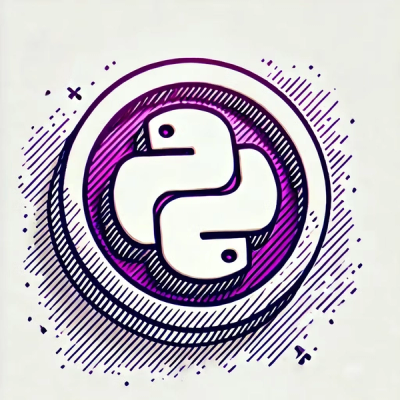
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
minimalistic-assert
Advanced tools
The minimalistic-assert npm package is a minimal assertion library that provides a simple way to assert truths in JavaScript code. It is designed to be small and straightforward, offering basic assertion functionality without any frills.
Assert truthiness
This feature allows you to assert that a value is truthy. If the value is not truthy, it throws an AssertionError with the provided message.
var assert = require('minimalistic-assert');
assert(true, 'This will not throw');
assert(false, 'This will throw an AssertionError');
Assert equality
This feature allows you to assert that two values are equal using the '==' operator. If they are not equal, it throws an AssertionError with the provided message.
var assert = require('minimalistic-assert');
assert.equal(1, 1, 'This will not throw');
assert.equal(1, 2, 'This will throw an AssertionError');
Assert strict equality
This feature allows you to assert that two values are strictly equal using the '===' operator. If they are not strictly equal, it throws an AssertionError with the provided message.
var assert = require('minimalistic-assert');
assert.strictEqual(1, 1, 'This will not throw');
assert.strictEqual(1, '1', 'This will throw an AssertionError');
The 'assert' module is a part of Node.js core and provides a simple set of assertion tests. It is more feature-rich than minimalistic-assert, offering a wider range of assertion methods such as 'deepEqual', 'notEqual', and 'throws'.
Chai is a BDD/TDD assertion library for node and the browser that can be paired with any JavaScript testing framework. It offers a more expressive and readable syntax compared to minimalistic-assert, with methods like 'expect', 'should', and 'assert' that support a range of assertions.
Expect.js is a minimalistic BDD-style assertions library that provides a similar set of features to minimalistic-assert but with a more fluent and chainable API. It allows for more readable tests and includes additional matchers.
very minimalistic assert module.
FAQs
minimalistic-assert ===
The npm package minimalistic-assert receives a total of 17,914,546 weekly downloads. As such, minimalistic-assert popularity was classified as popular.
We found that minimalistic-assert demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.