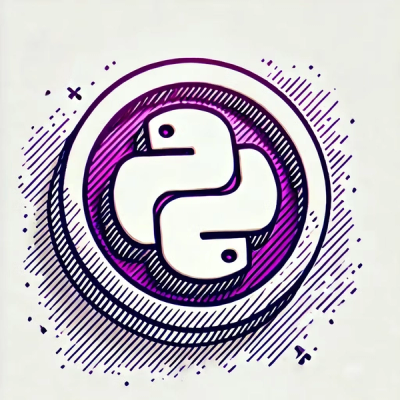
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Static typed facade for [IndexedDB](https://developer.mozilla.org/en-US/docs/Web/API/IndexedDB_API) with simple API.
Static typed facade for IndexedDB with simple API.
type User = {
name: string
admin: boolean
}
// Static typed schema
type DB = {
User: {
Key: [ string ]
Doc: User
Indexes: {
Name: [ string ]
}
}
}
// Automatic migrations
const db = await $mol_db< DB >( '$my_app',
mig => mig.store_make( 'User' ),
mig => {
const { User } = mig.stores
User.index_make( 'Name', [ 'name' ] )
},
)
// Writing transaction
const { User } = db.change( 'User' ).stores
await User.put({ name: 'Jin', admin: true })
// Reading transaction
const { Name } = db.read( 'User' ).User.indexes
const jins = await Name.select([ 'Jin' ])
type ACME = {
Users: {
Key: number
Doc: {
name: {
first: string
last: string
}
age: number
}
Indexes: {
names: [ string, string ]
ages: [ number ]
}
}
Articles: {
Key: string
Doc: {
title: string
content: string
}
Indexes: {
full: [ string, string ]
}
}
}
const db = await $$.$mol_db< ACME >( 'ACME' )
const db = await $$.$mol_db< ACME >( 'ACME',
mig => mig.store_make( 'Users' ),
mig => mig.stores.Users.index_make( 'ages', [ 'age' ] ),
mig => mig.stores.Users.index_make( 'names', [ 'name.first', 'name.last' ], true ),
mig => mig.store_make( 'Articles' ),
mig => mig.stores.Articles.index_make( 'full', [ 'title', 'content' ] ),
// mig => mig.stores.Articles.index_drop( 'full' ),
// mig => mig.store_drop( 'Articles' ),
)
There is 5 migrations. And DB version is 6. After uncommenting last 2 rows, it applies 2 additional migrations and DB version will be 8.
db.destructor()
db.kill()
const { Users, Articles } = db.read( 'Users', 'Articles' )
const trans = db.change( 'Users', 'Articles' )
const { Users, Articles } = trans.stores
// ...
trans.abort()
// or
await trans.commit()
Uncommitted transaction without errors will be committed automatically. Any modification error aborts transaction.
const key = await Users.put({
first: 'Jin',
last: 'Nin',
age: 36,
})
await Users.put( {
first: 'Jin',
last: 'Nin',
age: 37,
}, 1 )
const user = await Users.get( 1 )
const users = await Users.get( $mol_dom_context.IDBKeyRange.bound( 10, 50 ), 10 )
const count = await Users.count( $mol_dom_context.IDBKeyRange.bound( 10, 50 ) )
const { names, ages } = users.indexes
const user = await names.get([ 'Jin', 'Nin' ])
const users = await ages.get( [ 18 ], 10 )
const count = await Users.count([ 18 ])
npm install mol_db
import { $mol_db } from 'mol_db'
FAQs
Static typed facade for [IndexedDB](https://developer.mozilla.org/en-US/docs/Web/API/IndexedDB_API) with simple API.
The npm package mol_db receives a total of 487 weekly downloads. As such, mol_db popularity was classified as not popular.
We found that mol_db demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.