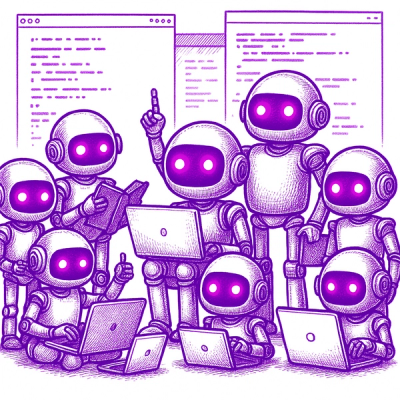
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600Ć Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
mongo-query-utils
Advanced tools
A simple mongo query builder for node.js for Node/Express.js.
ā
Create Documents ā Create a new document and save to database
ā
Fetch Documents ā Collect all or necessary documents from the database
ā
Update Documents ā Update all or specific document(s) from the database
ā
Delete Documents ā Remove documents from the database
ā
Join Documents ā Join documents from different tables
npm install mongo-query-utils
const {
fetchAll,
fetchOne,
generate,
fetchById,
patchOneAndUpdate,
patchByIdAndUpdate,
patchOne,
patchMany,
replaceOne,
removeOne,
removeMany,
summarize,
} = require("mongo-query-utils");
const Table = require("your-schema");
const document = {
first_name: "Sample",
last_name: "Test",
};
const newDoc = await generate(document, Table);
console.log(Table);
Fetch a single document based on the provided query.
const query = { email: "user@example.com" };
const user = await fetchOne(query, User);
console.log(user); // { _id: 123, email: "user@example.com", name: "John Doe" }
Retrieve a document using its unique _id.
const id = "60d5f9e8f1c2b4a3d8f5e7c6";
const document = await fetchById(id, Table);
console.log(document);
Update the first document that matches the query.
const query = { email: "user@example.com" };
const update = { name: "Updated Name" };
const updatedDoc = await patchOneAndUpdate(query, update, User);
console.log(updatedDoc);
const id = "60d5f9e8f1c2b4a3d8f5e7c6";
const update = { status: "Active" };
const updatedDoc = await patchByIdAndUpdate(id, update, Table);
console.log(updatedDoc);
const query = { status: "Pending" };
const update = { status: "Completed" };
const updatedDocs = await patchMany(query, update, Table);
console.log(updatedDocs);
Replace an entire document with new data.
const query = { email: "user@example.com" };
const newDocument = { email: "user@example.com", name: "New Name" };
const replacedDoc = await replaceOne(query, newDocument, User);
console.log(replacedDoc);
const query = { email: "user@example.com" };
const deletedDoc = await removeOne(query, User);
console.log(deletedDoc);
const query = { status: "Inactive" };
const deletedDocs = await removeMany(query, User);
console.log(deletedDocs);
Join documents from two collections using $lookup.
const pipeline = [
{
$match: {_id: 'sample_id'}
$lookup: {
from: "orders", // Collection to join
localField: "_id", // Field in primary collection
foreignField: "userId", // Field in foreign collection
as: "userOrders" // Output array
}
}
];
const usersWithOrders = await summarize(pipeline, User);
console.log(usersWithOrders);
Each function accepts an optional third parameter for customization:
const options = { sort: { createdAt: -1 }, limit: 10 };
const latestUsers = await fetchAll({}, User, options);
console.log(latestUsers);
MIT License. See LICENSE
for details.
š Author: Ryan Charles Alcaraz (github)
FAQs
A simple mongo query builder for node.js
We found that mongo-query-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.