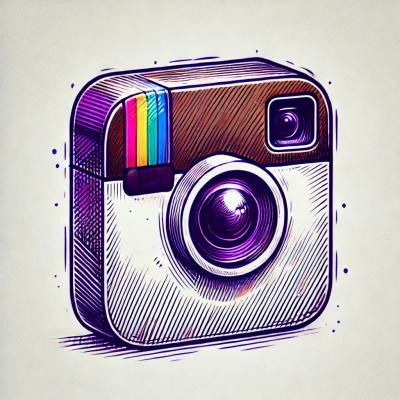
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Keeps track of a server's open sockets so they can be destroyed at a moment's notice.
The killable npm package provides a simple way to make server-like objects (such as those created by `http.createServer`) 'killable'. This means you can easily terminate all active connections with a single method call, allowing for graceful shutdowns of servers. This is particularly useful in scenarios where you need to cleanly stop a server during development, testing, or in production environments for updates or maintenance.
Making a server killable
This feature allows you to wrap an existing server object, making it 'killable'. After calling `killable(server)`, you can call `server.kill()` to terminate all active connections and stop the server.
const killable = require('killable');
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello World!');
});
killable(server);
server.listen(3000, () => console.log('Server running on port 3000'));
// Later, to kill the server and all active connections:
server.kill();
Similar to killable, stoppable also enhances server objects to allow for graceful shutdowns by terminating active connections. However, stoppable provides more fine-grained control over the shutdown process, including the ability to set a timeout for how long to wait for active connections to close before forcibly shutting down.
http-terminator is designed to gracefully terminate HTTP servers by closing existing connections and refusing new ones. It offers a slightly different API compared to killable, focusing on providing a comprehensive solution for shutting down HTTP and HTTPS servers, including support for websockets.
Keeps track of a server's open sockets so they can be destroyed at a moment's notice. This way, the server connection can be killed very fast.
npm install killable
Using express:
('server' in the example is just an http.server
, so other frameworks
or pure Node should work just as well.)
var killable = require('killable');
var app = require('express')();
var server;
app.route('/', function (req, res, next) {
res.send('Server is going down NOW!');
server.kill(function () {
//the server is down when this is called. That won't take long.
});
});
var server = app.listen(8080);
killable(server);
The killable
module is callable. When you call it on a Node
http.Server
object, it will add a server.kill()
method on it. It
returns the server object.
server.kill([callback])
closes all open sockets and calls
server.close()
, to which the callback
is passed on.
Inspired by: http://stackoverflow.com/a/14636625
ISC
FAQs
Keeps track of a server's open sockets so they can be destroyed at a moment's notice.
The npm package killable receives a total of 2,241,686 weekly downloads. As such, killable popularity was classified as popular.
We found that killable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.