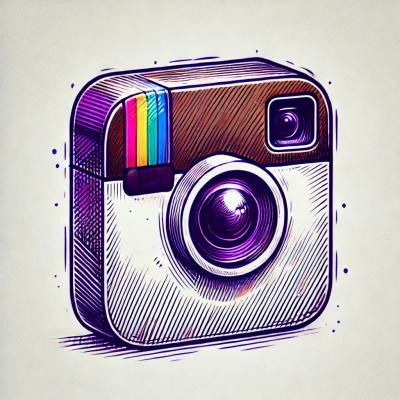
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
meta-cloud-api
Advanced tools
Meta Cloud API - A powerful TypeScript wrapper for Meta's Cloud API, providing a clean and type-safe interface for WhatsApp Business Platform integration.
npm install meta-cloud-api
# or
yarn add meta-cloud-api
# or
pnpm add meta-cloud-api
import WhatsApp, { MessageTypesEnum } from 'meta-cloud-api';
// Initialize the client
const client = new WhatsApp({
accessToken: process.env.CLOUD_API_ACCESS_TOKEN,
phoneNumberId: Number(process.env.WA_PHONE_NUMBER_ID),
businessAcctId: process.env.WA_BUSINESS_ACCOUNT_ID
});
// Send a WhatsApp text message
const response = await client.messages.text({
to: '+1234567890',
body: 'Hello from Meta Cloud API!'
});
console.log(`Message ID: ${response.messages[0].id}`);
Send different types of messages through WhatsApp Business Platform:
const result = await client.messages.text({
to: "15551234567",
body: "Hello from Meta Cloud API!"
});
import { ComponentTypesEnum, LanguagesEnum, ParametersTypesEnum } from 'meta-cloud-api';
const result = await client.messages.template({
to: "15551234567",
body: {
name: "shipping_confirmation",
language: {
code: LanguagesEnum.English_US,
policy: "deterministic"
},
components: [
{
type: ComponentTypesEnum.Body,
parameters: [
{
type: ParametersTypesEnum.Text,
text: "John Doe"
},
{
type: ParametersTypesEnum.Text,
text: "12345"
}
]
}
]
}
});
const result = await client.messages.image({
to: "15551234567",
body: {
link: "https://example.com/image.jpg"
}
});
import { InteractiveTypesEnum } from 'meta-cloud-api';
const result = await client.messages.interactive({
to: "15551234567",
body: {
type: InteractiveTypesEnum.Button,
body: {
text: "What would you like to do?"
},
action: {
buttons: [
{
type: "reply",
reply: {
id: "help_button",
title: "Get Help"
}
},
{
type: "reply",
reply: {
id: "info_button",
title: "Account Info"
}
}
]
}
}
});
We provide ready-to-use example projects demonstrating how to integrate Meta Cloud API in different frameworks:
Each example includes its own README with setup instructions and demonstration of core features.
Set up a webhook to receive messages and events from the WhatsApp API:
import WhatsApp, {
WebhookHandler,
MessageTypesEnum,
WebhookMessage
} from 'meta-cloud-api';
// Initialize the handler
const webhookHandler = new WebhookHandler({
accessToken: process.env.CLOUD_API_ACCESS_TOKEN,
phoneNumberId: Number(process.env.WA_PHONE_NUMBER_ID),
businessAcctId: process.env.WA_BUSINESS_ACCOUNT_ID,
webhookVerificationToken: process.env.WEBHOOK_VERIFICATION_TOKEN
});
// Handle webhook verification
app.get('/webhook', (req, res) => {
webhookHandler.handleVerificationRequest(req, res);
});
// Handle incoming messages
app.post('/webhook', (req, res) => {
webhookHandler.handleWebhookRequest(req, res);
});
// Pre-process all messages (e.g., mark as read)
webhookHandler.onMessagePreProcess(async (client: WhatsApp, message: WebhookMessage) => {
await client.messages.markAsRead({ messageId: message.id });
});
// Handle text messages
webhookHandler.onMessage(MessageTypesEnum.Text, async (client: WhatsApp, message: WebhookMessage) => {
if (message.text?.body) {
await client.messages.text({
body: `You said: "${message.text.body}"`,
to: message.from
});
}
});
// Handle image messages
webhookHandler.onMessage(MessageTypesEnum.Image, async (client: WhatsApp, message: WebhookMessage) => {
await client.messages.text({
body: "Thanks for the image!",
to: message.from
});
});
Manage message templates for your WhatsApp Business account:
// List all templates
const templates = await client.templates.list({
businessId: process.env.WA_BUSINESS_ACCOUNT_ID
});
// Create a new template
const newTemplate = await client.templates.create({
businessId: process.env.WA_BUSINESS_ACCOUNT_ID,
name: "my_special_template",
category: "MARKETING",
components: [
{
type: "HEADER",
format: "TEXT",
text: "Special Offer"
},
{
type: "BODY",
text: "Hi {{1}}, we have a special offer for you: {{2}}% off your next purchase!"
},
{
type: "FOOTER",
text: "Tap the button below to shop now"
}
],
language: "en_US"
});
Upload and retrieve media for your messages:
import fs from 'fs';
// Upload media
const mediaUpload = await client.media.upload({
file: fs.createReadStream("./path/to/image.jpg"),
type: "image/jpeg"
});
// Get media URL
const media = await client.media.get({
mediaId: mediaUpload.id
});
Update your WhatsApp Business profile information:
const profile = await client.businessProfile.update({
about: "We provide the best service!",
address: "123 Business St, City",
description: "Premium products and services",
email: "contact@business.com",
websites: ["https://www.business.com"]
});
We welcome contributions! Please see our Contributing Guide for details.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
TypeScript wrapper for Meta's Cloud API
The npm package meta-cloud-api receives a total of 336 weekly downloads. As such, meta-cloud-api popularity was classified as not popular.
We found that meta-cloud-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.