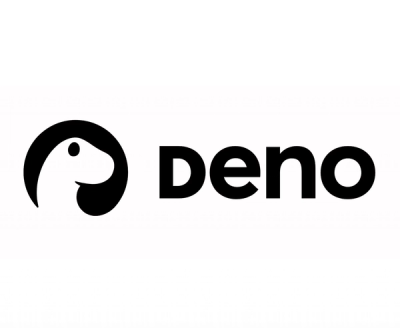
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Highlight keywords using JavaScript. Intended for every use case. Can e.g. be used to mark text in search results.
mark.js is a JavaScript library for highlighting text. It allows you to search for text within a web page and highlight it, making it useful for search functionality, text analysis, and more.
Basic Text Highlighting
This feature allows you to highlight specific text within a given context. In this example, the word 'highlight' will be highlighted within the element with the class 'context'.
const Mark = require('mark.js');
const context = document.querySelector('.context');
const instance = new Mark(context);
instance.mark('highlight');
Custom Highlighting Options
This feature allows you to customize the highlighting element and class. In this example, the word 'highlight' will be wrapped in a <span> element with the class 'custom-highlight'.
const Mark = require('mark.js');
const context = document.querySelector('.context');
const instance = new Mark(context);
instance.mark('highlight', {
'element': 'span',
'className': 'custom-highlight'
});
Unmarking Text
This feature allows you to remove all highlights within a given context. In this example, all highlights within the element with the class 'context' will be removed.
const Mark = require('mark.js');
const context = document.querySelector('.context');
const instance = new Mark(context);
instance.unmark();
highlight.js is a syntax highlighter written in JavaScript. It is used to highlight code syntax in web pages. Unlike mark.js, which is used for text highlighting, highlight.js is specifically designed for code syntax highlighting.
Prism is a lightweight, extensible syntax highlighter. It is used to highlight code syntax in web pages. Similar to highlight.js, Prism is focused on code syntax highlighting rather than general text highlighting like mark.js.
text-highlighter is a JavaScript library for highlighting text in web pages. It provides similar functionality to mark.js, allowing you to highlight text within a given context. However, it may not be as feature-rich or customizable as mark.js.
Please view the website for documentation and further information!
See the contribution guidelines.
Changes are documented in release descriptions.
You want to be notified about new releases? Click the "Subscribe to releases"
button on libraries.io.
Happy hacking!
FAQs
Highlight keywords using JavaScript. Intended for every use case. Can e.g. be used to mark text in search results.
The npm package mark.js receives a total of 981,407 weekly downloads. As such, mark.js popularity was classified as popular.
We found that mark.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.