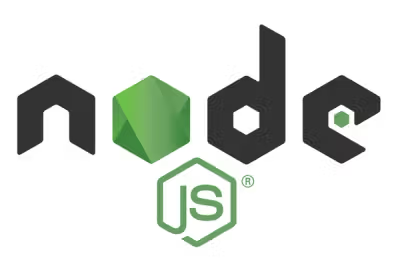
Security News
Node.js TSC Declines to Endorse Feature Bounty Program
The Node.js TSC opted not to endorse a feature bounty program, citing concerns about incentives, governance, and project neutrality.
nano-promise
Advanced tools
A small Promise/A++ node module.
var Promise = require('nano-promise');
new Promise(function (resolve, reject) {
resolve(1, 2, 3, 4); // you can pass several arguments
}).then(function (a, b, c, d) {
console.log(a, b, c, d); // 1 2 3 4
return new Promise.Arguments(5, 6, 7, 8); // and return
}).then(function (a, b, c, d) {
console.log(a, b, c, d); // 5 6 7 8
});
Promise.resolve(5, 'a')
.then(function (a, b) {
console.log(a, b);
});
Like Promise.all() but ...
function timer(ms, value) {
return new Promise(function (resolve, reject) {
var to = setTimeout(function () {
resolve(value);
}, ms);
return { cancel: function () {
clearTimeout(to); // can be called in pending state one time only
}};
});
}
Promise.resolve(timer(1000, 'go'), timer(800, 'up'))
.then(function (a, b) {
console.log(a, b); // 'go', 'up'
});
Promies.concat([
new Promise(function (resolve, reject) {
resolve(1, 2, 3, 4);
},
new Promise(function (resolve, reject) {
resolve(4, 5, 6, 7);
}])
.then(function (a,b,c,d,e,f,g,h) {
console.log(a,b,c,d,e,f,g,h); // 1 2 3 4 5 6 7 8
});
function timer(ms) {
return new Promise(function (resolve, reject) {
var to = setTimeout(resolve, ms);
return { cancel: function () {
clearTimeout(to); // can be called in pending state one time only
}};
});
}
timer(1000).then(function () {
console.log('timeout');
}, function (r) {
if (r === Promise.CANCEL_REASON)
console.log('cancelled');
}).cancel();
FAQs
A small Promise/A++ node module
The npm package nano-promise receives a total of 861 weekly downloads. As such, nano-promise popularity was classified as not popular.
We found that nano-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The Node.js TSC opted not to endorse a feature bounty program, citing concerns about incentives, governance, and project neutrality.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.