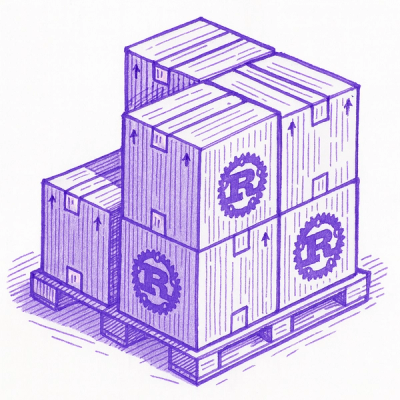
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
nexmo-conversation-js
Advanced tools
npm install nexmo-conversation
insteadnexmo-cli
tool[username]
)$ npm install nexmo-conversation
create a nexmo app using the nexmo-cli
$ nexmo app:create "Application name" --keyfile private.key
get a token to create a user:
$ nexmo jwt:generate private.key application_id=[application_id]
create a user in your app
$ curl -X POST -H 'Authorization: Bearer [JWT]' -H 'Content-Type:application/json' -d '{"name":"[username]"}' https://api.nexmo.com/beta/users
include the script in your web page
<script src="node_modules/nexmo-conversation/dist/conversationClient.js"></script>
get a user's token
$ nexmo jwt:generate private.key application_id=[application_id] sub=[username]
var rtc = new ConversationClient({debug:false});
//var token = request login token as above, with sub=<username>
rtc.login(token).then(
function(application){
//use the application object to manage the conversations
//access the available conversations
console.log(application.conversations);
});
rtc.notifications({
state: "on",
dir: "auto",
lang: "EN",
tag: "notificationPopUp",
icon: "https://int-dir.s3.amazonaws.com/uploads/282_282_social_icon_360x360.png"
});
rtc.notify("Title", "Message");
var conversationData = {name:'My Nexmo Conversation'};
application.newConversation(conversationData).then(
function(conversation) {
//join the created conversation
conversation.join(application.me).then(
function(member) {
console.log("Joined as " + member.name);
}).catch(
function(error) {
console.log(error);
});
}).catch(function(error) {
console.log(error);
});
application.getConversation(conversation_id).then(
function(conv) {
conversation = conv;
});
conversation.on("text", function(sender, textEvent){
if (textEvent.cid === conversation.id){
//if (rtc.isVisible) { textEvent.seen(); }
console.log("my message was:", textEvent, sender);
}else{
console.log("got a message from another member:", textEvent, sender);
}
});
conversation.sendText("Hi Nexmo").then(
function(){
console.log('message was sent');
}).catch(function(error){
console.log('error sending the message', error);
});
conversation.sendImage(fileInput.files[0]);
conversation.on("image", function(sender, imageEvent){
imageEvent.fetchImage().then(function(imagedata) {
message.body.thumbnail = imagedata;
});
}
$ npm install
$ cd node_modules/nexmo-conversation
$ grunt
FAQs
Nexmo Conversation SDK for JavaScript
The npm package nexmo-conversation-js receives a total of 1 weekly downloads. As such, nexmo-conversation-js popularity was classified as not popular.
We found that nexmo-conversation-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.