nativescript-slides
Advanced tools
Comparing version 2.2.14 to 2.3.0
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
require("nativescript-dom"); | ||
require('nativescript-dom'); | ||
var app = require("application"); | ||
@@ -23,3 +23,3 @@ var Platform = require("platform"); | ||
} | ||
var Slide = (function (_super) { | ||
var Slide = /** @class */ (function (_super) { | ||
__extends(Slide, _super); | ||
@@ -44,3 +44,3 @@ function Slide() { | ||
})(cancellationReason || (cancellationReason = {})); | ||
var SlideContainer = (function (_super) { | ||
var SlideContainer = /** @class */ (function (_super) { | ||
__extends(SlideContainer, _super); | ||
@@ -64,3 +64,3 @@ function SlideContainer() { | ||
if (typeof value === 'string') { | ||
value = (value == 'true'); | ||
value = value == 'true'; | ||
} | ||
@@ -111,3 +111,14 @@ this._pageIndicators = value; | ||
set: function (value) { | ||
if (this._disablePan === value) { | ||
return; | ||
} // Value did not change | ||
this._disablePan = value; | ||
if (this._loaded && this.currentPanel.panel !== undefined) { | ||
if (value === true) { | ||
this.currentPanel.panel.off('pan'); | ||
} | ||
else if (value === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
} | ||
}, | ||
@@ -188,3 +199,3 @@ enumerable: true, | ||
} | ||
_this.width = +(_this.slideWidth); | ||
_this.width = +_this.slideWidth; | ||
_this.eachLayoutChild(function (view) { | ||
@@ -294,3 +305,5 @@ if (view instanceof stack_layout_1.StackLayout) { | ||
SlideContainer.prototype.goToSlide = function (index) { | ||
if (this._slideMap && this._slideMap.length > 0 && index < this._slideMap.length) { | ||
if (this._slideMap && | ||
this._slideMap.length > 0 && | ||
index < this._slideMap.length) { | ||
var previousSlide = this.currentPanel; | ||
@@ -304,3 +317,3 @@ this.setupPanel(this._slideMap[index]); | ||
newIndex: this.currentPanel.index, | ||
oldIndex: previousSlide.index, | ||
oldIndex: previousSlide.index | ||
} | ||
@@ -329,3 +342,3 @@ }); | ||
// swiping left to right. | ||
if (args.deltaX > (pageWidth / 3)) { | ||
if (args.deltaX > pageWidth / 3) { | ||
if (_this.hasPrevious) { | ||
@@ -345,3 +358,4 @@ _this.transitioning = true; | ||
} | ||
else if (args.deltaX < (-pageWidth / 3)) { | ||
// swiping right to left | ||
else if (args.deltaX < -pageWidth / 3) { | ||
if (_this.hasNext) { | ||
@@ -385,2 +399,3 @@ _this.transitioning = true; | ||
if (app.ios) | ||
//for some reason i have to set these in ios or there is some sort of bounce back. | ||
_this.currentPanel.right.panel.translateX = 0; | ||
@@ -403,6 +418,6 @@ } | ||
else { | ||
if (!_this.transitioning | ||
&& previousDelta !== args.deltaX | ||
&& args.deltaX != null | ||
&& args.deltaX < 0) { | ||
if (!_this.transitioning && | ||
previousDelta !== args.deltaX && | ||
args.deltaX != null && | ||
args.deltaX < 0) { | ||
if (_this.hasNext) { | ||
@@ -414,10 +429,11 @@ _this.direction = direction.left; | ||
} | ||
else if (!_this.transitioning | ||
&& previousDelta !== args.deltaX | ||
&& args.deltaX != null | ||
&& args.deltaX > 0) { | ||
else if (!_this.transitioning && | ||
previousDelta !== args.deltaX && | ||
args.deltaX != null && | ||
args.deltaX > 0) { | ||
if (_this.hasPrevious) { | ||
_this.direction = direction.right; | ||
_this.currentPanel.panel.translateX = args.deltaX - _this.pageWidth; | ||
_this.currentPanel.left.panel.translateX = -(_this.pageWidth * 2) + args.deltaX; | ||
_this.currentPanel.left.panel.translateX = | ||
-(_this.pageWidth * 2) + args.deltaX; | ||
} | ||
@@ -512,3 +528,3 @@ } | ||
panel: view, | ||
index: index, | ||
index: index | ||
}); | ||
@@ -522,3 +538,3 @@ }); | ||
}); | ||
if (this.loop) { | ||
if (this.loop === true) { | ||
this._slideMap[0].left = this._slideMap[this._slideMap.length - 1]; | ||
@@ -588,3 +604,3 @@ this._slideMap[this._slideMap.length - 1].right = this._slideMap[0]; | ||
SlideContainer.prototype.iosProperty = function (theClass, theProperty) { | ||
if (typeof theProperty === "function") { | ||
if (typeof theProperty === 'function') { | ||
// xCode 7 and below | ||
@@ -598,8 +614,8 @@ return theProperty.call(theClass); | ||
}; | ||
SlideContainer.startEvent = 'start'; | ||
SlideContainer.changedEvent = 'changed'; | ||
SlideContainer.cancelledEvent = 'cancelled'; | ||
SlideContainer.finishedEvent = 'finished'; | ||
return SlideContainer; | ||
}(absolute_layout_1.AbsoluteLayout)); | ||
SlideContainer.startEvent = 'start'; | ||
SlideContainer.changedEvent = 'changed'; | ||
SlideContainer.cancelledEvent = 'cancelled'; | ||
SlideContainer.finishedEvent = 'finished'; | ||
exports.SlideContainer = SlideContainer; |
@@ -1,2 +0,2 @@ | ||
require("nativescript-dom"); | ||
require('nativescript-dom'); | ||
import * as app from 'application'; | ||
@@ -24,615 +24,656 @@ import * as Platform from 'platform'; | ||
if (app.android) { | ||
LayoutParams = <any>android.view.WindowManager.LayoutParams; | ||
LayoutParams = <any>android.view.WindowManager.LayoutParams; | ||
} else { | ||
LayoutParams = {}; | ||
LayoutParams = {}; | ||
} | ||
export class Slide extends StackLayout { } | ||
export class Slide extends StackLayout {} | ||
enum direction { | ||
none, | ||
left, | ||
right | ||
none, | ||
left, | ||
right | ||
} | ||
enum cancellationReason { | ||
user, | ||
noPrevSlides, | ||
noMoreSlides | ||
user, | ||
noPrevSlides, | ||
noMoreSlides | ||
} | ||
export interface ISlideMap { | ||
panel: StackLayout; | ||
index: number; | ||
left?: ISlideMap; | ||
right?: ISlideMap; | ||
panel: StackLayout; | ||
index: number; | ||
left?: ISlideMap; | ||
right?: ISlideMap; | ||
} | ||
export class SlideContainer extends AbsoluteLayout { | ||
private currentPanel: ISlideMap; | ||
private transitioning: boolean = false; | ||
private direction: direction = direction.none; | ||
private _loaded: boolean; | ||
private _pageWidth: number; | ||
private _loop: boolean; | ||
private _pagerOffset: string; | ||
private _angular: boolean; | ||
private _disablePan: boolean; | ||
private _footer: StackLayout; | ||
