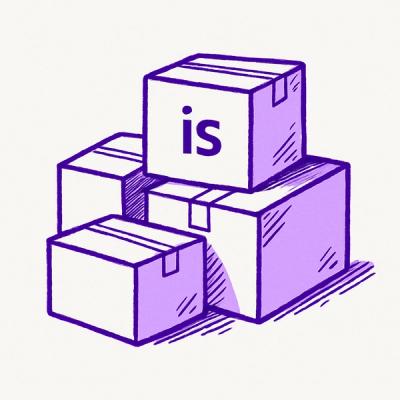
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
The 'matcher' npm package is a utility for matching strings against patterns. It is useful for filtering arrays of strings, validating input, and more. It supports wildcards and provides a simple API for common matching tasks.
Basic String Matching
This feature allows you to check if a string matches a given pattern using wildcards. In this example, 'hello' matches the pattern 'h*o'.
const matcher = require('matcher');
const result = matcher.isMatch('hello', 'h*o');
console.log(result); // true
Filtering Arrays
This feature allows you to filter an array of strings based on a pattern. In this example, the array ['foo', 'bar', 'baz'] is filtered to include only strings that start with 'b'.
const matcher = require('matcher');
const result = matcher(['foo', 'bar', 'baz'], 'b*');
console.log(result); // ['bar', 'baz']
Negated Patterns
This feature allows you to exclude strings that match a given pattern. In this example, the array ['foo', 'bar', 'baz'] is filtered to exclude strings that start with 'b'.
const matcher = require('matcher');
const result = matcher(['foo', 'bar', 'baz'], '!b*');
console.log(result); // ['foo']
Minimatch is a package for matching file paths against glob patterns. It is more focused on file system operations and supports a wide range of globbing features. Compared to 'matcher', 'minimatch' is more powerful for complex pattern matching but may be overkill for simple string matching tasks.
Micromatch is a fast and lightweight glob matcher for JavaScript. It provides extensive support for advanced globbing patterns and is optimized for performance. While 'micromatch' offers more features and flexibility, 'matcher' is simpler and easier to use for basic string matching.
Multimatch is a package that allows you to match multiple patterns against an array of strings. It is built on top of 'minimatch' and provides a convenient API for handling multiple patterns. 'Multimatch' is useful when you need to apply several patterns at once, whereas 'matcher' is more straightforward for single pattern matching.
Simple wildcard matching
Useful when you want to accept loose string input and regexes/globs are too convoluted.
npm install matcher
import {matcher, isMatch} from 'matcher';
matcher(['foo', 'bar', 'moo'], ['*oo', '!foo']);
//=> ['moo']
matcher(['foo', 'bar', 'moo'], ['!*oo']);
//=> ['bar']
matcher('moo', ['']);
//=> []
matcher('moo', []);
//=> []
matcher([''], ['']);
//=> ['']
isMatch('unicorn', 'uni*');
//=> true
isMatch('unicorn', '*corn');
//=> true
isMatch('unicorn', 'un*rn');
//=> true
isMatch('rainbow', '!unicorn');
//=> true
isMatch('foo bar baz', 'foo b* b*');
//=> true
isMatch('unicorn', 'uni\\*');
//=> false
isMatch(['foo', 'bar'], 'f*');
//=> true
isMatch(['foo', 'bar'], ['a*', 'b*']);
//=> true
isMatch('unicorn', ['']);
//=> false
isMatch('unicorn', []);
//=> false
isMatch([], 'bar');
//=> false
isMatch([], []);
//=> false
isMatch('', '');
//=> true
It matches even across newlines. For example, foo*r
will match foo\nbar
.
Accepts a string or an array of strings for both inputs
and patterns
.
Returns an array of inputs
filtered based on the patterns
.
Accepts a string or an array of strings for both inputs
and patterns
.
Returns a boolean
of whether any of given inputs
matches all the patterns
.
Type: string | string[]
The string or array of strings to match.
Type: object
Type: boolean
Default: false
Treat uppercase and lowercase characters as being the same.
Ensure you use this correctly. For example, files and directories should be matched case-insensitively, while most often, object keys should be matched case-sensitively.
import {isMatch} from 'matcher';
isMatch('UNICORN', 'UNI*', {caseSensitive: true});
//=> true
isMatch('UNICORN', 'unicorn', {caseSensitive: true});
//=> false
isMatch('unicorn', ['tri*', 'UNI*'], {caseSensitive: true});
//=> false
Type: boolean
Default: false
Require all negated patterns to not match and any normal patterns to match at least once. Otherwise, it will be a no-match condition.
import {matcher} from 'matcher';
// Find text strings containing both "edge" and "tiger" in arbitrary order, but not "stunt".
const demo = (strings) => matcher(strings, ['*edge*', '*tiger*', '!*stunt*'], {allPatterns: true});
demo(['Hey, tiger!', 'tiger has edge over hyenas', 'pushing a tiger over the edge is a stunt']);
//=> ['tiger has edge over hyenas']
import {matcher} from 'matcher';
matcher(['foo', 'for', 'bar'], ['f*', 'b*', '!x*'], {allPatterns: true});
//=> ['foo', 'for', 'bar']
matcher(['foo', 'for', 'bar'], ['f*'], {allPatterns: true});
//=> []
Type: string | string[]
Use *
to match zero or more characters.
A leading !
negates the pattern.
An input string will be omitted, if it does not match any non-negated patterns present, or if it matches a negated pattern, or if no pattern is present.
npm run bench
minimatch.match()
with support for multiple patternsFAQs
Simple wildcard matching
The npm package matcher receives a total of 3,193,558 weekly downloads. As such, matcher popularity was classified as popular.
We found that matcher demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.