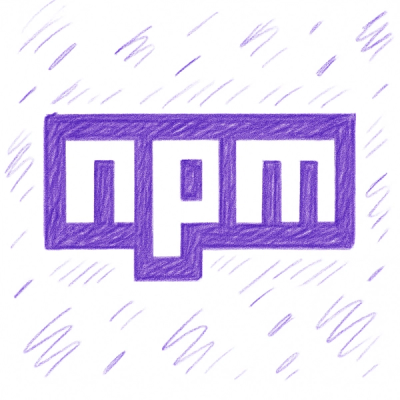
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
mygrsdatepicker
Advanced tools
Angular 2 date picker - Angular2 reusable UI component
Simple Angular2 date picker. Online demo is here
To install this component to an external project, follow the procedure:
npm install mygrsdatepicker --save
Add mygrsdatepickerModule import to your @NgModule like example below
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { MyTestApp } from './my-test-app';
import { mygrsdatepickerModule } from 'mygrsdatepicker';
@NgModule({
imports: [ BrowserModule, mygrsdatepickerModule ],
declarations: [ MyTestApp ],
bootstrap: [ MyTestApp ]
})
export class MyTestAppModule {}
Use the following snippet inside your template:
<my-date-picker [options]="mygrsdatepickerOptions"
(dateChanged)="onDateChanged($event)"></my-date-picker>
Mandatory attributes:
Optional attributes:
Example of the options data (not all properties listed):
mygrsdatepickerOptions = {
todayBtnTxt: 'Today',
dateFormat: 'yyyy-mm-dd',
firstDayOfWeek: 'mo',
sunHighlight: true,
height: '34px',
width: '260px',
inline: false,
disableUntil: {year: 2016, month: 8, day: 10},
selectionTxtFontSize: '16px'
};
onDateChanged(event:any) {
console.log('onDateChanged(): ', event.date, ' - formatted: ', event.formatted, ' - epoc timestamp: ', event.epoc);
}
If you are using systemjs package loader add the following mygrsdatepicker properties to the System.config:
(function (global) {
System.config({
paths: {
'npm:': 'node_modules/'
},
map: {
// Other components are here...
'mygrsdatepicker': 'npm:mygrsdatepicker',
},
packages: {
// Other components are here...
mygrsdatepicker: {
main: './index.js',
defaultExtension: 'js'
}
}
});
})(this);
Value of the options attribute is a javascript object. It can contain the following properties.
Option | Default | Description |
---|---|---|
dayLabels | {su: 'Sun', mo: 'Mon', tu: 'Tue', we: 'Wed', th: 'Thu', fr: 'Fri', sa: 'Sat'} | Day labels visible on the selector. |
monthLabels | { 1: 'Jan', 2: 'Feb', 3: 'Mar', 4: 'Apr', 5: 'May', 6: 'Jun', 7: 'Jul', 8: 'Aug', 9: 'Sep', 10: 'Oct', 11: 'Nov', 12: 'Dec' } | Month labels visible on the selector. |
dateFormat | yyyy-mm-dd | Date format on selection area and callback. |
todayBtnTxt | Today | Today button text. |
firstDayOfWeek | mo | First day of week on calendar. One of the following: mo, tu, we, th, fr, sa, su |
sunHighlight | true | Sunday red colored on calendar. |
editableMonthAndYear | true | Is month and year labels editable or not. |
minYear | 1000 | Minimum allowed year in calendar. Cannot be less than 1000. |
maxYear | 9999 | Maximum allowed year in calendar. Cannot be more than 9999. |
disableUntil | no default value | Disable dates backward starting from the given date. For example: {year: 2016, month: 6, day: 26} |
disableSince | no default value | Disable dates forward starting from the given date. For example: {year: 2016, month: 7, day: 22} |
disableWeekends | false | Disable weekends (Saturday and Sunday). |
inline | false | Show mygrsdatepicker in inline mode. |
height | 34px | mygrsdatepicker height without selector. Can be used if inline = false. |
width | 100% | mygrsdatepicker width. Can be used if inline = false. |
selectionTxtFontSize | 18px | Selection area font size. Can be used if inline = false. |
alignSelectorRight | false | Align selector right. Can be used if inline = false. |
indicateInvalidDate | true | If user typed date is not same format as dateFormat, show red background in the selection area. Can be used if inline = false. |
showDateFormatPlaceholder | false | Show value of dateFormat as placeholder in the selection area if it is empty. Can be used if inline = false. |
A two-letter ISO 639-1 language code can be provided as shorthand for several of the options listed above. Currently supported languages: en, fr, ja, fi and es. If the locale attribute is used it overrides dayLabels, monthLabels, dateFormat, todayBtnTxt, firstDayOfWeek and sunHighlight properties from the options.
Provide the initially chosen date that will display both in the text input field and provide the default for the popped-up selector.
If selDate is not specified, when the datepicker is opened, it will ordinarily default to selecting the current date. If you would prefer a different year and month to be the default for a freshly chosen date picking operation, specify a [defaultMonth] in the same format as that for the datepicker options (yyyy.mm if not otherwise specified).
At first fork and clone this repo.
Install all dependencies:
Run sample application:
Build dist folder (javascript version of the component):
Execute unit tests and coverage (output is generated to the test-output folder):
Online demo is here
FAQs
Angular2 date picker
The npm package mygrsdatepicker receives a total of 0 weekly downloads. As such, mygrsdatepicker popularity was classified as not popular.
We found that mygrsdatepicker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.