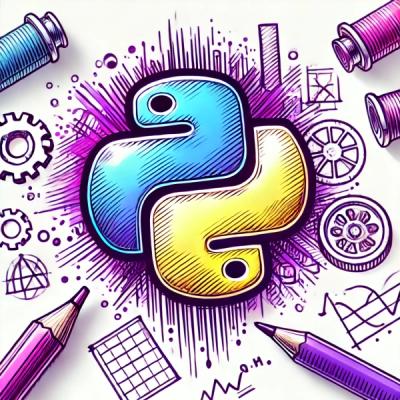
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
livereload
Advanced tools
An implementation of the LiveReload server in Node.js. It's an alternative to the graphical http://livereload.com/ application, which monitors files for changes and reloads your web browser.
You can use this by using the official browser extension or by adding JavaScript code to your page.
Install the LiveReload browser plugins by visiting http://help.livereload.com/kb/general-use/browser-extensions.
Note: Only Google Chrome supports viewing file:///
URLS, and you have to specifically enable it. If you are using other browsers and want to use file:///
URLs, add the JS code to the page as shown in the next section.
Once you have the plugin installed, start livereload
. Then, in the browser, click the LiveReload icon to connect the browser to the server.
Add this code:
<script>
document.write('<script src="http://' + (location.host || 'localhost').split(':')[0] +
':35729/livereload.js?snipver=1"></' + 'script>')
</script>
Note: If you are using a different port other than 35729
you will
need to change the above script.
You can run LiveReload two ways: using the CLI application or by writing your own server using the API.
To use livereload from the command line:
$ npm install -g livereload
$ livereload [path] [options]
To watch files in the current directory for changes and use the default extensions, run this command:
$ livereload
To watch files in another directory, specify its path:
$ livereload ~/website
The commandline options are
-p
or --port
to specify the listening port-d
or --debug
to show debug messages when the browser reloads.-e
or --exts
to specify extentions that you want to observe. Example: -e 'jade,scss'
. Removes the default extensions.-ee
or --extraExts
to include additional extentions that you want to observe. Example: -ee 'jade,scss'
.-x
or --exclusions
to specify additional exclusion patterns. Example: -x html, images/
.-u
or --usepolling
to poll for file system changes. Set this to true to successfully watch files over a network.-w
or --wait
to add a delay (in miliseconds) between when livereload detects a change to the filesystem and when it notifies the browser.-op
or --originalpath
to set a URL you use for development, e.g 'http:/domain.com', then LiveReload will proxy this url to local path.For example, to use a wait time and turn on debugging so you can see messages in your terminal, execute livereload
like this:
$ livereload -w 1000 -d
To turn on debugging and tell Livereload to only look at HTML files in the public
directory, run it like this:
$ livereload public/ -e 'html'
The file path can be at any place in the arguments. For example, you can put it at the end if you wish:
$ livereload -e 'html' public/
Finally, you can tell LiveReload to refresh the browser when specific filenames change. This is useful when there are files that don't have extensions, or when you want to exclude all HTML files except for index.html
throughout the project. Use the -f
or --filesToReload
option:
$ livereload -f 'index.html' public/
All changes to index.html
in any subdirectory will cause LiveReload to send the reload message.
To use the api within a project:
$ npm install livereload --save
Then, create a server and fire it up.
var livereload = require('livereload');
var server = livereload.createServer();
server.watch(__dirname + "/public");
You can also use this with a Connect server. Here's an example of a simple server
using connect
and a few other modules just to give you an idea:
var connect = require('connect');
var compiler = require('connect-compiler');
var static = require('serve-static');
var server = connect();
server.use(
compiler({
enabled : [ 'coffee', 'uglify' ],
src : 'src',
dest : 'public'
})
);
server.use( static(__dirname + '/public'));
server.listen(3000);
var livereload = require('livereload');
var lrserver = livereload.createServer();
lrserver.watch(__dirname + "/public");
You can then start up the server which will listen on port 3000
.
The createServer()
method accepts two arguments.
