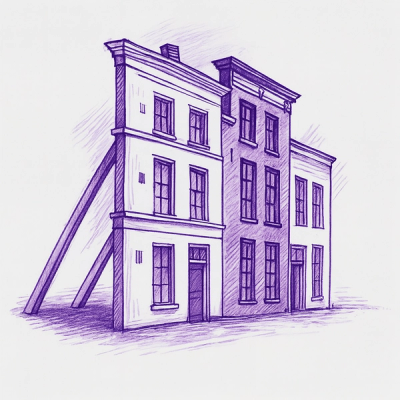
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
A **simple and convenient way** to use **Google Sheets** as a key-value store in **Node.js**. No more messing with heavy databases or hacky file-based storage — just put your data in a Google Sheet and enjoy the ease!
A simple and convenient way to use Google Sheets as a key-value store in Node.js. No more messing with heavy databases or hacky file-based storage — just put your data in a Google Sheet and enjoy the ease!
// index.js
const { createKeyValueStore } = require('kvsheet.js');
(async () => {
// Create the store (reads from .env by default or use inline params)
const store = await createKeyValueStore();
// Set or update values as if it's a normal JS object
store.total = 123;
store.username = 'Alice';
// Commit changes to Google Sheets
await store.save();
// Check what we have
console.log('Now "total" is:', store.total);
console.log('Done!');
})();
Familiar interface (Google Sheets) There’s no need to spin up a separate server or manage a database. Everything lives in a familiar Google Sheet that you and your team can already access and edit.
Lightweight Node.js integration Written in JavaScript for Node.js, it only requires a few lines of code to get a powerful key-value store up and running.
Minimal configuration
Just provide your spreadsheet ID (or URL), the path to your service account JSON, and an optional sheet name. Alternatively, configure them all via .env
for maximum convenience!
Flexible authentication
Use direct key-value pairs (client_email
and private_key
) or point to a service_account.json
file. Both methods are supported out of the box.
Intuitive Proxy wrapper
Access your data like a plain JavaScript object: store.someKey = 123; store.otherKey = "foo"
. Once you’re ready, just run store.save()
to commit everything to Google Sheets.
Automatic ID parsing Pass in a full Google Sheets URL, and kvsheet.js will extract the spreadsheet ID on its own. No extra hassle for your users.
npm install kvsheet.js
(Requires Node.js v12 or higher.)
service_account.json
).xxx@xxx.iam.gserviceaccount.com
.service_account.json
in your project and ignore it in .gitignore
.SHEET_ID=https://docs.google.com/spreadsheets/d/AAAABBBBCCCCDDDD/edit#gid=0
SHEET_NAME=Sheet1
SERVICE_ACCOUNT_CREDENTIALS=./service_account.json
START_X=A
START_Y=1
# Or use direct credentials instead of a JSON file:
GOOGLE_CLIENT_EMAIL=xxx@xxx.iam.gserviceaccount.com
GOOGLE_PRIVATE_KEY=-----BEGIN PRIVATE KEY-----\n...
createKeyValueStore(...)
, kvsheet.js:
A:B
(or any range you specify)._data
.store.save()
, kvsheet.js clears the range in the spreadsheet and writes your updated key-value pairs back.sheetName
empty, kvsheet.js will default to the first sheet in your spreadsheet.startX = 'C'
.1. Do I have to share the spreadsheet with the service account?
Yes. The service account needs "Editor" permission to read and write your spreadsheet.
2. What if I don’t want a .env
file?
No problem. Pass all parameters directly to createKeyValueStore({ ... })
. Using .env
is optional.
3. Can I store more rows than fit on the first 1,000 lines?
Sure. By default, kvsheet.js reads/writes A1:B
. As long as there are enough rows in those columns, it’ll handle them. Google Sheets typically supports up to 10 million cells per spreadsheet.
4. Is it safe to store private keys?
Keep service_account.json
or your private key in a secure location (and in .gitignore
). Avoid committing secrets to public repos.
kvsheet.js is open-sourced under the MIT License, so feel free to use it, modify it, or distribute it in both commercial and personal projects.
npm install kvsheet.js
Join the developers who save time with kvsheet.js. No more over-complication — keep your configs, counters, and lightweight data in a convenient Google Sheet!
Why complicate it? Put your data in Google Sheets and manage it with ease!
We’d love your feedback — star us on GitHub, open an issue, or submit a PR.
Happy coding and enjoy your new spreadsheet-based data store!
FAQs
A **simple and convenient way** to use **Google Sheets** as a key-value store in **Node.js**. No more messing with heavy databases or hacky file-based storage — just put your data in a Google Sheet and enjoy the ease!
The npm package kvsheet.js receives a total of 2 weekly downloads. As such, kvsheet.js popularity was classified as not popular.
We found that kvsheet.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.