cached template code");
tpl.render((error, htmlOutput) => {
// ...
});
All variables begin with $
symbol.
Each variable can be printed as {{$variable}}
. Output has escaped html entities (<, >, &, ", ').
If you want to print variable without escaping use {{!$variable}}
.
It's possible to define variable right inside
template with {{var $x = new Date().toString()}}
Define block of code which is rendered in place of definition.
Can be reused by {{include blockName}}
.
Block can be included many times.
{{block blockName}}Block content. Will be rendered here{{/block}}
Define is similar to block, but define will not be rendered in place of definition.
{{define defineBlockName}}Define block content{{/define}}
Both define
and block
can be checked by {{defined defineBlockName}}Block with name defineBlockName exists.{{/defined}}
.
Include block (define too) or other template file.
{{include blockName}}
will include block with given name.
{{include "./path/to/template.tpl"}}
will include file with given path; relative to current file.
There are two cycles.
{{for $x = 0; $x < $count; $x++}}For content{{/for}}
and
{{for $item of $list}}Foreach content{{/foreach}}
In case of for-of there is special variable $itemKey
which is accessible
inside cycle block and contains key of current item.
In both cycles you can use commands
{{first}}Will be rendered if this iteration is first{{/first}}
{{last}}Will be rendered if this iteration is last{{/last}}
{{even}}Will be rendered if this iteration is even{{/even}}
{{odd}}Will be rendered if this iteration is odd{{/odd}}
{{if $x == "condition"}}
if condition true...
{{elseif $x == "else if condition"}}
if else if condition true...
{{else}}
else...
{{/if}}
You can register localization hadler to Jumplate and
than use command {{loc Key.for.requested.translation}}
which will call your handler
with given key as parameter.
var tpl = new Jumplate(...);
// Register own handler
tpl.registerLocalizator(function(key) {
// Do whatever you want with your key and return translation
})
Jumplate has static methods setLocale
and setTimeZone
to set default locale and timezone which are used in .toLocaleString for Dates eg.
Same methods exist on instance too. Those methods override the static ones.
You can define own helpers, inline and block too. On class Jumplate
there are static methods registerHelper(name, func)
and registerBlockHelper(name, func)
.
Jumplate.registerHelper("link", function(text, url) {
return `<a href="${url}">${text}</a>`;
});
Then you can use it in template like this.
{{block menu}}
{{foreach $item in $menuItems}}
{{link($item.text, $item.url)}}
{{/foreach}}
{{/block}}
{{$createdOn.format("d.m.Y")}}
FAQs
Jumplate is one of the fastest JS template engine (faster than Jade, Handlebars,...) with precompilation which allows you to cache your template. Execution of precompiled templates is fast as possble because it's clear JavaScript.
We found that jumbo-template demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.