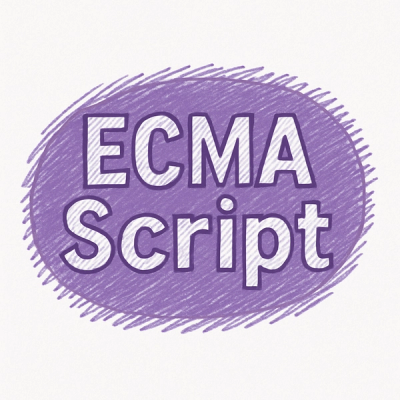
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
kioskboard
Advanced tools
A pure JavaScript library for using virtual keyboards.
2.3.0 *
https://furcan.github.io/KioskBoard/
Chrome
|| Firefox
|| Safari
|| Opera
|| Edge
|| IE 11
yarn add kioskboard
npm i kioskboard
import KioskBoard from 'kioskboard';
<link rel="stylesheet" href="dist/kioskboard-2.3.0.min.css" />
<script src="dist/kioskboard-2.3.0.min.js"></script>
<script src="dist/kioskboard-aio-2.3.0.min.js"></script>
3 types of keyboards can be used: all
, keyboard
, and numpad
.
5 types of themes can be used. light
, dark
, flat
, material
, and oldschool
.
KioskBoard Virtual Keyboard can be used with the input
or textarea
elements.
KioskBoard must be initialized with the required options. The other ones are optional. Keyboard Type (the default value is "all") data-kioskboard-type
, Keyboard Placement (the default value is "bottom") data-kioskboard-placement
, and Special Characters data-kioskboard-specialcharacters
settings are each element-based (data attributes).
All options and examples of data attribute usages are as below. Also, a custom class name can be defined as globally for all input and/or textarea elements to run KioskBoard.
<!-- An example of a textarea element: The keyboard type is "all", the placement is "top", and the availability of the special characters is "true". -->
<textarea class="js-virtual-keyboard" data-kioskboard-type="all" data-kioskboard-placement="top" data-kioskboard-specialcharacters="true" placeholder="Your Address"></textarea>
<!-- An example of an input element: The keyboard type is "keyboard", the placement is "bottom", and the availability of the special characters is "false". -->
<input class="js-virtual-keyboard" data-kioskboard-type="keyboard" data-kioskboard-placement="bottom" data-kioskboard-specialcharacters="false" placeholder="Your Name" />
<!-- An example of an input element: The keyboard type is "numpad", and the placement is "bottom". (Special characters are not allowed for "numpad".) -->
<input class="js-virtual-keyboard" data-kioskboard-type="numpad" data-kioskboard-placement="bottom" placeholder="Your Number" />
// Select the input or the textarea element(s) to run the KioskBoard
KioskBoard.run('.js-virtual-keyboard', {
// ...init options
});
// Initialize KioskBoard (default/all options)
KioskBoard.init({
/*!
* Required
* An Array of Objects has to be defined for the custom keys. Hint: Each object creates a row element (HTML) on the keyboard.
* e.g. [{"key":"value"}, {"key":"value"}] => [{"0":"A","1":"B","2":"C"}, {"0":"D","1":"E","2":"F"}]
*/
keysArrayOfObjects: null,
/*!
* Required only if "keysArrayOfObjects" is "null".
* The path of the "kioskboard-keys-${langugage}.json" file must be set to the "keysJsonUrl" option. (XMLHttpRequest to get the keys from JSON file.)
* e.g. '/Content/Plugins/KioskBoard/dist/kioskboard-keys-english.json'
*/
keysJsonUrl: null,
/*
* Optional: An Array of Strings can be set to override the built-in special characters.
* e.g. ["#", "€", "%", "+", "-", "*"]
*/
keysSpecialCharsArrayOfStrings: null,
/*
* Optional: An Array of Numbers can be set to override the built-in numpad keys. (From 0 to 9, in any order.)
* e.g. [1, 2, 3, 4, 5, 6, 7, 8, 9, 0]
*/
keysNumpadArrayOfNumbers: null,
// Optional: (Other Options)
// Language Code (ISO 639-1) for custom keys (for language support) => e.g. "de" || "en" || "fr" || "hu" || "tr" etc...
language: 'en',
// The theme of keyboard => "light" || "dark" || "flat" || "material" || "oldschool"
theme: 'light',
// Scrolls the document to the top or bottom(by the placement option) of the input/textarea element. Prevented when "false"
autoScroll: true,
// Uppercase or lowercase to start. Uppercased when "true"
capsLockActive: true,
/*
* Allow or prevent real/physical keyboard usage. Prevented when "false"
* In addition, the "allowMobileKeyboard" option must be "true" as well, if the real/physical keyboard has wanted to be used.
*/
allowRealKeyboard: false,
// Allow or prevent mobile keyboard usage. Prevented when "false"
allowMobileKeyboard: false,
// CSS animations for opening or closing the keyboard
cssAnimations: true,
// CSS animations duration as millisecond
cssAnimationsDuration: 360,
// CSS animations style for opening or closing the keyboard => "slide" || "fade"
cssAnimationsStyle: 'slide',
// Enable or Disable Spacebar functionality on the keyboard. The Spacebar will be passive when "false"
keysAllowSpacebar: true,
// Text of the space key (Spacebar). Without text => " "
keysSpacebarText: 'Space',
// Font family of the keys
keysFontFamily: 'sans-serif',
// Font size of the keys
keysFontSize: '22px',
// Font weight of the keys
keysFontWeight: 'normal',
// Size of the icon keys
keysIconSize: '25px',
// Text of the Enter key (Enter/Return). Without text => " "
keysEnterText: 'Enter',
// The callback function of the Enter key. This function will be called when the enter key has been clicked.
keysEnterCallback: undefined,
// The Enter key can close and remove the keyboard. Prevented when "false"
keysEnterCanClose: true,
});
// Select the input or the textarea element(s) to run the KioskBoard
KioskBoard.run('.js-virtual-keyboard');
The keysJsonUrl
option has to be set if custom keys are not defined with the keysArrayOfObjects
option. JSON format has to be: [{"key":"value", "key":"value"}, ...]
. Each object in that array creates a row element (HTML) on the keyboard. The "key" in the objects is used as an "index" for each Keyboard Keys. The "value" is each key's value and inner text.
Additionally, KioskBoard includes 9 different language packages: Arabic
, English
, French
, German
, Hungarian
, Persian
, Russian
, Spanish
, and Turkish
.
An example of a JSON file (for custom keys) in English.
[
{
"0": "Q",
"1": "W",
"2": "E",
"3": "R",
"4": "T",
"5": "Y",
"6": "U",
"7": "I",
"8": "O",
"9": "P"
},
{
"0": "A",
"1": "S",
"2": "D",
"3": "F",
"4": "G",
"5": "H",
"6": "J",
"7": "K",
"8": "L"
},
{
"0": "Z",
"1": "X",
"2": "C",
"3": "V",
"4": "B",
"5": "N",
"6": "M"
}
]
Copyright © 2022 KioskBoard - Virtual Keyboard
MIT license - https://opensource.org/licenses/MIT
FAQs
A pure JavaScript library for using virtual keyboards.
The npm package kioskboard receives a total of 479 weekly downloads. As such, kioskboard popularity was classified as not popular.
We found that kioskboard demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.