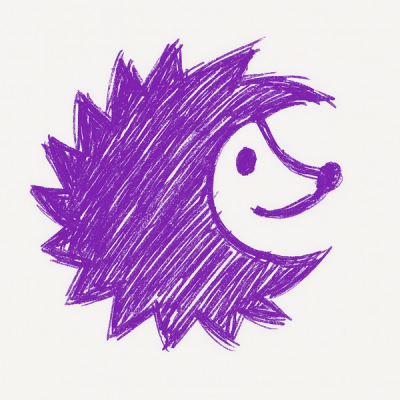
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
make-error
Advanced tools
The make-error package is a simple library for creating custom error types in JavaScript. It is useful for defining new error constructors that work similarly to native Error types, allowing for better error handling and debugging in applications.
Creating custom error types
This feature allows developers to create their own error types that can be used throughout their codebase. The custom error types will inherit from the base Error class, making them compatible with standard error handling mechanisms.
const makeError = require('make-error');
const CustomError = makeError('CustomError');
throw new CustomError('This is a custom error message.');
Extending custom error types
Developers can also extend their custom error types to create a hierarchy of errors. This is useful for creating more specific error types that still share common behavior with their parent error types.
const makeError = require('make-error');
const BaseError = makeError('BaseError');
const SpecificError = makeError(BaseError);
throw new SpecificError('This is a more specific error message.');
The http-errors package allows for the creation of HTTP error objects. It is similar to make-error but is specifically tailored for HTTP server use, providing a list of constructors for various HTTP status codes.
Verror is a library for richer JavaScript errors, which can include multiple error causes, formatted error messages, and other metadata. It offers more features compared to make-error, which is more minimalistic.
Extendable-error is a simple base class for creating extendable custom error classes in JavaScript. It is similar to make-error in its simplicity and focus on creating custom error types.
Make your own error types!
instanceof
supporterror.name
& error.stack
supporteval()
)Installation of the npm package:
> npm install --save make-error
Then require the package:
var makeError = require("make-error");
You can directly use the build provided at unpkg.com:
<script src="https://unpkg.com/make-error@1/dist/make-error.js"></script>
var CustomError = makeError("CustomError");
// Parameters are forwarded to the super class (here Error).
throw new CustomError("a message");
function CustomError(customValue) {
CustomError.super.call(this, "custom error message");
this.customValue = customValue;
}
makeError(CustomError);
// Feel free to extend the prototype.
CustomError.prototype.myMethod = function CustomError$myMethod() {
console.log("CustomError.myMethod (%s, %s)", this.code, this.message);
};
//-----
try {
throw new CustomError(42);
} catch (error) {
error.myMethod();
}
var SpecializedError = makeError("SpecializedError", CustomError);
throw new SpecializedError(42);
Best for ES2015+.
import { BaseError } from "make-error";
class CustomError extends BaseError {
constructor() {
super("custom error message");
}
}
Contributions are very welcomed, either on the documentation or on the code.
You may:
ISC © Julien Fontanet
FAQs
Make your own error types!
The npm package make-error receives a total of 29,994,528 weekly downloads. As such, make-error popularity was classified as popular.
We found that make-error demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.