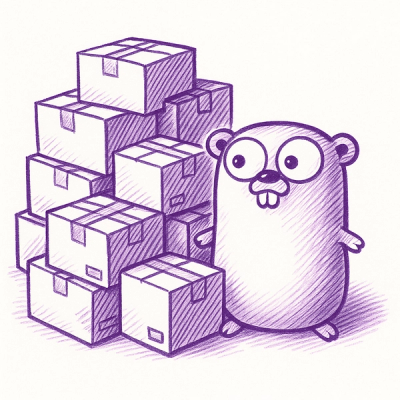
Research
/Security News
11 Malicious Go Packages Distribute Obfuscated Remote Payloads
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
nestjs-formdata-interceptor
Advanced tools
nestjs-formdata-interceptor
is a powerful library for NestJS that provides seamless interception and handling of multipart/form-data requests. This functionality is particularly beneficial for efficiently managing file uploads in your application.
To install nestjs-formdata-interceptor
using npm:
npm install nestjs-formdata-interceptor
yarn add nestjs-formdata-interceptor
To use nestjs-formdata-interceptor
, import it into the main directory of your NestJS application and configure it as shown below:
import { NestFactory } from "@nestjs/core";
import { NestExpressApplication } from "@nestjs/platform-express";
import { AppModule } from "./app.module";
import {
FormdataInterceptor,
LocalFileSaver,
} from "nestjs-formdata-interceptor";
async function bootstrap() {
const app = await NestFactory.create<NestExpressApplication>(AppModule);
app.useGlobalInterceptors(
new FormdataInterceptor({
customFileName(context, originalFileName) {
return `${Date.now()}-${originalFileName}`;
},
fileSaver: new LocalFileSaver({
prefixDirectory: "./public",
customDirectory(context, originalDirectory) {
return originalDirectory;
},
}),
}),
);
await app.listen(3000);
}
bootstrap();
need to install @fastify/multipart
package.
import { NestFactory } from "@nestjs/core";
import { AppModule } from "./app.module";
import {
FastifyAdapter,
NestFastifyApplication,
} from "@nestjs/platform-fastify";
import fastifyMultipart from "@fastify/multipart";
import {
LocalFileSaver,
FormdataInterceptor,
} from "nestjs-formdata-interceptor";
async function bootstrap() {
const app = await NestFactory.create<NestFastifyApplication>(
AppModule,
new FastifyAdapter(),
);
app.register(fastifyMultipart);
app.useGlobalInterceptors(
new FormdataInterceptor({
customFileName(context, originalFileName) {
return `${Date.now()}-${originalFileName}`;
},
fileSaver: new LocalFileSaver({
prefixDirectory: "./public",
customDirectory(context, originalDirectory) {
return originalDirectory;
},
}),
}),
);
await app.listen(3000);
}
bootstrap();
you can use route spesific interceptor
import { Body, Controller, Post, UseInterceptors } from "@nestjs/common";
import { AppService } from "./app.service";
import { CreateDto } from "./dto/create.dto";
import { FormdataInterceptor } from "nestjs-formdata-interceptor";
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Post()
@UseInterceptors(new FormdataInterceptor())
getHello(@Body() createDto: CreateDto) {
// your controller logic
}
}
The customFileName
function allows you to generate custom file names for each uploaded file. In the example above, the file name is prefixed with the current timestamp followed by the original file name.
The LocalFileSaver
is used to define the directory where the files will be saved.
prefixDirectory
specifies the root directory where all files will be saved.customDirectory
allows you to specify a custom sub-directory within the root directory. By default, it uses the original directory provided.If you need custom file-saving logic, implement the IFileSaver interface. Here's an example:
import { FileData, IFileSaver } from "nestjs-formdata-interceptor";
import { ExecutionContext } from "@nestjs/common";
export class CustomFileSaver implements IFileSaver {
public save(
fileData: FileData,
context: ExecutionContext,
args: unknown, // this will be get from save method payload
): any {
// do your file save logic
// and return the file save data
}
}
Then, use your custom file saver in the interceptor configuration:
import { NestFactory } from "@nestjs/core";
import { NestExpressApplication } from "@nestjs/platform-express";
import { AppModule } from "./app.module";
import { FormdataInterceptor } from "nestjs-formdata-interceptor";
import { CustomFileSaver } from "path-to-your-file-saver";
async function bootstrap() {
const app = await NestFactory.create<NestExpressApplication>(AppModule);
app.useGlobalInterceptors(
new FormdataInterceptor({
customFileName(context, originalFileName) {
return `${Date.now()}-${originalFileName}`;
},
fileSaver: new CustomFileSaver(),
}),
);
await app.listen(3000);
}
bootstrap();
If you are using class-validator
describe dto and specify validation rules
import { IsArray, IsNotEmpty } from "class-validator";
import {
FileData,
LocalFileData,
HasMimeType,
IsFileData,
MaxFileSize,
MimeType,
MinFileSize,
} from "nestjs-formdata-interceptor";
export class CreateDto {
@IsArray()
@IsNotEmpty()
@IsFileData({ each: true })
@HasMimeType([MimeType["video/mp4"], "image/png"], { each: true })
@MinFileSize(2000000, { each: true })
@MaxFileSize(4000000, { each: true })
// array file
files: LocalFileData[];
@IsFileData()
@IsNotEmpty()
@HasMimeType([MimeType["video/mp4"], "image/png"])
@MinFileSize(2000000)
@MaxFileSize(4000000)
// single file
file: LocalFileData;
@IsFileData()
@IsNotEmpty()
@HasMimeType([MimeType["video/mp4"], "image/png"])
@MinFileSize(2000000)
@MaxFileSize(4000000)
/**
* customize file data save method
* @param args [string] the payload sent to the custom file saver
* @returns [Promise<string>] the file path where the file was saved
* */
customizeFileData: FileData<Promise<string>, string>;
}
Define your controller to handle file uploads:
import { Body, Controller, Post } from "@nestjs/common";
import { AppService } from "./app.service";
import { CreateDto } from "./dto/create.dto";
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Post()
async getHello(@Body() createDto: CreateDto) {
// save single file
createDto.file.save(); // by default the file save data type is string
// custom the file path on save function
createDto.file.save({ path: "/custom-path" }); // This path will be appended to the prefix directory if it is set.
// save multiple file
createDto.files.map((file) => file.save()); // by default the file save data type is string
// customize file data example
await createDto.customizeFileData.save("bucket_name"); // it will be returning Promise<string>
}
}
With this setup, nestjs-formdata-interceptor
will manage multipart/form-data requests efficiently, allowing for structured handling of file uploads in your NestJS application.
FAQs
nest js formdata interceptor
We found that nestjs-formdata-interceptor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).