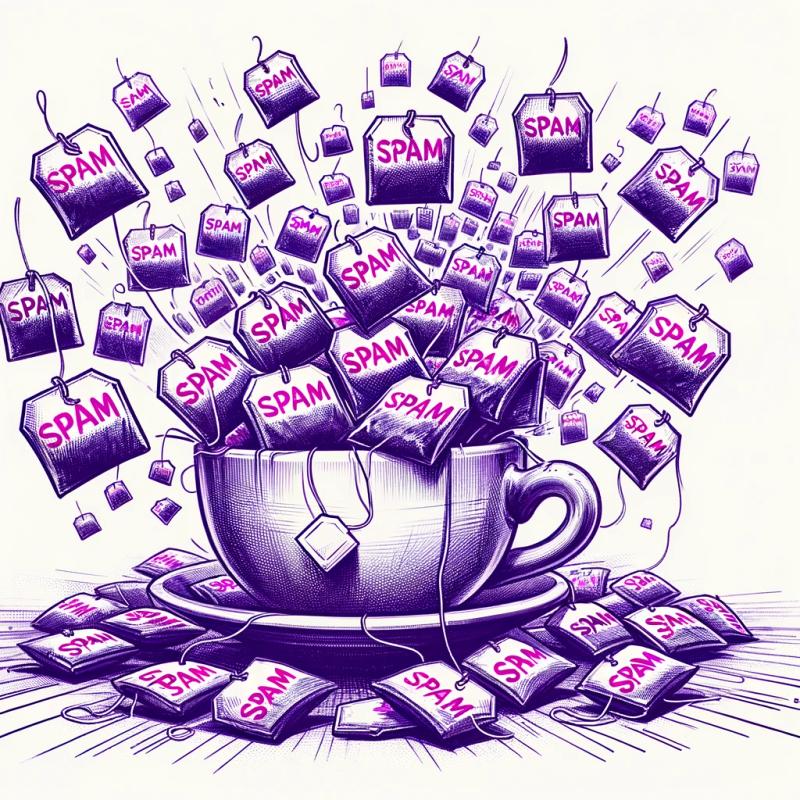
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
karix-api
Advanced tools
Readme
Karix.io API wrapper for Node.js
The Karix.io API V2 Reference is a good resource to learn more about these APIs.
# Using npm
npm install --save karix-api
# Using yarn
yarn add karix-api
const Karix = require("karix-api");
var client = new Karix({
accountId: process.env.KARIX_ACCOUNT_ID
, accountToken: process.env.KARIX_ACCOUNT_TOKEN
// This is optional
, host: process.env.KARIX_HOST || "https://api.karix.io/"
});
client.getSubaccount((err, data) => {
console.log(err || data);
// =>
// {
// "objects": [
// {
// "name": "Beth Smith",
// "status": "enabled",
// "uid": "7fea9708-ea28-42e9-871f-a07fe7cef72f",
// "token": "e664221d-4aed-415b-929b-7edf887e4680",
// "is_parent": false,
// "created_time": "2017-08-04T09:59:29.660Z",
// "updated_time": "2017-08-05T09:59:29.660Z",
// "account_type": "prepaid",
// "credit_balance": "127.33",
// "auto_recharge": false
// }
// ],
// "meta": {
// "request_uuid": "e54b13f5-0831-40f1-959f-e9c5a8ff2957",
// "next": "https://api.karix.co/<resource>/?limit=10&offset=10",
// "previous": "string",
// "total": 1
// }
// }
});
client.getSubaccountById({ uid: "7fea9708-ea28-42e9-871f-a07fe7cef72f" }, (err, data) => {
console.log(err || data);
// =>
// {
// "meta": {
// "request_uuid": "e54b13f5-0831-40f1-959f-e9c5a8ff2957"
// },
// "data": {
// "name": "Beth Smith",
// "status": "enabled",
// "uid": "7fea9708-ea28-42e9-871f-a07fe7cef72f",
// "token": "e664221d-4aed-415b-929b-7edf887e4680",
// "is_parent": false,
// "created_time": "2017-08-04T09:59:29.660Z",
// "updated_time": "2017-08-05T09:59:29.660Z",
// "account_type": "prepaid",
// "credit_balance": "127.33",
// "auto_recharge": false
// }
// }
});
There are few ways to get help:
Please post questions on Stack Overflow. You can open issues with questions, as long you add a link to your Stack Overflow question.
