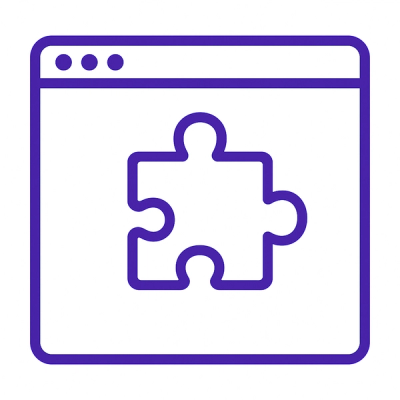
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
mongo-connection-string
Advanced tools
Utilities to help with MongoDB connection strings and related tasks.
Parse a connection string into ConnectionString
object:
const { ConnectionString } = require('mongo-connection-string');
const connectionString = new ConnectionString('mongodb://user:p@ssw0rd@host1,host2:2700/db?w=majority');
The connection string object has the following fields:
{
protocol: 'mongodb://',
username: 'user',
password: 'p@ssw0rd',
hosts: [{ host: 'host1', port: null }, { host: 'host2', port: 2700 }],
database: 'db',
options: {
w: 'majority'
}
}
You can now write it as a MongoDB compatible URI, or a more human readable string:
connectionString.toURI();
// Produces:
// mongodb://user:p%40ssw0rd/db?readPreference=secondary
connectionString.toString();
// Produces:
// mongodb://user:********/db?readPreference=secondary
// (a little bit safer to go into logs/error messages etc)!
The ConnectionString
constructor can also take the fields required to build a
connection string:
const { ConnectionString } = require('mongo-connection-string');
const connectionString = ConnectionString({
username: 'user',
password: 'pwd',
hosts: [{ host: 'host1' }],
database: 'db',
options: {
readPreference: 'secondary'
}
});
// Write out the connection string.
console.log(connectionString.toURI());
Produces:
mongodb://user:pwd@host1/db?readPreference=secondary
When parsing a connection string, encoded charecters are unencoded:
const { parse } = require('mongo-connection-string');
const connectionString = new ConnectionString('mongodb://%40dmin:P%40ssword%3C%3E%2F@host1,host2:2700/db?w=majority');
// Write out the connection string.
console.log(connectionString.username);
console.log(connectionString.password);
Produces:
@dmin
P@ssword<>/
Similarly, connection string object usernames and passwords are encoded:
const { ConnectionString } = require('mongo-connection-string');
const connectionString = ConnectionString({
username: '@dmin',
password: 'P@ssword<>/',
hosts: [{ host: 'localhost' }]
});
// Write out the connection string.
console.log(connectionString.toURI());
Produces:
mongodb://%40dmin:P%40ssword%3C%3E%2F@localhost
This means you can actually use the library to clean up a non-url encoded connection string. This can be useful to allow connection strings to be input in a more readable way (mongo-monitor does this):
new ConnectionString('@dmin:P@ssword<>/@localhost').toURI();
Produces:
mongodb://%40dmin:P%40ssword%3C%3E%2F@localhost
mongodb://
should be used.%
symbol in the username or password, the code will try to URI decode it. If this fails, it will assume the username or password is plain text with a %
symbol as part of the password. This means that if your password is actually something like p%40ssword
then this will be URI decoded to p@ssword
.0.1.5 (2020-02-17)
<a name="0.1.4"></a>
FAQs
Handle MongoDB connection strings with ease!
The npm package mongo-connection-string receives a total of 180 weekly downloads. As such, mongo-connection-string popularity was classified as not popular.
We found that mongo-connection-string demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.