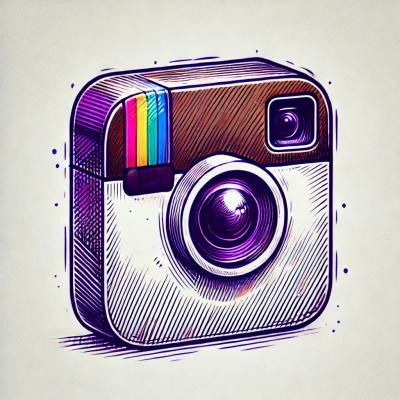
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
lodash.includes
Advanced tools
Supply Chain Security
Vulnerability
Quality
Maintenance
License
The lodash.includes package is a utility library that provides a method to check if a value is present in a collection (array, string, or object). It is a part of the larger Lodash library, which is known for its utility functions for common programming tasks.
Check if a value is in an array
This feature allows you to check if a specific value is present in an array. In the example, it checks if the number 3 is in the array [1, 2, 3, 4].
const includes = require('lodash.includes');
const array = [1, 2, 3, 4];
const result = includes(array, 3);
console.log(result); // true
Check if a value is in a string
This feature allows you to check if a substring is present in a string. In the example, it checks if the substring 'world' is in the string 'hello world'.
const includes = require('lodash.includes');
const string = 'hello world';
const result = includes(string, 'world');
console.log(result); // true
Check if a value is in an object
This feature allows you to check if a value is present in an object. In the example, it checks if the value 2 is in the object { 'a': 1, 'b': 2, 'c': 3 }.
const includes = require('lodash.includes');
const object = { 'a': 1, 'b': 2, 'c': 3 };
const result = includes(object, 2);
console.log(result); // true
Underscore is a JavaScript library that provides utility functions for common programming tasks. It includes a method called 'contains' which is similar to lodash.includes. However, Lodash is generally considered to be faster and more feature-rich compared to Underscore.
Ramda is a functional programming library for JavaScript. It provides a method called 'includes' which is similar to lodash.includes. Ramda focuses more on functional programming paradigms and immutability, whereas Lodash provides a broader range of utility functions.
array-includes is a small, focused package that provides a method to check if a value is present in an array. It is similar to lodash.includes but is limited to arrays only. It is a lightweight alternative if you only need to work with arrays.
The lodash method _.includes
exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save lodash.includes
In Node.js:
var includes = require('lodash.includes');
See the documentation or package source for more details.
FAQs
The lodash method `_.includes` exported as a module.
The npm package lodash.includes receives a total of 13,359,737 weekly downloads. As such, lodash.includes popularity was classified as popular.
We found that lodash.includes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.