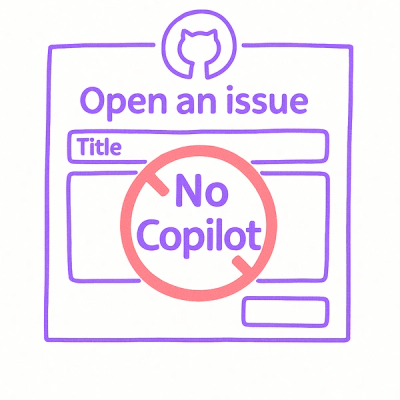
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Adapted from purescript-logging
fp-ts
and TypeScript compatibilitylogging-ts version | required fp-ts version | required TypeScript version |
---|---|---|
0.3.0 | 2.0.0+ | 3.5+ |
0.2.0 | 1.7.0+ | 2.8.0+ |
From purescript-logging
's README
A logger receives records and potentially performs some effects. You can create a logger from any function
(a: A) => HKT<M, void>
for anyA
andM
.Unlike most other logging libraries,
logging-ts
has no separate concepts "loggers" and "handlers". Instead, loggers can be composed into larger loggers using theSemigroup
instance. Loggers can also be transformed usingcontramap
(for transforming records) andfilter
(for filtering records). An example use case might be the following:
import * as C from 'fp-ts/lib/Console'
import * as D from 'fp-ts/lib/Date'
import { chain, IO } from 'fp-ts/lib/IO'
import { pipe } from 'fp-ts/lib/pipeable'
import * as L from '../src/IO'
type Level = 'Debug' | 'Info' | 'Warning' | 'Error'
interface Entry {
message: string
time: Date
level: Level
}
function showEntry(entry: Entry): string {
return `[${entry.level}] ${entry.time.toLocaleString()} ${entry.message}`
}
function getLoggerEntry(prefix: string): L.LoggerIO<Entry> {
return entry => C.log(`${prefix}: ${showEntry(entry)}`)
}
const debugLogger = L.filter(getLoggerEntry('debug.log'), e => e.level === 'Debug')
const productionLogger = L.filter(getLoggerEntry('production.log'), e => e.level !== 'Debug')
const logger = L.getMonoid<Entry>().concat(debugLogger, productionLogger)
const info = (message: string) => (time: Date): IO<void> => logger({ message, time, level: 'Info' })
const debug = (message: string) => (time: Date): IO<void> => logger({ message, time, level: 'Debug' })
const program = pipe(
D.create,
chain(info('boot')),
chain(() => D.create),
chain(debug('Hello!'))
)
program()
/*
production.log: [Info] 10/4/2019, 12:44:48 PM boot
debug.log: [Debug] 10/4/2019, 12:44:48 PM Hello!
*/
0.3.4
IO
withLogger
combinator, #21 (@waynevanson)FAQs
Composable loggers for TypeScript
The npm package logging-ts receives a total of 8,333 weekly downloads. As such, logging-ts popularity was classified as popular.
We found that logging-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.