private _pageIndicators: boolean; | ||
private _slideMap: ISlideMap[]; | ||
private _slideWidth: string; | ||
private currentPanel: ISlideMap; | ||
private transitioning: boolean = false; | ||
private direction: direction = direction.none; | ||
private _loaded: boolean; | ||
private _pageWidth: number; | ||
private _loop: boolean; | ||
private _pagerOffset: string; | ||
private _angular: boolean; | ||
private _disablePan: boolean; | ||
private _footer: StackLayout; | ||
private _pageIndicators: boolean; | ||
private _slideMap: ISlideMap[]; | ||
private _slideWidth: string; | ||
public static startEvent = 'start'; | ||
public static changedEvent = 'changed'; | ||
public static cancelledEvent = 'cancelled'; | ||
public static finishedEvent = 'finished'; | ||
public static startEvent = 'start'; | ||
public static changedEvent = 'changed'; | ||
public static cancelledEvent = 'cancelled'; | ||
public static finishedEvent = 'finished'; | ||
/* page indicator stuff*/ | ||
get pageIndicators(): boolean { | ||
return this._pageIndicators; | ||
} | ||
set pageIndicators(value: boolean) { | ||
if (typeof value === 'string') { | ||
value = (<any>value == 'true'); | ||
} | ||
this._pageIndicators = value; | ||
} | ||
/* page indicator stuff*/ | ||
get pageIndicators(): boolean { | ||
return this._pageIndicators; | ||
} | ||
set pageIndicators(value: boolean) { | ||
if (typeof value === 'string') { | ||
value = <any>value == 'true'; | ||
} | ||
this._pageIndicators = value; | ||
} | ||
get pagerOffset(): string { | ||
return this._pagerOffset; | ||
} | ||
set pagerOffset(value: string) { | ||
this._pagerOffset = value; | ||
} | ||
get pagerOffset(): string { | ||
return this._pagerOffset; | ||
} | ||
set pagerOffset(value: string) { | ||
this._pagerOffset = value; | ||
} | ||
get hasNext(): boolean { | ||
return !!this.currentPanel && !!this.currentPanel.right; | ||
} | ||
get hasPrevious(): boolean { | ||
return !!this.currentPanel && !!this.currentPanel.left; | ||
} | ||
get hasNext(): boolean { | ||
return !!this.currentPanel && !!this.currentPanel.right; | ||
} | ||
get hasPrevious(): boolean { | ||
return !!this.currentPanel && !!this.currentPanel.left; | ||
} | ||
get loop() { | ||
return this._loop; | ||
} | ||
get loop() { | ||
return this._loop; | ||
} | ||
set loop(value: boolean) { | ||
this._loop = value; | ||
} | ||
set loop(value: boolean) { | ||
this._loop = value; | ||
} | ||
get disablePan() { | ||
return this._disablePan; | ||
} | ||
get disablePan() { | ||
return this._disablePan; | ||
} | ||
set disablePan(value: boolean) { | ||
this._disablePan = value; | ||
} | ||
set disablePan(value: boolean) { | ||
if (this._disablePan === value) { | ||
return; | ||
} // Value did not change | ||
get pageWidth() { | ||
if (!this.slideWidth) { | ||
return Platform.screen.mainScreen.widthDIPs; | ||
} | ||
return +this.slideWidth; | ||
} | ||
this._disablePan = value; | ||
if (this._loaded && this.currentPanel.panel !== undefined) { | ||
if (value === true) { | ||
this.currentPanel.panel.off('pan'); | ||
} else if (value === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
} | ||
} | ||
get angular(): boolean { | ||
return this._angular; | ||
} | ||
get pageWidth() { | ||
if (!this.slideWidth) { | ||
return Platform.screen.mainScreen.widthDIPs; | ||
} | ||
return +this.slideWidth; | ||
} | ||
set angular(value: boolean) { | ||
this._angular = value; | ||
} | ||
get angular(): boolean { | ||
return this._angular; | ||
} | ||
get currentIndex(): number { | ||
return this.currentPanel.index; | ||
} | ||
set angular(value: boolean) { | ||
this._angular = value; | ||
} | ||
get slideWidth(): string { | ||
return this._slideWidth; | ||
} | ||
set slideWidth(width: string) { | ||
this._slideWidth = width; | ||
} | ||
get currentIndex(): number { | ||
return this.currentPanel.index; | ||
} | ||
constructor() { | ||
super(); | ||
this.setupDefaultValues(); | ||
// if being used in an ng2 app we want to prevent it from excuting the constructView | ||
// until it is called manually in ngAfterViewInit. | ||
get slideWidth(): string { | ||
return this._slideWidth; | ||
} | ||
set slideWidth(width: string) { | ||
this._slideWidth = width; | ||
} | ||
this.constructView(true); | ||
} | ||
constructor() { | ||
super(); | ||
this.setupDefaultValues(); | ||
// if being used in an ng2 app we want to prevent it from excuting the constructView | ||
// until it is called manually in ngAfterViewInit. | ||
private setupDefaultValues(): void { | ||
this.clipToBounds = true; | ||
this.constructView(true); | ||
} | ||
private setupDefaultValues(): void { | ||
this.clipToBounds = true; | ||
this._loaded = false; | ||
if (this._loop == null) { | ||
this.loop = false; | ||
} | ||
this._loaded = false; | ||
if (this._loop == null) { | ||
this.loop = false; | ||
} | ||
this.transitioning = false; | ||
this.transitioning = false; | ||
if (this._disablePan == null) { | ||
this.disablePan = false; | ||
} | ||
if (this._disablePan == null) { | ||
this.disablePan = false; | ||
} | ||
if (this._angular == null) { | ||
this.angular = false; | ||
} | ||
if (this._angular == null) { | ||
this.angular = false; | ||
} | ||
if (this._pageIndicators == null) { | ||
this._pageIndicators = false; | ||
} | ||
if (this._pageIndicators == null) { | ||
this._pageIndicators = false; | ||
} | ||
if (this._pagerOffset == null) { | ||
this._pagerOffset = '88%'; //defaults to white. | ||
} | ||
} | ||
if (this._pagerOffset == null) { | ||
this._pagerOffset = '88%'; //defaults to white. | ||
} | ||
} | ||
public constructView(constructor: boolean = false): void { | ||
this.on(AbsoluteLayout.loadedEvent, (data: any) => { | ||
//// console.log('LOADDED EVENT'); | ||
if (!this._loaded) { | ||
this._loaded = true; | ||
if (this.angular === true && constructor === true) { | ||
return; | ||
} | ||
public constructView(constructor: boolean = false): void { | ||
this.on(AbsoluteLayout.loadedEvent, (data: any) => { | ||
//// console.log('LOADDED EVENT'); | ||
