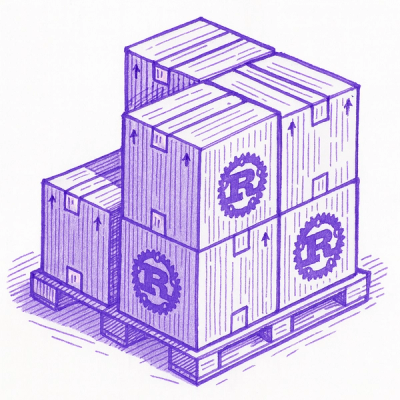
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
请查看 example 文件夹。
请访问 https://github.com/zhaotoday/iview。
账号:admin
密码:123456
注:按 F12 打开 Chrome 开发者工具查看接口请求。
Koa2 使用了 async/await 等新语法,请保证 Node.js 版本在 7.6 及以上。
修改 package.json,将 {app-name} 改成自己的:
{
...,
"scripts": {
"stop": "pm2 stop {app-name}"
},
...
}
修改 processes.json,将 {app-name} 改成自己的:
{
"apps": [
{
"name": "{app-name}",
...
},
...
]
}
# 安装 pm2 到全局
$ npm install -g pm2
# 安装 less.js
$ npm install --save less.js
# JS 代码校验
$ npm run eslintfix
$ npm run eslint
# 开发调试
$ npm run dev
# 启动项目
$ npm run start:dev
# 同步数据库模型
$ npm run sync-models:dev
# 停止项目
$ npm run stop
注::dev 表示执行命令时调用的是开发环境的配置。dev 表示开发环境,test 表示测试环境,beta 表示预生产环境,prod 表示生产环境。
├─ src 源码
│ ├─ app 业务代码
│ │ ├─ controllers 控制器:用于解析用户输入,处理后返回相应的结果
│ │ ├─ models 模型 :用于定义数据模型
│ │ └─ services 服务 :用于编写业务逻辑层,比如连接数据库,调用第三方接口等
│ │
│ ├─ config 配置
│ ├─ extends 扩展
│ ├─ middlewares 中间件
│ ├─ public 静态资源
│ ├─ redis Redis 数据库
│ ├─ router URL 路由
│ ├─ utils 工具库
│ └─ index.js 入口:用于自定义启动时的初始化工作,比如启动 https,调用中间件、路由等
│
├─ .eslintrc eslint 配置文件
├─ nodemon.json nodemon 配置文件
├─ package.json npm 配置文件
├─ processes.json pm2 配置文件
├─ config 配置
│ ├─ base.js 基础配置
│ ├─ development.js 开发环境配置
│ ├─ test.js 测试环境配置
│ ├─ beta.js 预生产环境配置
│ └─ production.js 生产环境配置
├─ extends 扩展
│ ├─ controller.js 对控制器进行扩展
│ ├─ helpers.js 对辅助函数进行扩展
│ ├─ initialize.js 初始化
│ └─ service.js 对服务进行扩展
├─ controllers 控制器
│ └─ v1 接口版本
│ ├─ admin 管理接口
│ │ ├─ actions 动作类接口
│ │ │
│ │ ├─ articles.js articles 接口控制器
│ │ └─ files.js files 接口控制器
│ │
│ ├─ public 公开接口(无需鉴权即可调用)
│ │
│ └─ some-app 某个应用的接口
对 Koa.js 的一些扩展,用 $ 前缀命名,与 Koa.js 内置对象做区分。
app.$config:配置
app.$module:加载内置模块
app.$helpers:辅助函数
app.$resources:生成 RESTful 规范的路由
app.$model:公用模型对象
app.$Service:服务基类
app.$Controller:控制器基类
app.$models:模型集合
app.$services:服务集合
app.$controllers:控制器集合
如扩展辅助函数,请新建 src/extends/helpers.js:
module.exports = app => {
return {
myFunc () {}
}
}
src/app/models/articles.js
module.exports = app => {
const { STRING, TEXT, INTEGER } = app.$Sequelize
return app.$model.define('articles', {
id: {
type: INTEGER(10).UNSIGNED,
primaryKey: true,
autoIncrement: true,
allowNull: false,
comment: 'ID'
},
title: {
type: STRING(200),
allowNull: false,
comment: '标题'
},
content: {
type: TEXT('long'),
comment: '内容'
}
})
}
src/app/services/articles.js
module.exports = app => {
return class extends app.$Service {
constructor () {
super()
this.model = app.$models.articles
}
}
}
src/app/controllers/api/v1/public/articles.js
module.exports = app => {
const service = app.$services.articles
return class extends app.$Controller {
async index (ctx, next) {
ctx.send({
status: 200,
data: await service.find({ offset: 0, limit: 10 })
})
}
}
}
src/router/index.js
const router = require('koa-router')()
module.exports = app => {
router.get('/', app.$controllers.web.home.index)
router.get('/articles/:id?', app.$controllers.web.articles.index)
app.use(router.routes()).use(router.allowedMethods())
}
FAQs
A write less, do more MVC framework based on Koa.
The npm package less.js receives a total of 82 weekly downloads. As such, less.js popularity was classified as not popular.
We found that less.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.