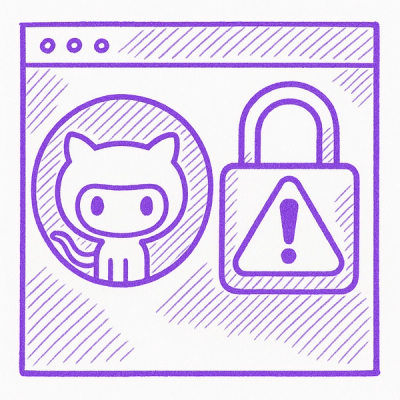
Research
/Security News
Toptal’s GitHub Organization Hijacked: 10 Malicious Packages Published
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
map-or-similar
Advanced tools
A JavaScript (JS) Map or Similar object polyfill if Map is not available.
The map-or-similar npm package provides a simple and flexible way to create and manage map-like collections. It allows for the easy manipulation of key-value pairs with a focus on providing a consistent interface that works similarly to the native JavaScript Map object, but with additional utilities and fallbacks for environments where Map is not available or desired.
Creating a new map
This feature allows users to create a new map-like collection. The collection can store key-value pairs, similar to the native JavaScript Map.
const MapOrSimilar = require('map-or-similar');
const myMap = new MapOrSimilar();
Setting and getting values
Users can set key-value pairs in the collection and retrieve values by their keys. This functionality mimics that of the JavaScript Map, providing a familiar interface for managing collections.
myMap.set('key', 'value');
const value = myMap.get('key');
Checking for key existence
This feature allows users to check whether a specific key exists in the collection. It's useful for conditionally adding or modifying data based on the presence of keys.
const hasKey = myMap.has('key');
Lodash is a comprehensive utility library that offers a wide range of functions for manipulating objects, arrays, and more. While it provides some similar functionalities to map-or-similar, such as managing collections and key-value pairs, lodash is much broader in scope and includes a vast array of utilities beyond just map-like collections.
Immutable.js offers persistent data structures including Map, List, Set, and more. Unlike map-or-similar, which provides a simple map-like interface, Immutable.js focuses on immutable collections. This ensures that the original collection cannot be modified, offering a different approach to collection management that can be beneficial for certain applications.
Returns a JavaScript Map() or a similar object with the same interface, if Map is not available. Focuses on performance. No dependencies. Made for the browser and nodejs.
npm install map-or-similar --save
var MapOrSimilar = require('map-or-similar');
// make a new map or similar object
var myMap = new MapOrSimilar();
// use it like a map
myMap.set('key1', 'value1');
myMap.set({ val: 'complex object as key' }, 'value2');
The following methods and properties are supported identically to Map():
set(key, val) : Sets a value to a key. Key can be a complex object, array, etc.
get(key) : Returns the value of a key.
has(key) : Returns true if the key exists, otherwise false.
delete(key) : Deletes a key and its value.
forEach(callback) : Invokes callback(val, key, object) once for each key-value pair in insertion order.
size : Returns the number of keys-value pairs.
Does not support other Map methods or properties.
npm run test
Released under an MIT license.
FAQs
A JavaScript (JS) Map or Similar object polyfill if Map is not available.
We found that map-or-similar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
Research
/Security News
Socket researchers investigate 4 malicious npm and PyPI packages with 56,000+ downloads that install surveillance malware.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.