if (!this._loaded) { | ||
this._loaded = true; | ||
if (this.angular === true && constructor === true) { | ||
return; | ||
} | ||
let slides: StackLayout[] = []; | ||
let slides: StackLayout[] = []; | ||
if (!this.slideWidth) { | ||
this.slideWidth = <any>this.pageWidth; | ||
} | ||
this.width = +(this.slideWidth); | ||
if (!this.slideWidth) { | ||
this.slideWidth = <any>this.pageWidth; | ||
} | ||
this.width = +this.slideWidth; | ||
this.eachLayoutChild((view: View) => { | ||
if (view instanceof StackLayout) { | ||
AbsoluteLayout.setLeft(view, this.pageWidth); | ||
view.width = this.pageWidth; | ||
(<any>view).height = '100%'; //get around compiler | ||
slides.push(view); | ||
} | ||
}); | ||
this.eachLayoutChild((view: View) => { | ||
if (view instanceof StackLayout) { | ||
AbsoluteLayout.setLeft(view, this.pageWidth); | ||
view.width = this.pageWidth; | ||
(<any>view).height = '100%'; //get around compiler | ||
slides.push(view); | ||
} | ||
}); | ||
if (this.pageIndicators) { | ||
this._footer = this.buildFooter(slides.length, 0); | ||
this.setActivePageIndicator(0); | ||
this.insertChild(this._footer, this.getChildrenCount()); | ||
} | ||
if (this.pageIndicators) { | ||
this._footer = this.buildFooter(slides.length, 0); | ||
this.setActivePageIndicator(0); | ||
this.insertChild(this._footer, this.getChildrenCount()); | ||
} | ||
this.currentPanel = this.buildSlideMap(slides); | ||
if (this.currentPanel) { | ||
this.currentPanel = this.buildSlideMap(slides); | ||
if (this.currentPanel) { | ||
this.positionPanels(this.currentPanel); | ||
this.positionPanels(this.currentPanel); | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (app.ios) { | ||
this.ios.clipsToBound = true; | ||
} | ||
//handles application orientation change | ||
app.on( | ||
app.orientationChangedEvent, | ||
(args: app.OrientationChangedEventData) => { | ||
//event and page orientation didn't seem to alwasy be on the same page so setting it in the time out addresses this. | ||
setTimeout(() => { | ||
// console.log('orientationChangedEvent'); | ||
this.width = parseInt(this.slideWidth); | ||
this.eachLayoutChild((view: View) => { | ||
if (view instanceof StackLayout) { | ||
AbsoluteLayout.setLeft(view, this.pageWidth); | ||
view.width = this.pageWidth; | ||
} | ||
}); | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (app.ios) { | ||
this.ios.clipsToBound = true; | ||
} | ||
//handles application orientation change | ||
app.on(app.orientationChangedEvent, (args: app.OrientationChangedEventData) => { | ||
//event and page orientation didn't seem to alwasy be on the same page so setting it in the time out addresses this. | ||
setTimeout(() => { | ||
// console.log('orientationChangedEvent'); | ||
this.width = parseInt(this.slideWidth); | ||
this.eachLayoutChild((view: View) => { | ||
if (view instanceof StackLayout) { | ||
AbsoluteLayout.setLeft(view, this.pageWidth); | ||
view.width = this.pageWidth; | ||
} | ||
}); | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (this.pageIndicators) { | ||
AbsoluteLayout.setTop(this._footer, 0); | ||
var pageIndicatorsLeftOffset = this.pageWidth / 4; | ||
AbsoluteLayout.setLeft( | ||
this._footer, | ||
pageIndicatorsLeftOffset | ||
); | ||
this._footer.width = this.pageWidth / 2; | ||
this._footer.marginTop = <any>this._pagerOffset; | ||
} | ||
if (this.pageIndicators) { | ||
AbsoluteLayout.setTop(this._footer, 0); | ||
var pageIndicatorsLeftOffset = this.pageWidth / 4; | ||
AbsoluteLayout.setLeft(this._footer, pageIndicatorsLeftOffset); | ||
this._footer.width = this.pageWidth / 2; | ||
this._footer.marginTop = <any>this._pagerOffset; | ||
} | ||
this.positionPanels(this.currentPanel); | ||
}, 0); | ||
} | ||
); | ||
} | ||
} | ||
}); | ||
} | ||
this.positionPanels(this.currentPanel); | ||
}, 0); | ||
}); | ||
} | ||
} | ||
}); | ||
} | ||
public nextSlide(): void { | ||
if (!this.hasNext) { | ||
this.triggerCancelEvent(cancellationReason.noMoreSlides); | ||
return; | ||
} | ||
public nextSlide(): void { | ||
if (!this.hasNext) { | ||
this.triggerCancelEvent(cancellationReason.noMoreSlides); | ||
return; | ||
} | ||
this.direction = direction.left; | ||
this.transitioning = true; | ||
this.triggerStartEvent(); | ||
this.showRightSlide(this.currentPanel).then(() => { | ||
this.setupPanel(this.currentPanel.right); | ||
this.triggerChangeEventRightToLeft(); | ||
}); | ||
} | ||
public previousSlide(): void { | ||
if (!this.hasPrevious) { | ||
this.triggerCancelEvent(cancellationReason.noPrevSlides); | ||
return; | ||
} | ||
this.direction = direction.left; | ||
this.transitioning = true; | ||
this.triggerStartEvent(); | ||
this.showRightSlide(this.currentPanel).then(() => { | ||
this.setupPanel(this.currentPanel.right); | ||
this.triggerChangeEventRightToLeft(); | ||
}); | ||
} | ||
public previousSlide(): void { | ||
if (!this.hasPrevious) { | ||
this.triggerCancelEvent(cancellationReason.noPrevSlides); | ||
return; | ||
} | ||
this.direction = direction.right; | ||
this.transitioning = true; | ||
this.triggerStartEvent(); | ||
this.showLeftSlide(this.currentPanel).then(() => { | ||
this.setupPanel(this.currentPanel.left); | ||
this.triggerChangeEventLeftToRight(); | ||
}); | ||
} | ||
this.direction = direction.right; | ||
this.transitioning = true; | ||
this.triggerStartEvent(); | ||
this.showLeftSlide(this.currentPanel).then(() => { | ||
this.setupPanel(this.currentPanel.left); | ||
this.triggerChangeEventLeftToRight(); | ||
}); | ||
} | ||
private setupPanel(panel: ISlideMap) { | ||
this.direction = direction.none; | ||
this.transitioning = false; | ||
this.currentPanel.panel.off('pan'); | ||
this.currentPanel = panel; | ||
private setupPanel(panel: ISlideMap) { | ||
this.direction = direction.none; | ||
this.transitioning = false; | ||
this.currentPanel.panel.off('pan'); | ||
this.currentPanel = panel; | ||
// sets up each panel so that they are positioned to transition either way. | ||
this.positionPanels(this.currentPanel); | ||
