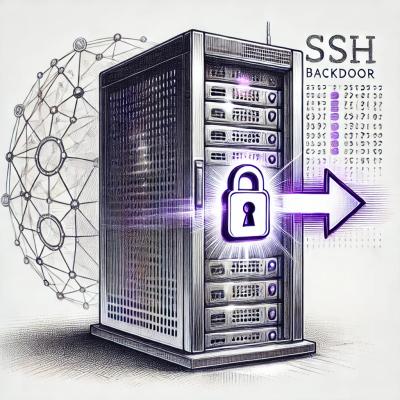
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Connected to ldap server
npm install --save ldap-agent
{
"cn": ["Manager"],
"dc": ["maxcrc", "com"],
"url": "ldap://127.0.0.1:389",
"password": "123456"
}
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
// Add entry
const entry = {
cn: 'foo',
sn: 'bar',
mail: 'sergio@telefonica.com',
objectclass: [
'person',
'organizationalPerson',
'inetOrgPerson',
],
};
return Client.Add(['usuarios'], ['testFolder'], entry, 'mail'); // Send query
})
.then((data) => {
console.log('Field added:', data); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
Client.Init((connectError) => {
// Error handler
if (connectError) {
console.error(`Error connection: ${connectError.message}`);
return;
}
// Connection ready
console.log('Connected to ldap server (callback)');
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
Client.Init()
.then(() => {
console.log('Connected to ldap server (promise)');
})
.catch((connectError) => {
console.error(`Error connection: ${connectError.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
// Delete entry
const condition = {
mail: 'test@telefonica.com',
};
return Client.Delete(condition, ['usuarios'], ['testFolder']); // Send query
})
.then((data) => {
console.log('Field deleted:', data); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
return Client.Find(['usuarios'], ['testFolder'], { attributes: 'sn' }); // Send query
})
.then((data) => {
console.log(`${data.length} fields found!`); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
// Config
const options = {
attributes: 'sn',
filter: {
mail: 'test@telefonica.com',
},
};
return Client.Find(['usuarios'], ['testFolder'], options); // Send query
})
.then((data) => {
console.log('Field found:', data); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
// Config
const options = {
attributes: 'sn',
filter: {
mail: '*@telefonica.com',
sn: 'bar',
},
};
return Client.Find(['usuarios'], ['testFolder'], options); // Send query
})
.then((data) => {
console.log('Fields found:', data.length); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
// Module
const LdapAgent = require('ldap-agent');
// Config
const config = require('./config.json');
// Init client
const Client = new LdapAgent(config);
/* Connection */
// With promise
Client.Init()
.then(() => {
console.log('Connected to ldap server'); // Connected
// Update params
const update = {
sn: 'New value',
};
const where = {
mail: 'test@telefonica.com',
};
return Client.Update(update, where, ['usuarios'], ['testFolder']); // Send query
})
.then((data) => {
console.log('Field updated:', data); // Query success
})
.catch((errorGeneric) => {
console.error(`Error generic: ${errorGeneric.message}`);
});
FAQs
Connected to ldap server
We found that ldap-agent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.