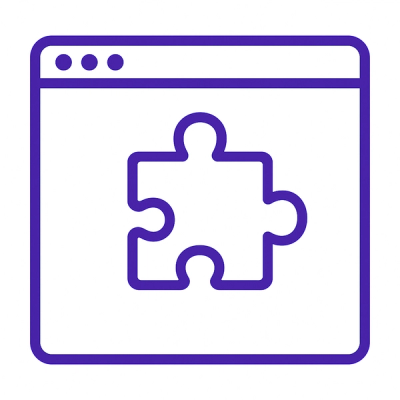
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
merge-stream
Advanced tools
The merge-stream npm package is used to create a stream that emits events from multiple other streams. This is useful when you want to handle multiple streams as if they were a single stream, for example, when you are dealing with multiple sources of data or tasks in a build process.
Merging multiple streams
This feature allows you to merge multiple streams into one. In the code sample, `stream1` and `stream2` are merged into `mergedStream`. You can then listen to events from `mergedStream` as if it was a single stream.
var stream1 = source1.pipe(transform1);
var stream2 = source2.pipe(transform2);
var mergedStream = mergeStream(stream1, stream2);
mergedStream.on('data', function(data) {
console.log(data);
});
Adding streams dynamically
This feature allows you to add streams to the merged stream dynamically. Initially, `mergedStream` is created without any sources. Streams `stream1` and `stream2` are then created and added to `mergedStream` dynamically.
var mergedStream = mergeStream();
var stream1 = source1.pipe(transform1);
mergedStream.add(stream1);
var stream2 = source2.pipe(transform2);
mergedStream.add(stream2);
Similar to merge-stream, multistream allows you to combine multiple streams into one. However, multistream works by taking an array of streams or a function that returns a stream, and reads them one after the other, not necessarily in parallel as merge-stream does.
Combined-stream is another package that is similar to merge-stream. It is used to create a stream that emits data from multiple streams one after the other. It differs from merge-stream in that it does not handle the streams in parallel but sequentially.
Pumpify combines streams into a single duplex stream. This is similar to merge-stream in that it deals with multiple streams, but pumpify is more about creating a pipeline of streams where the output of one stream is the input to the next, rather than merging their outputs.
Merge (interleave) a bunch of streams.
var stream1 = new Stream();
var stream2 = new Stream();
var merged = mergeStream(stream1, stream2);
var stream3 = new Stream();
merged.add(stream3);
merged.isEmpty();
//=> false
This is adapted from event-stream separated into a new module, using Streams3.
mergeStream
Type: function
Merges an arbitrary number of streams. Returns a merged stream.
merged.add
A method to dynamically add more sources to the stream. The argument supplied to add
can be either a source or an array of sources.
merged.isEmpty
A method that tells you if the merged stream is empty.
When a stream is "empty" (aka. no sources were added), it could not be returned to a gulp task.
So, we could do something like this:
stream = require('merge-stream')();
// Something like a loop to add some streams to the merge stream
// stream.add(streamA);
// stream.add(streamB);
return stream.isEmpty() ? null : stream;
An example use case for merge-stream is to combine parts of a task in a project's gulpfile.js like this:
const gulp = require('gulp');
const htmlValidator = require('gulp-w3c-html-validator');
const jsHint = require('gulp-jshint');
const mergeStream = require('merge-stream');
function lint() {
return mergeStream(
gulp.src('src/*.html')
.pipe(htmlValidator())
.pipe(htmlValidator.reporter()),
gulp.src('src/*.js')
.pipe(jsHint())
.pipe(jsHint.reporter())
);
}
gulp.task('lint', lint);
MIT
FAQs
Create a stream that emits events from multiple other streams
The npm package merge-stream receives a total of 45,290,840 weekly downloads. As such, merge-stream popularity was classified as popular.
We found that merge-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.