// sets up each panel so that they are positioned to transition either way. | ||
this.positionPanels(this.currentPanel); | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (this.disablePan === false) { | ||
this.applySwipe(this.pageWidth); | ||
} | ||
if (this.pageIndicators) { | ||
this.setActivePageIndicator(this.currentPanel.index); | ||
} | ||
} | ||
if (this.pageIndicators) { | ||
this.setActivePageIndicator(this.currentPanel.index); | ||
} | ||
} | ||
private positionPanels(panel: ISlideMap) { | ||
// sets up each panel so that they are positioned to transition either way. | ||
if (panel.left != null) { | ||
panel.left.panel.translateX = -this.pageWidth * 2; | ||
} | ||
panel.panel.translateX = -this.pageWidth; | ||
if (panel.right != null) { | ||
panel.right.panel.translateX = 0; | ||
} | ||
} | ||
private positionPanels(panel: ISlideMap) { | ||
// sets up each panel so that they are positioned to transition either way. | ||
if (panel.left != null) { | ||
panel.left.panel.translateX = -this.pageWidth * 2; | ||
} | ||
panel.panel.translateX = -this.pageWidth; | ||
if (panel.right != null) { | ||
panel.right.panel.translateX = 0; | ||
} | ||
} | ||
public goToSlide(index: number): void { | ||
if ( | ||
this._slideMap && | ||
this._slideMap.length > 0 && | ||
index < this._slideMap.length | ||
) { | ||
let previousSlide = this.currentPanel; | ||
public goToSlide(index: number): void { | ||
if (this._slideMap && this._slideMap.length > 0 && index < this._slideMap.length) { | ||
let previousSlide = this.currentPanel; | ||
this.setupPanel(this._slideMap[index]); | ||
this.setupPanel(this._slideMap[index]); | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.none, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: previousSlide.index | ||
} | ||
}); | ||
} else { | ||
// console.log('invalid index'); | ||
} | ||
} | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.none, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: previousSlide.index, | ||
} | ||
}); | ||
} else { | ||
// console.log('invalid index'); | ||
} | ||
} | ||
public applySwipe(pageWidth: number): void { | ||
let previousDelta = -1; //hack to get around ios firing pan event after release | ||
let endingVelocity = 0; | ||
let startTime, deltaTime; | ||
public applySwipe(pageWidth: number): void { | ||
let previousDelta = -1; //hack to get around ios firing pan event after release | ||
let endingVelocity = 0; | ||
let startTime, deltaTime; | ||
this.currentPanel.panel.on( | ||
'pan', | ||
(args: gestures.PanGestureEventData): void => { | ||
if (args.state === gestures.GestureStateTypes.began) { | ||
startTime = Date.now(); | ||
previousDelta = 0; | ||
endingVelocity = 250; | ||
this.currentPanel.panel.on('pan', (args: gestures.PanGestureEventData): void => { | ||
if (args.state === gestures.GestureStateTypes.began) { | ||
startTime = Date.now(); | ||
previousDelta = 0; | ||
endingVelocity = 250; | ||
this.triggerStartEvent(); | ||
} else if (args.state === gestures.GestureStateTypes.ended) { | ||
deltaTime = Date.now() - startTime; | ||
// if velocityScrolling is enabled then calculate the velocitty | ||
this.triggerStartEvent(); | ||
} else if (args.state === gestures.GestureStateTypes.ended) { | ||
deltaTime = Date.now() - startTime; | ||
// if velocityScrolling is enabled then calculate the velocitty | ||
// swiping left to right. | ||
if (args.deltaX > pageWidth / 3) { | ||
if (this.hasPrevious) { | ||
this.transitioning = true; | ||
this.showLeftSlide( | ||
this.currentPanel, | ||
args.deltaX, | ||
endingVelocity | ||
).then(() => { | ||
this.setupPanel(this.currentPanel.left); | ||
// swiping left to right. | ||
if (args.deltaX > (pageWidth / 3)) { | ||
if (this.hasPrevious) { | ||
this.transitioning = true; | ||
this.showLeftSlide(this.currentPanel, args.deltaX, endingVelocity).then(() => { | ||
this.setupPanel(this.currentPanel.left); | ||
this.triggerChangeEventLeftToRight(); | ||
}); | ||
} else { | ||
//We're at the start | ||
//Notify no more slides | ||
this.triggerCancelEvent(cancellationReason.noPrevSlides); | ||
} | ||
return; | ||
} | ||
// swiping right to left | ||
else if (args.deltaX < -pageWidth / 3) { | ||
if (this.hasNext) { | ||
this.transitioning = true; | ||
this.showRightSlide( | ||
this.currentPanel, | ||
args.deltaX, | ||
endingVelocity | ||
).then(() => { | ||
this.setupPanel(this.currentPanel.right); | ||
this.triggerChangeEventLeftToRight(); | ||
}); | ||
} else { | ||
//We're at the start | ||
//Notify no more slides | ||
this.triggerCancelEvent(cancellationReason.noPrevSlides); | ||
} | ||
return; | ||
} | ||
// swiping right to left | ||
else if (args.deltaX < (-pageWidth / 3)) { | ||
if (this.hasNext) { | ||
this.transitioning = true; | ||
this.showRightSlide(this.currentPanel, args.deltaX, endingVelocity).then(() => { | ||
this.setupPanel(this.currentPanel.right); | ||
// Notify changed | ||
this.triggerChangeEventRightToLeft(); | ||
// Notify changed | ||
this.triggerChangeEventRightToLeft(); | ||
if (!this.hasNext) { | ||
// Notify finsihed | ||
this.notify({ | ||
eventName: SlideContainer.finishedEvent, | ||
object: this | ||
}); | ||
} | ||
}); | ||
} else { | ||
// We're at the end | ||
// Notify no more slides | ||
this.triggerCancelEvent(cancellationReason.noMoreSlides); | ||
} | ||
return; | ||
} | ||
if (!this.hasNext) { | ||
// Notify finsihed | ||
this.notify({ | ||
eventName: SlideContainer.finishedEvent, | ||
object: this | ||
}); | ||
} | ||
}); | ||
} else { | ||
// We're at the end | ||
// Notify no more slides | ||
this.triggerCancelEvent(cancellationReason.noMoreSlides); | ||
} | ||
return; | ||
} | ||
if (this.transitioning === false) { | ||
//Notify cancelled | ||
this.triggerCancelEvent(cancellationReason.user); | ||
this.transitioning = true; | ||
this.currentPanel.panel.animate({ | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (this.hasNext) { | ||
this.currentPanel.right.panel.animate({ | ||
translate: { x: 0, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (app.ios) | ||