The first are some configuration options, passed as a JavaScript object:
https
is an optional object of options to be passed to https.createServer (if not provided, http.createServer
is used instead)port
is the listening port. It defaults to 35729
which is what the LiveReload extensions use currently.exts
is an array of extensions you want to observe. This overrides the default extensions of [
html,
css,
js,
png,
gif,
jpg,
php,
php5,
py,
rb,
erb,
coffee]
.extraExts
is an array of extensions you want to observe. The default extensions are [
html,
css,
js,
png,
gif,
jpg,
php,
php5,
py,
rb,
erb,
coffee]
.applyCSSLive
tells LiveReload to reload CSS files in the background instead of refreshing the page. The default for this is true
.applyImgLive
tells LiveReload to reload image files in the background instead of refreshing the page. The default for this is true
. Namely for these extensions: jpg, jpeg, png, gifexclusions
lets you specify files to ignore. By default, this includes .git/
, .svn/
, and .hg/
originalPath
Set URL you use for development, e.g 'http:/domain.com', then LiveReload will proxy this url to local path.overrideURL
lets you specify a different host for CSS files. This lets you edit local CSS files but view a live site. See http://feedback.livereload.com/knowledgebase/articles/86220-preview-css-changes-against-a-live-site-then-uplo for details.usePolling
Poll for file system changes. Set this to true
to successfully watch files over a network.delay
add a delay (in miliseconds) between when livereload detects a change to the filesystem and when it notifies the browser. Useful if the browser is reloading/refreshing before a file has been compiled, for example, by browserify.noListen
Pass as true
to indicate that the websocket server should not be started automatically. (useful if you want to start it yourself later)The second argument is an optional callback
that will be sent to the LiveReload server and called for the listening
event. (ie: when the server is ready to start accepting connections)
Passing an array of paths or glob patterns will allow you to watch multiple directories. All directories have the same configuration options.
server.watch([__dirname + "/js", __dirname + "/css"]);
Command line:
$ livereload "path1, path2, path3"
originalPath
optionYou can map local CSS files to a remote URL. If your HTML file specifies live CSS files at example.com
like this:
<!-- html -->
<head>
<link rel="stylesheet" href="http://domain.com/css/style.css">
</head>
Then you can tell livereload to substitute a local CSS file instead:
// server.js
var server = livereload.createServer({
originalPath: "http://domain.com"
});
server.watch('/User/Workspace/test');
Then run the server:
$ node server.js
When /User/Workspace/test/css/style.css
is modified, the stylesheet will be reloaded on the page.
If you're using file:///
urls, make sure the browser extension is configured to access local files. Alternatively, embed the livereload.js
script on your page as shown in this README.
Your editor is most likely using a swapfile, and when you save, there's a split second where the existing file is deleted from the file system before the swap file is saved in its place. This happens with Vim. You can disable swapfiles in your editor, or you can add a slight delay to Livereload using the -w
option on the command line.
This library is implemented in CoffeeScript 1.x. It may eventually be converted to JavaScript, but because there are many projects that depend on this library, the conversion isn't a priority.
To build the distributable versions, run npm run build
.
Run npm test
to run the test suite.
Contributions welcome, but remember that this library is meant to be small and serve its intended purpose only. Before submitting a pull request, open a new issue to discuss your feature or bug. Please check all open and closed issues.
When submitting code, please keep commits small, and do not modify the README file. Commit both the Coffee and JS files.
filesToReload
option to specify a list of filenames that should trigger the reload, rather than relying on extensions alone.-f
or --filesToReload
option with the command line tool to specifiy filenames that should trigger a reload.-op
or --originalpath
option with the command line tool instead of writing your own server.Cakefile
as Cake is no longer needed. Use npm run tests
and npm run build
instead.chokidar
dependency to 3.5.1livereload-js
dependency to 3.3.1ws
dependency to 7.4.3cake
tasks for building the project.input
message from clientsws
dependency to v6.2.1 to close security vulnerabilityexts
and e
options now replace the default extensions.extraExts
and ee
options to preserve the old behavior of adding extensions to watch.server.on 'error'
in your code to catch the "port in use" message gracefully. The CLI now handles this nicely as well.watcher
object is actually defined before attempting to close.exts
option. In the next version, extensions you specify on the command line will OVERRIDE the default extensions. We'll add a new option for adding your exts to the defaults.noListen
optionws
librarydelay
optionws
libraryusePolling
optionOlder version history not kept.
Copyright (c) 2010-2021 Brian P. Hogan and Joshua Peek
Released under the MIT license. See LICENSE
for details.
FAQs
LiveReload server
We found that livereload demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.