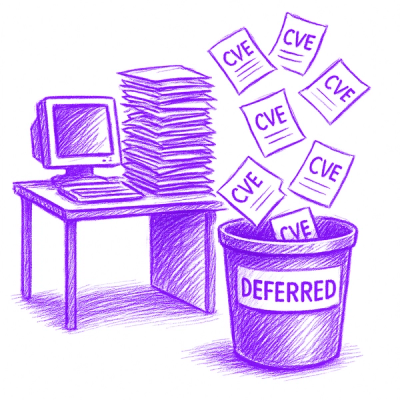
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
minimal-react-router
Advanced tools
minimal-react-router
is a lightweight router for React with a small API.
Uses React hooks and requires a peer dependency of react >=16.8.0
.
npm install minimal-react-router
import { createRouter } from "minimal-react-router";
const router = createRouter(window.history, location.href);
const routes = {
"/one": () => ComponentOne,
"/two": () => ComponentTwo
};
function App() {
// Routes are resolved async so the inital value is undefined.
// Set a default value for the placeholder.
const [Component = Spinner] = router.useRoutes(routes);
return <Component />;
}
router = createRouter(urlHistory, initialURL)
Creates a router instance.
@param urlHistory
History object. Implements pushState and replaceState methods.@param initialURL
Initial URL string.@returns router
[
result,
{
parameters: string[],
path: PathURL {}
}
] = router.useRoutes(routes)
A custom React hook that takes a routes
object and returns a result of the matching route.
@param routes
An object describing the routes.@returns [result, { parameters, path }]
router.push("/new/path")
Navigates to a new path and calls urlHistory.pushState
internally.
Returns a promise that resolves when all currently loaded routes are resolved.
router.replace("/replaced/path")
Replaces the current path and calls urlHistory.replaceState
internally.
Returns a promise that resolves when all currently loaded routes are resolved.
An object describing the routes.
const routes = {
"/one": () => ComponentOne,
"/two": () => ComponentTwo
};
"/foo/bar": () => Component
"/foo?param#hash": () => Component // Error!
"/foo": ({ path }) => {
// do something with path.searchParams or path.hash
}
"/foo/:param/:anotherParam": ({ parameters }) => {
// path: "/foo/bar/baz" = parameters: ["bar", "baz"]
}
"/foo/*/": () => FooSomething,
"/foo/*": () => FooEverythingElse
"/home": async () => await isAuthenticated() ? UserHome : Home
"/oldhome": ({ redirect }) => redirect("/home")
"/foo/:bar/:baz": ({ parameters, path }) => {
path.toString() // "/foo/some/thing?q=1#hash"
parameters // ["some", "thing"]
}
A path object similar to URL but only deals with paths, query parameters, and hashes.
Example:
{
hash: "#hash",
pathname: "/foo/bar",
searchParams: URLSearchParams {},
ensureFinalSlash: () => "/foo/bar/",
// Case insensitive, final slash insensitive, compares pathname, query params, and hash.
matches: (path: string | PathURL) => boolean,
toString: () => "/foo/bar?queryParam=foo#hash"
}
FAQs
A lightweight router for React.
The npm package minimal-react-router receives a total of 1 weekly downloads. As such, minimal-react-router popularity was classified as not popular.
We found that minimal-react-router demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.