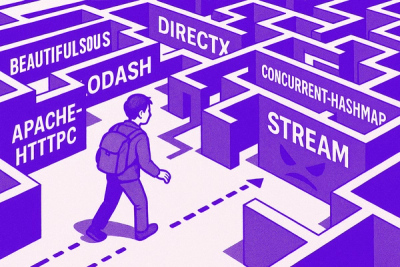
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
nestjs-simple-acl
Advanced tools
A simple easy to use access control level module for NestJS
Nest Simple ACL framework TypeScript starter repository Nest.
$ npm install --save nestjs-simple-acl
The first thing you need to do to implement an AuthorizationStrategy. Here is an example based on user role authorization:
role.model.ts
export class Roles {
name: string;
authorizations: string[];
}
user.model.ts
import { Role } from "./role.model";
export class User {
name: string;
email: string;
password: string;
role: Role;
}
If you are using the JWT authentication approach with Passport.js, this guard should work for you:
role-authorization.strategy.ts
import { Request } from "express";
import { AuthorizationStrategy } from "nestjs-simple-acl";
import { User } from "./user.model";
export class RoleAuthorizationStrategy implements AuthorizationStrategy {
isAuthorized(request: Request, requiredAuthorization: string): boolean {
// User injected on every jwt authenticated request
const user: User = request.user as User;
if (
// Checks if required authorization is present on user's role authorizations
user.role.authorizations.some(
(authorization) => authorization.name === requiredAuthorization
)
) {
// If so, grant access
return true;
}
// If not, deny access
return false;
}
}
Now you import ACLModule on app.module.js.
app.module.ts
import { DynamicModule, Module } from "@nestjs/common";
import { TypeOrmModule } from "@nestjs/typeorm";
import { ACLModule } from "nestjs-simple-acl";
import { RoleAuthorizationStrategy } from "./strategies/role.strategy";
@Module({
imports: [
ACLModule.forRoot({
strategy: new RoleAuthorizationStrategy(),
}),
],
controllers: [],
providers: [],
exports: [],
})
export class AppModule {}
You will need to create ENUM containing your app's or module's authorizations list. Here is an example:
test.authorizations.ts
export enum TestAuthorizations {
SEARCH = "SEARCH",
CREATE = "CREATE",
}
Then you need to register the authorizations on ACLModule.
app.module.ts
import { DynamicModule, Module } from "@nestjs/common";
import { TypeOrmModule } from "@nestjs/typeorm";
import { ACLModule } from "nestjs-simple-acl";
import { RoleAuthorizationStrategy } from "./strategies/role.strategy";
import { TestAuthorizations } from "./test.authorizations";
@Module({
imports: [
ACLModule.forRoot({
strategy: new RoleAuthorizationStrategy(),
}),
ACLModule.registerAuthorizations([TestAuthorizations]),
],
controllers: [],
providers: [],
exports: [],
})
export class AppModule {}
Now you will need to use the AuthorizationGuard on your controller and anotate the functions that should be protected with @RequiredAuthorization() decorator passing the required authorization as parameter.
import { Controller, Get, Post, UseGuards } from "@nestjs/common";
import { AuthorizationGuard, RequiredAuthorization } from "nest-simple-acl";
import { TestAuthorizations } from "./test.authorizations";
@UseGuards(AuthorizationGuard)
@Controller("test")
export class TestController {
@RequiredAuthorization(TestAuthorizations.SEARCH)
@Get()
async search(): Promise<string> {
return "Ok";
}
@RequiredAuthorization(TestAuthorizations.CREATE)
@Post()
async create(): Promise<string> {
return "Ok";
}
}
That's it!
# e2e tests
$ npm run test:e2e
NestJS Simple ACL MIT licensed.
FAQs
NestJS module for Access Control Level support
The npm package nestjs-simple-acl receives a total of 7 weekly downloads. As such, nestjs-simple-acl popularity was classified as not popular.
We found that nestjs-simple-acl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.