//for some reason i have to set these in ios or there is some sort of bounce back. | ||
this.currentPanel.right.panel.translateX = 0; | ||
} | ||
if (this.hasPrevious) { | ||
this.currentPanel.left.panel.animate({ | ||
translate: { x: -this.pageWidth * 2, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (app.ios) | ||
this.currentPanel.left.panel.translateX = -this.pageWidth; | ||
} | ||
if (app.ios) this.currentPanel.panel.translateX = -this.pageWidth; | ||
if (this.transitioning === false) { | ||
//Notify cancelled | ||
this.triggerCancelEvent(cancellationReason.user); | ||
this.transitioning = true; | ||
this.currentPanel.panel.animate({ | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (this.hasNext) { | ||
this.currentPanel.right.panel.animate({ | ||
translate: { x: 0, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (app.ios) //for some reason i have to set these in ios or there is some sort of bounce back. | ||
this.currentPanel.right.panel.translateX = 0; | ||
} | ||
if (this.hasPrevious) { | ||
this.currentPanel.left.panel.animate({ | ||
translate: { x: -this.pageWidth * 2, y: 0 }, | ||
duration: 200, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
if (app.ios) | ||
this.currentPanel.left.panel.translateX = -this.pageWidth; | ||
this.transitioning = false; | ||
} | ||
} else { | ||
if ( | ||
!this.transitioning && | ||
previousDelta !== args.deltaX && | ||
args.deltaX != null && | ||
args.deltaX < 0 | ||
) { | ||
if (this.hasNext) { | ||
this.direction = direction.left; | ||
this.currentPanel.panel.translateX = args.deltaX - this.pageWidth; | ||
this.currentPanel.right.panel.translateX = args.deltaX; | ||
} | ||
} else if ( | ||
!this.transitioning && | ||
previousDelta !== args.deltaX && | ||
args.deltaX != null && | ||
args.deltaX > 0 | ||
) { | ||
if (this.hasPrevious) { | ||
this.direction = direction.right; | ||
this.currentPanel.panel.translateX = args.deltaX - this.pageWidth; | ||
this.currentPanel.left.panel.translateX = | ||
-(this.pageWidth * 2) + args.deltaX; | ||
} | ||
} | ||
} | ||
if (app.ios) | ||
this.currentPanel.panel.translateX = -this.pageWidth; | ||
if (args.deltaX !== 0) { | ||
previousDelta = args.deltaX; | ||
} | ||
} | ||
} | ||
); | ||
} | ||
this.transitioning = false; | ||
} | ||
} else { | ||
if (!this.transitioning | ||
&& previousDelta !== args.deltaX | ||
&& args.deltaX != null | ||
&& args.deltaX < 0) { | ||
private showRightSlide( | ||
panelMap: ISlideMap, | ||
offset: number = this.pageWidth, | ||
endingVelocity: number = 32 | ||
): AnimationModule.AnimationPromise { | ||
let animationDuration: number; | ||
animationDuration = 300; // default value | ||
if (this.hasNext) { | ||
this.direction = direction.left; | ||
this.currentPanel.panel.translateX = args.deltaX - this.pageWidth; | ||
this.currentPanel.right.panel.translateX = args.deltaX; | ||
let transition = new Array(); | ||
} | ||
} else if (!this.transitioning | ||
&& previousDelta !== args.deltaX | ||
&& args.deltaX != null | ||
&& args.deltaX > 0) { | ||
transition.push({ | ||
target: panelMap.right.panel, | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
transition.push({ | ||
target: panelMap.panel, | ||
translate: { x: -this.pageWidth * 2, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
let animationSet = new AnimationModule.Animation(transition, false); | ||
if (this.hasPrevious) { | ||
this.direction = direction.right; | ||
this.currentPanel.panel.translateX = args.deltaX - this.pageWidth; | ||
this.currentPanel.left.panel.translateX = -(this.pageWidth * 2) + args.deltaX; | ||
} | ||
} | ||
return animationSet.play(); | ||
} | ||
if (args.deltaX !== 0) { | ||
previousDelta = args.deltaX; | ||
} | ||
private showLeftSlide( | ||
panelMap: ISlideMap, | ||
offset: number = this.pageWidth, | ||
endingVelocity: number = 32 | ||
): AnimationModule.AnimationPromise { | ||
let animationDuration: number; | ||
animationDuration = 300; // default value | ||
let transition = new Array(); | ||
} | ||
}); | ||
} | ||
transition.push({ | ||
target: panelMap.left.panel, | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
transition.push({ | ||
target: panelMap.panel, | ||
translate: { x: 0, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
let animationSet = new AnimationModule.Animation(transition, false); | ||
private showRightSlide(panelMap: ISlideMap, offset: number = this.pageWidth, endingVelocity: number = 32): AnimationModule.AnimationPromise { | ||
let animationDuration: number; | ||
animationDuration = 300; // default value | ||
return animationSet.play(); | ||
} | ||
let transition = new Array(); | ||
private buildFooter( | ||
pageCount: number = 5, | ||
activeIndex: number = 0 | ||
): StackLayout { | ||
let footerInnerWrap = new StackLayout(); | ||
transition.push({ | ||
target: panelMap.right.panel, | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
transition.push({ | ||
target: panelMap.panel, | ||
translate: { x: -this.pageWidth * 2, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
let animationSet = new AnimationModule.Animation(transition, false); | ||
//footerInnerWrap.height = 50; | ||
if (app.ios) { | ||
footerInnerWrap.clipToBounds = false; | ||
} | ||
footerInnerWrap.className = SLIDE_INDICATOR_WRAP; | ||
return animationSet.play(); | ||
} | ||
AbsoluteLayout.setTop(footerInnerWrap, 0); | ||
private showLeftSlide(panelMap: ISlideMap, offset: number = this.pageWidth, endingVelocity: number = 32): AnimationModule.AnimationPromise { | ||
footerInnerWrap.orientation = 'horizontal'; | ||
footerInnerWrap.horizontalAlignment = 'center'; | ||
footerInnerWrap.width = this.pageWidth / 2; | ||
let animationDuration: number; | ||
animationDuration = 300; // default value | ||
let transition = new Array(); | ||
let index = 0; | ||
while (index < pageCount) { | ||
footerInnerWrap.addChild(this.createIndicator(index)); | ||
index++; | ||
} | ||
transition.push({ | ||
target: panelMap.left.panel, | ||
translate: { x: -this.pageWidth, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