For bug reports and feature requests, open issues. :bug:
For direct and quick help, you can use Codementor. :rocket:
Karix(options)
Creates the instance of the Karix
class.
options
: An object containing:accountId
(String): Karix Account ID (mandatory).accountToken
(String): Karix Account Token (mandatory)host
(String): The karix.io
host (default: https://api.karix.io/
).createSubaccount(data, cb)
Create a new subaccount
data
: The Subaccount object as documented here.cb
: The callback function.getSubaccount(params, cb)
Get a list of details of all subaccounts, including the main account.
params
: The query params as documented here.cb
: The callback function.getSubaccountById(uid, cb)
Get details of an account by its uid. Both main account and subaccounts can be fetched using their uids.
uid
: Alphanumeric ID of the subaccount to get.cb
: The callback function.patchSubaccount(uid, data, cb)
Edit details of your account or its subaccount
uid
: Alphanumeric ID of the subaccount to edit.data
: The Subaccount object (documented here).cb
: The callback function.sendMessage(data, cb)
Send a message to a list of destinations.
data
: The Create Message object data (documented here).cb
: The callback function.getMessage(params, cb)
Get list of messages sent or received. Sorted by descending order of created_time (latest messages are first)
params
: The query params (documented here).cb
: The callback function.getMessageById(uid, cb)
Get message details by id.
uid
: Alphanumeric ID of the message to get.cb
: The callback function.getMedia(uid, cb)
Download or Stream media by id
uid
: Alphanumeric ID of the media to get.cb
: The callback function.createWebhook(data, cb)
Create webhooks to receive Message
data
: The Create Webhook object (documented here).cb
: The callback function.getWebhook(params, cb)
Get a list of your webhooks
params
: The query params (documented here).cb
: The callback function.getWebhookById(uid, cb)
Get a webhook by ID
params
: Alphanumeric ID of the webhook to get.cb
: The callback function.patchWebhook(uid, data, cb)
Edit a webhook
uid
: Alphanumeric ID of the webhook to edit.data
: The request body (documented here).cb
: The callback function.deleteWebhookById(uid, cb)
Delete a webhook by ID
uid
: Alphanumeric ID of the webhook to delete.cb
: The callback function.numberSearch(params, cb)
Query for phone numbers in our inventory.
params
: The query params (documented here).cb
: The callback function.rentNumber(data, cb)
Rent a phone number
data
: The Rent Details object (documented here).cb
: The callback function.getNumber(params, cb)
Get details of all phone numbers linked to your account.
params
: The query params data (documented here).cb
: The callback function.number(number, cb)
Get details of a number
number
: Number for which details need to be fetched.cb
: The callback function.patchNumber(number, data, cb)
Edit phone number belonging to your account
number
: Number which needs to be edited.data
: The request body object (documented here).cb
: The callback function.unrentNumber(number, cb)
Unrent number from your account
number
: Number which needs to be unrented.cb
: The callback function.Have an idea? Found a bug? See how to contribute.
I do web services and open-source my used projects as much as I can. I will try to reply to everyone needing help using these projects. It consumes a lot of time and hardwork. You can integrate and use these projects in your applications for free! You can even change the source code and redistribute (even resell it).
However, if you get some profit from this or just want to encourage me to continue creating stuff, there are few ways you can do it:
—You can make one-time donations via PayPal. I'll probably buy a
coffee tea. :tea:
—Set up a recurring monthly donation and you will get interesting news about what I'm doing (things that I don't share with everyone).
Bitcoin—You can send me bitcoins at this address (or scanning the code below): 344FWmvxDt6FFFoYoFjftiT3gGus68AqNw
Thank you! :heart:
[MIT][license]
FAQs
Karix.io API wrapper for Node.js
The npm package karix-api receives a total of 7 weekly downloads. As such, karix-api popularity was classified as not popular.
We found that karix-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.