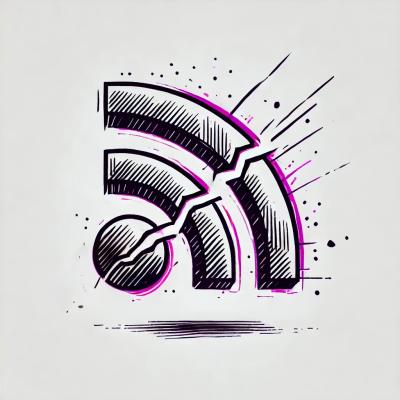
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
lt-portal-menu
Advanced tools
一个轻量级的门户菜单组件,不依赖任何前端框架,可以在 Vue、React 或原生 JavaScript 项目中使用。
npm install lt-portal-menu
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<title>LT Portal Menu Demo</title>
<link rel="stylesheet" href="node_modules/lt-portal-menu/dist/index.css" />
</head>
<body>
<div id="portal-trigger">点击显示菜单</div>
<script src="node_modules/lt-portal-menu/dist/index.js"></script>
<script>
// 菜单数据
const menuData = [
{
id: "1",
title: "常用系统",
submenus: [
{ id: "1-1", text: "告警中心", url: "#", iconUrl: "" },
{ id: "1-2", text: "交通事件管理", url: "#", iconUrl: "" },
{ id: "1-3", text: "视频综合管理", url: "#", iconUrl: "" },
],
},
{
id: "2",
title: "监控一体化",
submenus: [
{ id: "2-1", text: "告警中心", url: "#", iconUrl: "" },
{ id: "2-2", text: "交通事件管理", url: "#", iconUrl: "" },
],
},
];
// 初始化菜单
const portalMenu = new LTPortalMenu({
el: "#portal-trigger",
placement: "bottom-end",
menuData: menuData,
onOpen: function () {
console.log("菜单已打开");
},
onClose: function () {
console.log("菜单已关闭");
},
});
</script>
</body>
</html>
<template>
<div>
<div ref="portalTrigger">打开菜单</div>
</div>
</template>
<script>
import LTPortalMenu from "lt-portal-menu";
import "lt-portal-menu/dist/index.css";
export default {
data() {
return {
portalMenu: null,
menuData: [
{
id: "1",
title: "常用系统",
submenus: [
{ id: "1-1", text: "告警中心", url: "#", iconUrl: "" },
{ id: "1-2", text: "交通事件管理", url: "#", iconUrl: "" },
],
},
],
};
},
mounted() {
this.portalMenu = new LTPortalMenu({
el: this.$refs.portalTrigger,
placement: "bottom-end",
menuData: this.menuData,
});
},
beforeUnmount() {
// 组件销毁时清理资源
if (this.portalMenu) {
this.portalMenu.destroy();
}
},
};
</script>
import { useEffect, useRef } from "react";
import LTPortalMenu from "lt-portal-menu";
import "lt-portal-menu/dist/index.css";
function PortalMenuDemo() {
const triggerRef = useRef(null);
const menuRef = useRef(null);
const menuData = [
{
id: "1",
title: "常用系统",
submenus: [
{ id: "1-1", text: "告警中心", url: "#", iconUrl: "" },
{ id: "1-2", text: "交通事件管理", url: "#", iconUrl: "" },
],
},
];
useEffect(() => {
if (triggerRef.current) {
menuRef.current = new LTPortalMenu({
el: triggerRef.current,
placement: "bottom-end",
menuData: menuData,
});
}
return () => {
if (menuRef.current) {
menuRef.current.destroy();
}
};
}, []);
return (
<div>
<div ref={triggerRef}>打开菜单</div>
</div>
);
}
export default PortalMenuDemo;
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
el | HTMLElement | string | null | 触发器元素或 CSS 选择器 |
placement | string | 'bottom-end' | 菜单位置,可选:'top-start', 'top-end', 'bottom-start', 'bottom-end' |
menuData | Array | [] | 菜单数据 |
onOpen | Function | null | 菜单打开时的回调函数 |
onClose | Function | null | 菜单关闭时的回调函数 |
icons | Object | null | 自定义图标配置 |
icons.trigger | string | null | 自定义触发器图标 URL |
icons.setting | string | null | 自定义设置图标 URL |
icons.close | string | null | 自定义关闭图标 URL |
icons.default | string | null | 自定义默认菜单项图标 URL |
[
{
id: "1", // 菜单ID
title: "分类标题", // 菜单分类标题
submenus: [
// 子菜单列表
{
id: "1-1", // 子菜单ID
text: "菜单名称", // 子菜单名称
url: "链接地址", // 子菜单链接
iconUrl: "图标地址", // 子菜单图标
},
],
},
];
方法名 | 说明 |
---|---|
toggle() | 切换菜单显示状态 |
open() | 打开菜单 |
close() | 关闭菜单 |
renderMenuData(menuData) | 重新渲染菜单数据 |
destroy() | 销毁实例,清理资源 |
您可以通过两种方式自定义图标:
icons
选项提供自定义图标 URL:const portalMenu = new LTPortalMenu({
el: "#trigger",
menuData: menuData,
icons: {
trigger: "path/to/your-trigger-icon.png",
setting: "path/to/your-setting-icon.png",
close: "path/to/your-close-icon.png",
default: "path/to/your-default-icon.png",
},
});
iconUrl
:const menuData = [
{
id: "1",
title: "系统菜单",
submenus: [
{
id: "1-1",
text: "菜单项",
url: "#",
iconUrl: "path/to/your-custom-icon.png",
},
],
},
];
组件使用lt-
前缀命名空间,确保不会与您项目中的其他样式冲突。所有 CSS 类都有明确的前缀,如:
.lt-portal-menu-container {
...;
}
.lt-portal-menu {
...;
}
.lt-portal-menu-header {
...;
}
支持现代浏览器,包括 Chrome、Firefox、Safari、Edge 等。
FAQs
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.