transition.push({ | ||
target: panelMap.panel, | ||
translate: { x: 0, y: 0 }, | ||
duration: animationDuration, | ||
curve: AnimationCurve.easeOut | ||
}); | ||
let animationSet = new AnimationModule.Animation(transition, false); | ||
let pageIndicatorsLeftOffset = this.pageWidth / 4; | ||
AbsoluteLayout.setLeft(footerInnerWrap, pageIndicatorsLeftOffset); | ||
footerInnerWrap.marginTop = <any>this._pagerOffset; | ||
return animationSet.play(); | ||
return footerInnerWrap; | ||
} | ||
} | ||
private setwidthPercent(view: View, percentage: number) { | ||
(<any>view).width = percentage + '%'; | ||
} | ||
private buildFooter(pageCount: number = 5, activeIndex: number = 0): StackLayout { | ||
let footerInnerWrap = new StackLayout(); | ||
private newFooterButton(name: string): Button { | ||
let button = new Button(); | ||
button.id = 'btn-info-' + name.toLowerCase(); | ||
button.text = name; | ||
this.setwidthPercent(button, 100); | ||
return button; | ||
} | ||
//footerInnerWrap.height = 50; | ||
if (app.ios) { | ||
footerInnerWrap.clipToBounds = false; | ||
} | ||
footerInnerWrap.className = SLIDE_INDICATOR_WRAP; | ||
private buildSlideMap(views: StackLayout[]) { | ||
this._slideMap = []; | ||
views.forEach((view: StackLayout, index: number) => { | ||
this._slideMap.push({ | ||
panel: view, | ||
index: index | ||
}); | ||
}); | ||
this._slideMap.forEach((mapping: ISlideMap, index: number) => { | ||
if (this._slideMap[index - 1] != null) | ||
mapping.left = this._slideMap[index - 1]; | ||
if (this._slideMap[index + 1] != null) | ||
mapping.right = this._slideMap[index + 1]; | ||
}); | ||
if (this.loop === true) { | ||
this._slideMap[0].left = this._slideMap[this._slideMap.length - 1]; | ||
this._slideMap[this._slideMap.length - 1].right = this._slideMap[0]; | ||
} | ||
return this._slideMap[0]; | ||
} | ||
AbsoluteLayout.setTop(footerInnerWrap, 0); | ||
private triggerStartEvent() { | ||
this.notify({ | ||
eventName: SlideContainer.startEvent, | ||
object: this, | ||
eventData: { | ||
currentIndex: this.currentPanel.index | ||
} | ||
}); | ||
} | ||
footerInnerWrap.orientation = 'horizontal'; | ||
footerInnerWrap.horizontalAlignment = 'center'; | ||
footerInnerWrap.width = this.pageWidth / 2; | ||
private triggerChangeEventLeftToRight() { | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.left, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: this.currentPanel.index + 1 | ||
} | ||
}); | ||
} | ||
let index = 0; | ||
while (index < pageCount) { | ||
footerInnerWrap.addChild(this.createIndicator(index)); | ||
index++; | ||
} | ||
private triggerChangeEventRightToLeft() { | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.right, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: this.currentPanel.index - 1 | ||
} | ||
}); | ||
} | ||
let pageIndicatorsLeftOffset = this.pageWidth / 4; | ||
AbsoluteLayout.setLeft(footerInnerWrap, pageIndicatorsLeftOffset); | ||
footerInnerWrap.marginTop = <any>this._pagerOffset; | ||
private triggerCancelEvent(cancelReason: cancellationReason) { | ||
this.notify({ | ||
eventName: SlideContainer.cancelledEvent, | ||
object: this, | ||
eventData: { | ||
currentIndex: this.currentPanel.index, | ||
reason: cancelReason | ||
} | ||
}); | ||
} | ||
return footerInnerWrap; | ||
} | ||
createIndicator(index: number): Label { | ||
let indicator = new Label(); | ||
private setwidthPercent(view: View, percentage: number) { | ||
(<any>view).width = percentage + '%'; | ||
} | ||
(<any>indicator).classList.add(SLIDE_INDICATOR_INACTIVE); | ||
return indicator; | ||
} | ||
private newFooterButton(name: string): Button { | ||
let button = new Button(); | ||
button.id = 'btn-info-' + name.toLowerCase(); | ||
button.text = name; | ||
this.setwidthPercent(button, 100); | ||
return button; | ||
} | ||
setActivePageIndicator(index: number) { | ||
let indicatorsToDeactivate = (<any>this._footer).getElementsByClassName( | ||
SLIDE_INDICATOR_ACTIVE | ||
); | ||
private buildSlideMap(views: StackLayout[]) { | ||
this._slideMap = []; | ||
views.forEach((view: StackLayout, index: number) => { | ||
this._slideMap.push({ | ||
panel: view, | ||
index: index, | ||
}); | ||
}); | ||
this._slideMap.forEach((mapping: ISlideMap, index: number) => { | ||
if (this._slideMap[index - 1] != null) | ||
mapping.left = this._slideMap[index - 1]; | ||
if (this._slideMap[index + 1] != null) | ||
mapping.right = this._slideMap[index + 1]; | ||
}); | ||
indicatorsToDeactivate.forEach(activeIndicator => { | ||
activeIndicator.classList.remove(SLIDE_INDICATOR_ACTIVE); | ||
activeIndicator.classList.add(SLIDE_INDICATOR_INACTIVE); | ||
}); | ||
if (this.loop) { | ||
this._slideMap[0].left = this._slideMap[this._slideMap.length - 1]; | ||
this._slideMap[this._slideMap.length - 1].right = this._slideMap[0]; | ||
} | ||
return this._slideMap[0]; | ||
} | ||
let activeIndicator = (<any>this._footer).getElementsByClassName( | ||
SLIDE_INDICATOR_INACTIVE | ||
)[index]; | ||
if (activeIndicator) { | ||
activeIndicator.classList.remove(SLIDE_INDICATOR_INACTIVE); | ||
activeIndicator.classList.add(SLIDE_INDICATOR_ACTIVE); | ||
} | ||
} | ||
private triggerStartEvent() { | ||
this.notify({ | ||
eventName: SlideContainer.startEvent, | ||
object: this, | ||
eventData: { | ||
currentIndex: this.currentPanel.index | ||
} | ||
}); | ||
} | ||
private triggerChangeEventLeftToRight() { | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.left, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: this.currentPanel.index + 1 | ||
} | ||
}); | ||
} | ||
private triggerChangeEventRightToLeft() { | ||
this.notify({ | ||
eventName: SlideContainer.changedEvent, | ||
object: this, | ||
eventData: { | ||
direction: direction.right, | ||
newIndex: this.currentPanel.index, | ||
oldIndex: this.currentPanel.index - 1 | ||
} | ||
}); | ||
} | ||
private triggerCancelEvent(cancelReason: cancellationReason) { | ||
this.notify({ | ||
eventName: SlideContainer.cancelledEvent, | ||
object: this, | ||
eventData: { | ||
