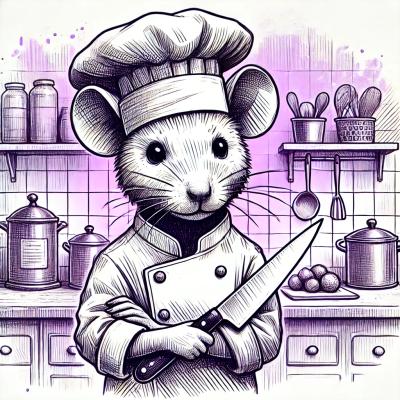
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
mobile-detect
Advanced tools
Device detection (phone, tablet, desktop, mobile grade, os, versions)
The 'mobile-detect' npm package is a lightweight JavaScript library used to detect mobile devices, operating systems, and user agents. It helps developers tailor their web applications to different devices by providing detailed information about the user's device.
Detect Mobile Device
This feature allows you to detect if the user is on a mobile device and identify the type of device. The code sample demonstrates how to use the 'mobile' method to get the device type.
const MobileDetect = require('mobile-detect');
const md = new MobileDetect(window.navigator.userAgent);
console.log(md.mobile()); // 'iPhone' for iPhone, 'Android' for Android devices, null for desktop
Detect Operating System
This feature allows you to detect the operating system of the user's device. The code sample shows how to use the 'os' method to get the operating system.
const MobileDetect = require('mobile-detect');
const md = new MobileDetect(window.navigator.userAgent);
console.log(md.os()); // 'iOS' for iPhone, 'AndroidOS' for Android devices, null for desktop
Detect User Agent
This feature allows you to detect the user agent of the browser. The code sample demonstrates how to use the 'userAgent' method to get the browser's user agent.
const MobileDetect = require('mobile-detect');
const md = new MobileDetect(window.navigator.userAgent);
console.log(md.userAgent()); // 'Safari' for Safari browser, 'Chrome' for Chrome browser, etc.
Detect Phone Model
This feature allows you to detect the specific phone model. The code sample shows how to use the 'phone' method to get the phone model.
const MobileDetect = require('mobile-detect');
const md = new MobileDetect(window.navigator.userAgent);
console.log(md.phone()); // 'iPhone' for iPhone, 'Nexus' for Nexus devices, etc.
The 'ua-parser-js' package is a JavaScript library for parsing user agent strings. It provides detailed information about the browser, engine, OS, CPU, and device. Compared to 'mobile-detect', 'ua-parser-js' offers more comprehensive parsing capabilities and supports a wider range of devices and browsers.
The 'detect-browser' package is a lightweight library for detecting the browser and its version. It is simpler and more focused on browser detection compared to 'mobile-detect', which provides broader device and OS detection capabilities.
The 'platform' package is a library for detecting the platform (operating system, browser, and engine) of the user's device. It provides detailed information similar to 'mobile-detect' but focuses more on the platform rather than the specific device type.
A loose port of Mobile-Detect to JavaScript.
This script will detect the device by comparing patterns against a given User-Agent string. You can find out information about the device rendering your web page:
Current master
branch is using detection logic from Mobile-Detect@2.8.37
Demo/check (sorry about the missing styling) can be found here.
TL;DR: you should not use this library in your HTML page and it's less reliable when used server-side (Node.js)
As mentioned later, "User-Agent" based detection is not a reliable solution in most cases, because:
If you still want to (or have to) use this library, you should always encapsulate it with your own code, because chances a very high that you have to tweak the behaviour a bit or are not satisfied with the result of mobile-detect.js. Don't spread usage of MobileDetect all over your own code! As you can see in the issues, there are some "bugs", feature-requests, pull-requests where people are not so happy how MobileDetect works. But I cannot change its behaviour from version to version, even if this was reasonable from new users' point of view. I hope you show understanding.
At least there is a way to monkey-patch the library (see "Extending" below).
<script src="mobile-detect.js"></script>
<script>
var md = new MobileDetect(window.navigator.userAgent);
// ... see below
</script>
var MobileDetect = require('mobile-detect'),
md = new MobileDetect(req.headers['user-agent']);
// ... see below
var md = new MobileDetect(
'Mozilla/5.0 (Linux; U; Android 4.0.3; en-in; SonyEricssonMT11i' +
' Build/4.1.A.0.562) AppleWebKit/534.30 (KHTML, like Gecko)' +
' Version/4.0 Mobile Safari/534.30');
// more typically we would instantiate with 'window.navigator.userAgent'
// as user-agent; this string literal is only for better understanding
console.log( md.mobile() ); // 'Sony'
console.log( md.phone() ); // 'Sony'
console.log( md.tablet() ); // null
console.log( md.userAgent() ); // 'Safari'
console.log( md.os() ); // 'AndroidOS'
console.log( md.is('iPhone') ); // false
console.log( md.is('bot') ); // false
console.log( md.version('Webkit') ); // 534.3
console.log( md.versionStr('Build') ); // '4.1.A.0.562'
console.log( md.match('playstation|xbox') ); // false
There is some documentation generated by JSDoc:
https://hgoebl.github.io/mobile-detect.js/doc/MobileDetect.html
Script creates the global property MobileDetect
.
When using Modernizr, you can include mobile-detect-modernizr.js
.
It will add the CSS classes mobile
, phone
, tablet
and mobilegradea
if applicable.
You can easily extend it, e.g. android
, iphone
, etc.
cat mobile-detect.min.js | gzip -9f | wc -c
)$ bower install hgoebl/mobile-detect.js --save
$ npm install mobile-detect --save
<script src="https://cdn.jsdelivr.net/npm/mobile-detect@1.4.5/mobile-detect.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/mobile-detect/1.4.5/mobile-detect.min.js"></script>
Though it is not recommended to rely on internal methods or structures of MobileDetect, you can alter the behaviour by replacing particular internal methods with your own implementations. If you feel like this is the only possibility, then go ahead and have a look at the source code and examples in tests/spec/MobileDetectSpec.js (search for "Extensibility").
Often device detection is the first solution in your mind. Please consider looking for other solutions like media queries and feature detection (e.g. w/ Modernizr). Maybe there are better (simpler, smaller, faster) device detection libraries, so here you have a list (order has no meaning apart from first element):
If you have to provide statistics about how many and which mobile devices are hitting your web-site, you can generate statistics (data and views) with hgoebl/mobile-usage. There are many hooks to customize statistical calculation to your needs.
MIT-License (see LICENSE file).
Your contribution is welcome. If you want new devices to be supported, please contribute to Mobile-Detect instead.
To run generate-script it is necessary to have Mobile-Detect
as a sibling directory to mobile-detect.js/.
(I tried to use git subtree
but had some problems on Mac OS X - probably my fault...)
npm install
grunt
(needs PHP >= 5.4 in your path)Open tests/SpecRunner.html
in different browsers.
$ npm test
$ # or
$ grunt jasmine_node
If you want, you can donate to Mobile-Detect.
FAQs
Device detection (phone, tablet, desktop, mobile grade, os, versions)
The npm package mobile-detect receives a total of 171,120 weekly downloads. As such, mobile-detect popularity was classified as popular.
We found that mobile-detect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.