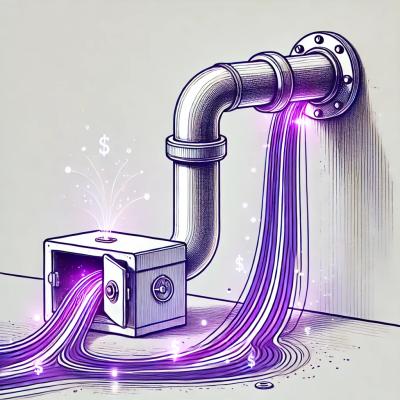
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
lambdaorm
Advanced tools
λORM is an ORM that allows us to perform distributed queries on different database engines.
In λORM, queries are defined using lambda expressions based on a domain model which abstracts us from the infrastructure. For example, in a query you can obtain or modify records from different entities, where some persist in MySQL, others in Postgres, and others in Mongo.
λORM allows you to define different scenarios for the same domain. For example, in one scenario, the infrastructure may consist of distributed instances across SQL Server, MongoDB, and Oracle, while in another scenario it may be a single Postgres instance. This allows the CQRS pattern to be implemented through configuration, without needing to write a single line of code. view example
In addition to being used as a Node.js library, it can be consumed from a command line interface (CLI), a REST service, or a REST service client in other programming languages.
Example of a query where orders and their details associated with a customer are obtained:
const query = (country: string) => Products
.map(p => ({ category: p.category.name, largestPrice: max(p.price) }))
.filter(p => (p.price > 5 && p.supplier.country == country) || (p.inStock < 3))
.having(p => max(p.price) > 50)
.sort(p => desc(p.largestPrice));
// Run the query passing the value of the country parameter
const result = await orm.execute(query, { country: 'ARG' });
In this example:
view: queries | select | join | grouping | include | insert | bulkInsert | update | delete | repository | usage | metadata
The include allows us to obtain the entity data and its relationships in the same query. These data may be in different databases.
In this example the query is expressed as a text string. (Which is another alternative to the lambda expression)
import { orm } from '../../lib'
(async () => {
try {
await orm.init('./config/northwind.yaml')
const query = `Orders
.filter(p => p.id === id)
.include(p =>
[ p.customer.map(p => p.name),
p.details
.include(p =>
p.product
.include(p => p.category.map(p => p.name))
.map(p => p.name))
.map(p => [p.quantity, p.unitPrice])
]
)`
const params = { id: 102 }
const result = await orm.execute(query, params, { stage: 'PostgreSQL' })
console.log(JSON.stringify(result, null, 2))
} catch (error:any) {
console.error(error.message)
} finally {
await orm.end()
}
})()
Result:
[
{
"id": 102,
"customerId": "SPLIR",
"employeeId": 7,
"orderDate": "1996-11-07T23:00:00.000Z",
"requiredDate": "1996-12-05T23:00:00.000Z",
"shippedDate": "1996-11-14T23:00:00.000Z",
"shipViaId": 1,
"freight": 8.63,
"name": "Split Rail Beer & Ale",
"address": "P.O. Box 555",
"city": "Lander",
"region": "WY",
"postalCode": "82520",
"country": "USA",
"customer": {
"name": "Split Rail Beer & Ale"
},
"details": [
{
"quantity": 24,
"unitPrice": 5.9,
"product": {
"name": "Tourtire",
"category": {
"name": "Meat/Poultry"
}
}
}
]
}
]
more info: include
Through the schema, you can define entities, enumerations, indexes, unique keys, default values, constraints, mapping, sources, stages, listeners, etc. The schema can be defined in a JSON or YAML format. Conditions or actions are performed using the same expression language that is used to define queries.
view: schema | definition | use | expressions | environment Variables | composite | listener | multiple stages | multiple sources | push | pull | fetch | introspect | incorporate
Would you like to contribute? Read our contribution guidelines to learn more. There are many ways to help!
Full documentation is available in the Wiki.
You can access various labs at lambdaorm labs
FAQs
ORM
We found that lambdaorm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.