currentIndex: this.currentPanel.index, | ||
reason: cancelReason | ||
} | ||
}); | ||
} | ||
createIndicator(index: number): Label { | ||
let indicator = new Label(); | ||
(<any>indicator).classList.add(SLIDE_INDICATOR_INACTIVE); | ||
return indicator; | ||
} | ||
setActivePageIndicator(index: number) { | ||
let indicatorsToDeactivate = (<any>this._footer).getElementsByClassName(SLIDE_INDICATOR_ACTIVE); | ||
indicatorsToDeactivate.forEach(activeIndicator => { | ||
activeIndicator.classList.remove(SLIDE_INDICATOR_ACTIVE); | ||
activeIndicator.classList.add(SLIDE_INDICATOR_INACTIVE); | ||
}); | ||
let activeIndicator = (<any>this._footer).getElementsByClassName(SLIDE_INDICATOR_INACTIVE)[index]; | ||
if (activeIndicator) { | ||
activeIndicator.classList.remove(SLIDE_INDICATOR_INACTIVE); | ||
activeIndicator.classList.add(SLIDE_INDICATOR_ACTIVE); | ||
} | ||
} | ||
iosProperty(theClass, theProperty) { | ||
if (typeof theProperty === "function") { | ||
// xCode 7 and below | ||
return theProperty.call(theClass); | ||
} else { | ||
// xCode 8+ | ||
return theProperty; | ||
} | ||
} | ||
iosProperty(theClass, theProperty) { | ||
if (typeof theProperty === 'function') { | ||
// xCode 7 and below | ||
return theProperty.call(theClass); | ||
} else { | ||
// xCode 8+ | ||
return theProperty; | ||
} | ||
} | ||
} |
{ | ||
"name": "nativescript-slides", | ||
"version": "2.2.14", | ||
"version": "2.3.0", | ||
"description": "NativeScript Slides plugin.", | ||
@@ -9,12 +9,13 @@ "main": "nativescript-slides", | ||
"platforms": { | ||
"android": "3.0.0", | ||
"ios": "3.0.0" | ||
"android": "5.0.0", | ||
"ios": "5.0.0" | ||
} | ||
}, | ||
"scripts": { | ||
"prepublish": "cp -av ../README.md ./", | ||
"build": "tsc", | ||
"demo.ios": "npm run preparedemo && cd demo && tns run ios", | ||
"demo.android": "npm run preparedemo && cd demo && tns run android", | ||
"preparedemo": "npm run build && cd demo && npm install .. --save", | ||
"setup": "npm install && cd demo && npm install && cd .. && npm run build && cd demo && npm install .. -S && cd ..", | ||
"demo.ios": "npm run preparedemo && cd ../demo && tns run ios", | ||
"demo.android": "npm run preparedemo && cd ../demo && tns run android", | ||
"preparedemo": "npm run build && cd ../demo && npm install ../src --save", | ||
"setup": "npm install && cd ../demo && npm install && cd ../src && npm run build && cd ../demo && npm install ../src && cd ..", | ||
"ios": "xcproj --project platforms/ios/YourApp.xcodeproj touch; xcproj --project platforms/ios/Pods/Pods.xcodeproj touch; tns livesync ios --emulator --watch" | ||
@@ -24,3 +25,3 @@ }, | ||
"type": "git", | ||
"url": "https://github.com/TheOriginalJosh/nativescript-slides.git" | ||
"url": "https://github.com/JoshDSommer/nativescript-slides.git" | ||
}, | ||
@@ -64,9 +65,9 @@ "keywords": [ | ||
"bugs": { | ||
"url": "https://github.com/TheOriginalJosh/nativescript-slides/issues" | ||
"url": "https://github.com/JoshDSommer/nativescript-slides/issues" | ||
}, | ||
"license": { | ||
"type": "MIT", | ||
"url": "https://github.com/TheOriginalJosh/nativescript-slides/blob/master/LICENSE" | ||
"url": "https://github.com/JoshDSommer/nativescript-slides/blob/master/LICENSE" | ||
}, | ||
"homepage": "https://github.com/TheOriginalJosh/nativescript-slides", | ||
"homepage": "https://github.com/JoshDSommer/nativescript-slides", | ||
"readmeFilename": "README.md", | ||
@@ -81,2 +82,2 @@ "devDependencies": { | ||
} | ||
} | ||
} |
141
README.md
# NativeScript Slides for iOS and Android | ||
### Supports {N} 2.0+ | {N} 3.0 RC3 + | ||
[](https://www.npmjs.com/package/nativescript-slides) | ||
@@ -11,5 +9,7 @@ [](https://www.npmjs.com/package/nativescript-slides) | ||
### Intro slides example: | ||
[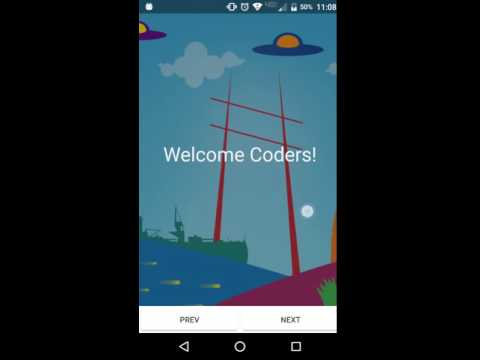](https://www.youtube.com/embed/kGby8qtSDjM) | ||
### Image carousel example: | ||
[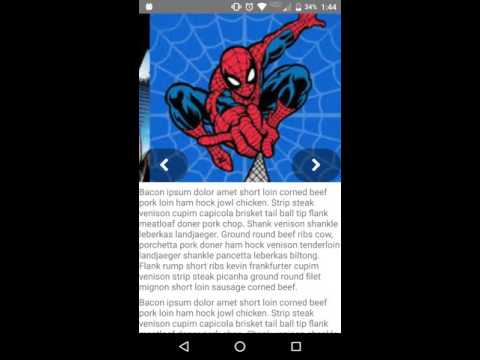](https://www.youtube.com/embed/RsEqGAKm62k) | ||
@@ -20,3 +20,5 @@ | ||
## Example Usage: | ||
### XML | ||
```xml | ||
@@ -43,22 +45,24 @@ | ||
``` | ||
### CSS | ||
```css | ||
.slide-1{ | ||
.slide-1 { | ||
background-color: darkslateblue; | ||
} | ||
.slide-2{ | ||
.slide-2 { | ||
background-color: darkcyan; | ||
} | ||
.slide-3{ | ||
.slide-3 { | ||
background-color: darkgreen; | ||
} | ||
.slide-4{ | ||
.slide-4 { | ||
background-color: darkgoldenrod; | ||
} | ||
.slide-5{ | ||
.slide-5 { | ||
background-color: darkslategray; | ||
} | ||
Label{ | ||
label { | ||
text-align: center; | ||
@@ -69,11 +73,11 @@ width: 100%; | ||
} | ||
``` | ||
``` | ||
Great for Intros/Tutorials to Image Carousels. | ||
To use the intro slide plugin you need to first import it into your xml layout with `xmlns:Slides="nativescript-slides"` | ||
To use the intro slide plugin you need to first import it into your xml layout with `xmlns:Slides="nativescript-slides"` | ||
when using the intro slide plugin you need at least two ``<Slides:Slide>`` views inside of the ``<Slides:SlideContainer>``. | ||
when using the intro slide plugin you need at least two `<Slides:Slide>` views inside of the `<Slides:SlideContainer>`. | ||
add as many ``<Slides:Slide>`` as you want. | ||
add as many `<Slides:Slide>` as you want. | ||
@@ -86,14 +90,13 @@ ### Methods for SlideContainer | ||
### Attributes for SlideContainer | ||
- **loop : boolean** - If true will cause the slide to be an endless loop. The suggested use case would be for a Image Carousel or something of that nature. | ||
- **loop : boolean** - If true will cause the slide to be an endless loop. The suggested use case would be for a Image Carousel or something of that nature. | ||
- **disablePan : boolean** - If true panning is disabled. So that you can call nextSlide()/previousSlide() functions to change the slide. If slides is used to get details about users like email, phone number, username etc. in this case you don't want users to move from one slide to another slide without filling details. | ||
- **pagerOffset : string** - Margin-top for the pager. Number or percentage, default 88%. | ||
