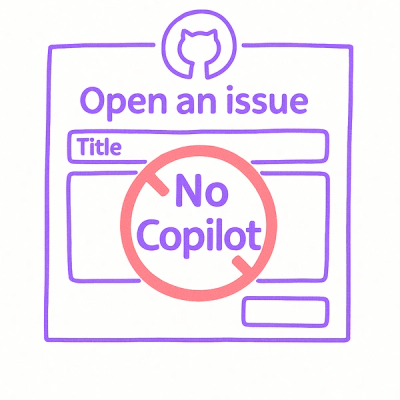
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Warning: This version is not API compatible with 0.1.
I have had some issues with my npm auth and got it in a bit of a rut, so for now you have to:
npm install mu2
Currently mu does not support changing the tag form ({{ }} to say <% %>).
There are a few ways to use mu 0.5. Here is the simplest:
var mu = require('mu2'); // notice the "2" which matches the npm repo, sorry..
mu.root = __dirname + '/templates'
mu.compileAndRender('index.html', {name: "john"})
.on('data', function (data) {
console.log(data.toString());
});
Here is an example mixing it with the http module:
var http = require('http')
, util = require('util')
, mu = require('mu2');
mu.root = __dirname + '/templates';
http.createServer(function (req, res) {
var stream = mu.compileAndRender('index.html', {name: "john"});
stream.pipe(res);
}).listen(8000);
Taking that last example here is a little trick to always compile the templates in development mode (so the changes are immediately reflected).
var http = require('http')
, util = require('util')
, mu = require('mu2');
mu.root = __dirname + '/templates';
http.createServer(function (req, res) {
if (process.env.NODE_ENV == 'DEVELOPMENT') {
mu.clearCache();
}
var stream = mu.compileAndRender('index.html', {name: "john"});
util.pump(stream, res);
}).listen(8000);
mu.root
A path to lookup templates from. Defaults to the working directory.
mu.compileAndRender(String templateName, Object view)
Returns: Stream
The first time this function is called with a specific template name, the
template will be compiled and then rendered to the stream. Subsequent
calls with the same template name will use a cached version of the compiled
template to improve performance (a lot).
mu.compile(filename, callback)
Returns nil
Callback (Error err, Any CompiledTemplate)
This function is used to compile a template. Usually you will not use it
directly but when doing wierd things, this might work for you. Does not
use the internal cache when called multiple times, though it does add the
compiled form to the cache.
mu.compileText(String name, String template, Function callback)
Returns nil
Callback (err, CompiledTemplate)
Similar to mu.compile except it taks in a name and the actual string of the
template. Does not do disk io. Does not auto-compile partials either.
mu.render(Mixed filenameOrCompiledTemplate, Object view)
Returns Stream
The brother of mu.compile. This function takes either a name of a template
previously compiled (in the cache) or the result of the mu.compile step.
This function is responsible for transforming the compiled template into the
proper output give the input view data.
mu.renderText(String template, Object view, Object partials)
Returns Stream
Like render, except takes a template as a string and an object for the partials.
This is not a very performant way to use mu, so only use this for dev/testing.
mu.clearCache(String templateNameOrNull)
Clears the cache for a specific template. If the name is omitted, clears all cache.
FAQs
A Mustache template engine for Node.js
The npm package mu2 receives a total of 73,064 weekly downloads. As such, mu2 popularity was classified as popular.
We found that mu2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.