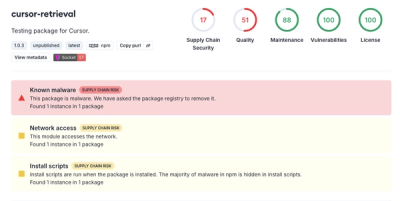
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
mongodb-uri
Advanced tools
The mongodb-uri npm package is a utility for parsing and formatting MongoDB URIs. It helps in converting MongoDB connection strings into a more manageable object format and vice versa.
Parsing MongoDB URIs
This feature allows you to parse a MongoDB URI string into an object that contains all the components of the URI, such as username, password, host, port, and database.
const mongodbUri = require('mongodb-uri');
const uri = 'mongodb://username:password@host:port/database';
const parsedUri = mongodbUri.parse(uri);
console.log(parsedUri);
Formatting MongoDB URIs
This feature allows you to format an object containing MongoDB URI components back into a URI string.
const mongodbUri = require('mongodb-uri');
const uriObject = {
scheme: 'mongodb',
username: 'username',
password: 'password',
hosts: [
{ host: 'host', port: 27017 }
],
database: 'database'
};
const formattedUri = mongodbUri.format(uriObject);
console.log(formattedUri);
Handling Multiple Hosts
This feature allows you to parse MongoDB URIs that contain multiple hosts, which is useful for connecting to replica sets.
const mongodbUri = require('mongodb-uri');
const uri = 'mongodb://username:password@host1:27017,host2:27018/database';
const parsedUri = mongodbUri.parse(uri);
console.log(parsedUri);
The official MongoDB driver for Node.js. While it provides comprehensive functionality for interacting with MongoDB databases, it also includes methods for parsing and formatting MongoDB URIs. However, it is more heavyweight compared to mongodb-uri, which is a lightweight utility focused solely on URI parsing and formatting.
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It provides a higher-level abstraction for working with MongoDB, including schema definitions and validation. Mongoose also includes URI parsing capabilities, but its primary focus is on data modeling and validation rather than just URI manipulation.
Parse and format MongoDB URIs of the form:
mongodb://[username[:password]@]host1[:port1][,host2[:port2],...[,hostN[:portN]]][/[database]][?options]
Note that there are two minor differences between this format and the standard MongoDB connect string URI format:
password
is optional even when a username
is supplieddatabase
is not required when leaving out the database
but specifying options
Neither of these differences should prevent this library from parsing any URI conforming to the standard format.
Takes a URI string and returns a URI object of the form:
{
scheme: !String,
username: String=,
password: String=,
hosts: [ { host: !String, port: Number= }, ... ],
database: String=,
options: Object=
}
scheme
and hosts
will always be present. Other fields will only be present in the result if they were present in the
input.
var mongodbUri = require('mongodb-uri');
var uri = 'mongodb://user%3An%40me:p%40ssword@host:1234/d%40tabase?authSource=%40dmin';
var uriObject = mongodbUri.parse(uri);
console.log(JSON.stringify(uriObject, null, 2));
{
"scheme": "mongodb",
"hosts": [
{
"host": "host",
"port": 1234
}
],
"username": "user:n@me",
"password": "p@ssword",
"options": {
"authSource": "@dmin"
},
"database": "d@tabase"
}
Takes a URI object and returns a URI string.
var mongodbUri = require('mongodb-uri');
var uri = mongodbUri.format(
{
username: 'user:n@me',
password: 'p@ssword',
hosts: [
{
host: 'host',
port: 1234
}
],
database: 'd@tabase',
options: {
authSource: '@dmin'
}
}
);
console.log(uri);
mongodb://user%3An%40me:p%40ssword@host:1234/d%40tabase?authSource=%40dmin
Takes either a URI object or string and returns a Mongoose connection string. Specifically, instead of listing all hosts and ports in a single URI, a Mongoose connection string contains a list of URIs each with a single host and port pair.
Useful in environments where a MongoDB URI environment variable is provided, but needs to be programmatically transformed into a string digestible by mongoose.connect()--for example, when deploying to a PaaS like Heroku using a MongoDB add-on like MongoLab.
var mongoose = require('mongoose');
var mongodbUri = require('mongodb-uri');
// A MongoDB URI, not compatible with Mongoose because it lists multiple hosts in the address
// Could be pulled from an environment variable or config file
var uri = 'mongodb://username:password@host1:1234,host2:5678/database';
// Reformat to a Mongoose connect string and connect()
var mongooseConnectString = mongodbUri.formatMongoose(uri);
mongoose.connect(mongooseConnectString);
// Test for connection success
var db = mongoose.connection;
db.on('error', console.error.bind(console, 'Connection error: '));
db.once('open', function callback () {
console.log('Successfully connected to MongoDB');
});
FAQs
A parser and formatter for MongoDB URIs.
The npm package mongodb-uri receives a total of 101,838 weekly downloads. As such, mongodb-uri popularity was classified as popular.
We found that mongodb-uri demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.