- **pagerOffset : string** - Margin-top for the pager. Number or percentage, default 88%. | ||
- **pageIndicators : boolean** - If true adds indicator dots to the bottom of your slides. | ||
- **slideWidth: number - set the width of your slides. (Only currently works on android). | ||
- \*\*slideWidth: number - set the width of your slides. (Only currently works on android). | ||
@@ -105,22 +108,22 @@ #### Indicators | ||
```css | ||
.slide-indicator-inactive{ | ||
background-color: #fff; | ||
opacity : 0.4; | ||
width : 10; | ||
height : 10; | ||
margin-left : 2.5; | ||
margin-right : 2.5; | ||
margin-top : 0; | ||
border-radius : 5; | ||
.slide-indicator-inactive { | ||
background-color: #fff; | ||
opacity: 0.4; | ||
width: 10; | ||
height: 10; | ||
margin-left: 2.5; | ||
margin-right: 2.5; | ||
margin-top: 0; | ||
border-radius: 5; | ||
} | ||
.slide-indicator-active{ | ||
background-color: #fff; | ||
opacity : 0.9; | ||
width : 10; | ||
height : 10; | ||
margin-left : 2.5; | ||
margin-right : 2.5; | ||
margin-top : 0; | ||
border-radius : 5; | ||
.slide-indicator-active { | ||
background-color: #fff; | ||
opacity: 0.9; | ||
width: 10; | ||
height: 10; | ||
margin-left: 2.5; | ||
margin-right: 2.5; | ||
margin-top: 0; | ||
border-radius: 5; | ||
} | ||
@@ -130,2 +133,3 @@ ``` | ||
#### Events | ||
- **start** - Start pan | ||
@@ -137,56 +141,56 @@ - **changed** - Transition complete | ||
#### Angular 2 compatibility | ||
I've started working on a Angular 2 version they can be checked out here: | ||
[Angular 2 version of slides](https://github.com/TheOriginalJosh/nativescript-ng2-slides) | ||
[Angular 2 version of slides](https://github.com/JoshDSommer/nativescript-ng2-slides) | ||
If you want to use this plugin with Angular 2 the `registerElement` from `nativescript-angular` you will want to set the `SlideContainer`'s property of `angular` to `true`. Then in your angular component in the `ngAfterViewInit`. you will want to have an instance of your slide container to call the function `constructView()`. | ||
[Follow the example](https://github.com/TheOriginalJosh/nativescript-slides/issues/37#issuecomment-224820901) | ||
If you want to use this plugin with Angular 2 the `registerElement` from `nativescript-angular` you will want to set the `SlideContainer`'s property of `angular` to `true`. Then in your angular component in the `ngAfterViewInit`. you will want to have an instance of your slide container to call the function `constructView()`. | ||
[Follow the example](https://github.com/JoshDSommer/nativescript-slides/issues/37#issuecomment-224820901) | ||
#### Plugin Development Work Flow: | ||
* Clone repository to your machine. | ||
* Run `npm run setup` to prepare the demo project | ||
* Build with `npm run build` | ||
* Run and deploy to your device or emulator with `npm run demo.android` or `npm run demo.ios` | ||
- Clone repository to your machine. | ||
- Run `npm run setup` to prepare the demo project | ||
- Build with `npm run build` | ||
- Run and deploy to your device or emulator with `npm run demo.android` or `npm run demo.ios` | ||
#### Known issues | ||
* There appears to be a bug with the loop resulting in bad transitions going right to left. | ||
* Currently in Android there is an known issue when a slide component inside of a scroll view. | ||
- There appears to be a bug with the loop resulting in bad transitions going right to left. | ||
- Currently in Android there is an known issue when a slide component inside of a scroll view. | ||
#### How To: Load slides dynamically | ||
You want to hook into the loaded event of the view and then create your view elements. | ||
[Demo Code](https://github.com/TheOriginalJosh/nativescript-slides/blob/master/demo/app/dynamic-page.xml) | ||
``` xml | ||
[Demo Code](https://github.com/JoshDSommer/nativescript-slides/blob/master/demo/app/dynamic-page.xml) | ||
```xml | ||
<Slides:SlideContainer loaded="onSlideContainerLoaded" | ||
``` | ||
``` ts | ||
import * as slides from 'nativescript-slides/nativescript-slides' | ||
```ts | ||
import * as slides from 'nativescript-slides/nativescript-slides'; | ||
export function onSlideContainerLoaded(args) { | ||
let slideContainer = <slides.SlideContainer>args.object; | ||
let slideContainer = <slides.SlideContainer>args.object; | ||
//Construct the slides | ||
slideContainer.addChild(getSlide("Page 1", "slide-1")); | ||
slideContainer.addChild(getSlide("Page 2", "slide-2")); | ||
slideContainer.addChild(getSlide("Page 3", "slide-3")); | ||
slideContainer.addChild(getSlide("Page 4", "slide-4")); | ||
slideContainer.addChild(getSlide("Page 5", "slide-5")); | ||
//Construct the slides | ||
slideContainer.addChild(getSlide('Page 1', 'slide-1')); | ||
slideContainer.addChild(getSlide('Page 2', 'slide-2')); | ||
slideContainer.addChild(getSlide('Page 3', 'slide-3')); | ||
slideContainer.addChild(getSlide('Page 4', 'slide-4')); | ||
slideContainer.addChild(getSlide('Page 5', 'slide-5')); | ||
} | ||
} | ||
function getSlide(labelText: string, className: string) { | ||
let slide = new slides.Slide(); | ||
slide.className = className; | ||
let label = new labelModule.Label(); | ||
label.text = labelText; | ||
slide.addChild(label); | ||
function getSlide(labelText: string, className: string) { | ||
let slide = new slides.Slide(); | ||
slide.className = className; | ||
let label = new labelModule.Label(); | ||
label.text = labelText; | ||
slide.addChild(label) | ||
return slide; | ||
} | ||
return slide; | ||
} | ||
``` | ||
### Thanks to these awesome contributors! | ||
@@ -214,4 +218,5 @@ | ||
## Contributing guidelines | ||
[Contributing guidelines](https://github.com/TheOriginalJosh/nativescript-swiss-army-knife/blob/master/CONTRIBUTING.md) | ||
[Contributing guidelines](https://github.com/JoshDSommer/nativescript-swiss-army-knife/blob/master/CONTRIBUTING.md) | ||
## License | ||
@@ -218,0 +223,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
218
73295
7
1282