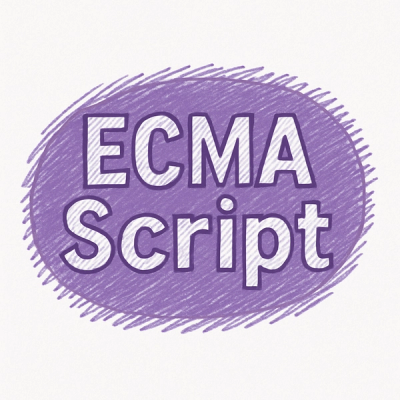
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
mock-aws-s3
Advanced tools
This is a very simple interface that mocks the AWS SDK for Node.js. The implementation is incomplete but most basic features are supported.
Available:
It uses a directory to mock a bucket and its content.
If you'd like to see some more features or you have some suggestions, feel free to use the issues or submit a pull request.
var AWSMock = require('mock-aws-s3');
AWSMock.config.basePath = '/tmp/buckets/' // Can configure a basePath for your local buckets
var s3 = AWSMock.S3({
params: { Bucket: 'example' }
});
s3.putObject({Key: 'sea/animal.json', Body: '{"is dog":false,"name":"otter","stringified object?":true}'}, function(err, data) {
s3.listObjects({Prefix: 'sea'}, function (err, data) {
console.log(data);
});
});
var params = { Bucket: 'example' };
s3.createBucket(params, function(err) {
if(err) {
console.error(err);
}
});
var params = { Bucket: 'example' };
s3.deleteBucket(params, function(err) {
if(err) {
console.error(err);
}
});
FAQs
Mock AWS S3 SDK for Node.js
The npm package mock-aws-s3 receives a total of 98,156 weekly downloads. As such, mock-aws-s3 popularity was classified as popular.
We found that mock-aws-s3 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.