All In One Downloader
Download Media From Multiple Website Using One Library.
All downloader scrape directly from the sites without 3rd api.
Available Sites
Still Adding New More...

Instagram
|

Facebook
|

Tiktok
|

Twitter
|

Youtube
|

GoogleDrive
|
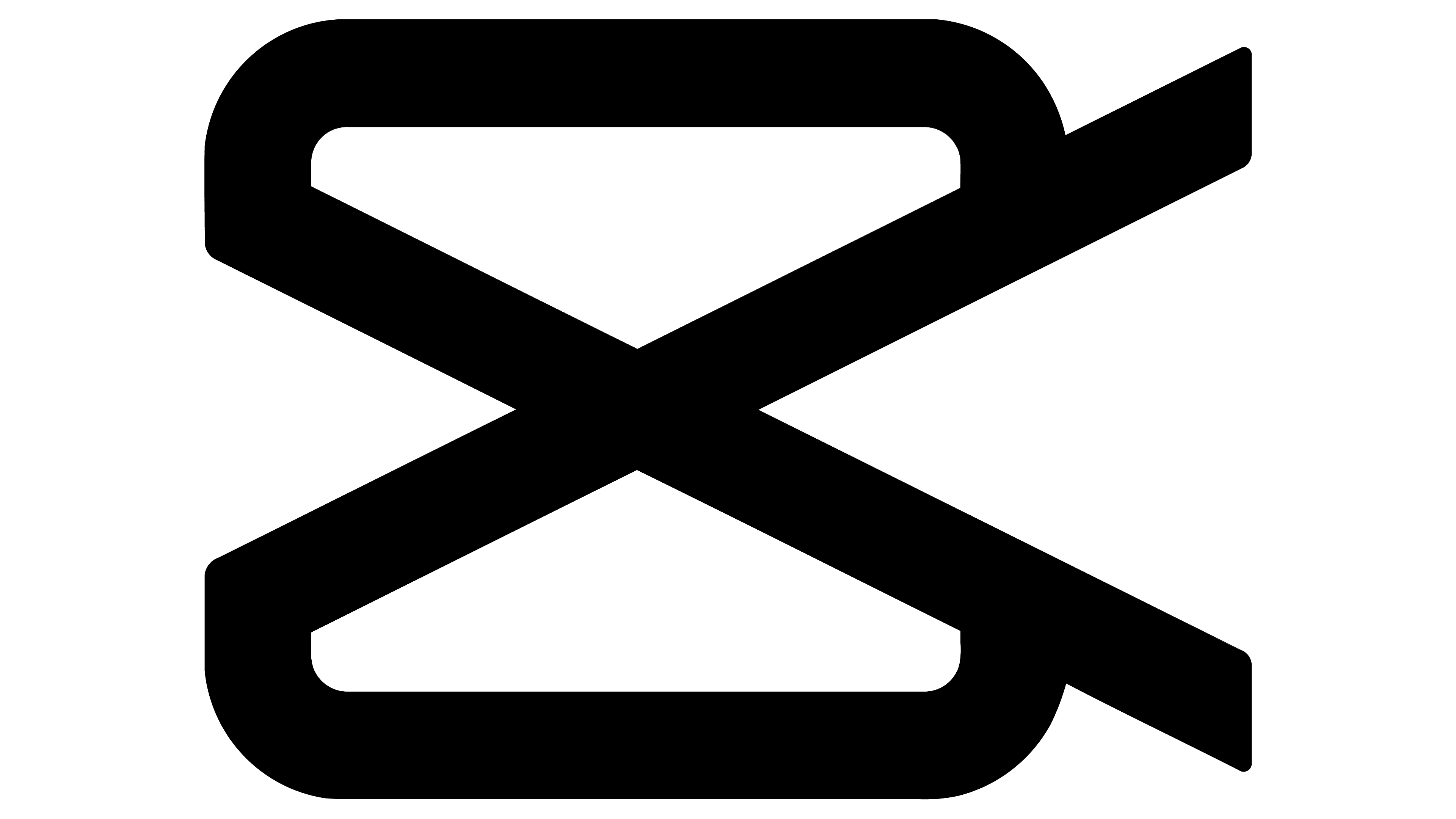
Capcut
|

Pixiv
|

Mega
|
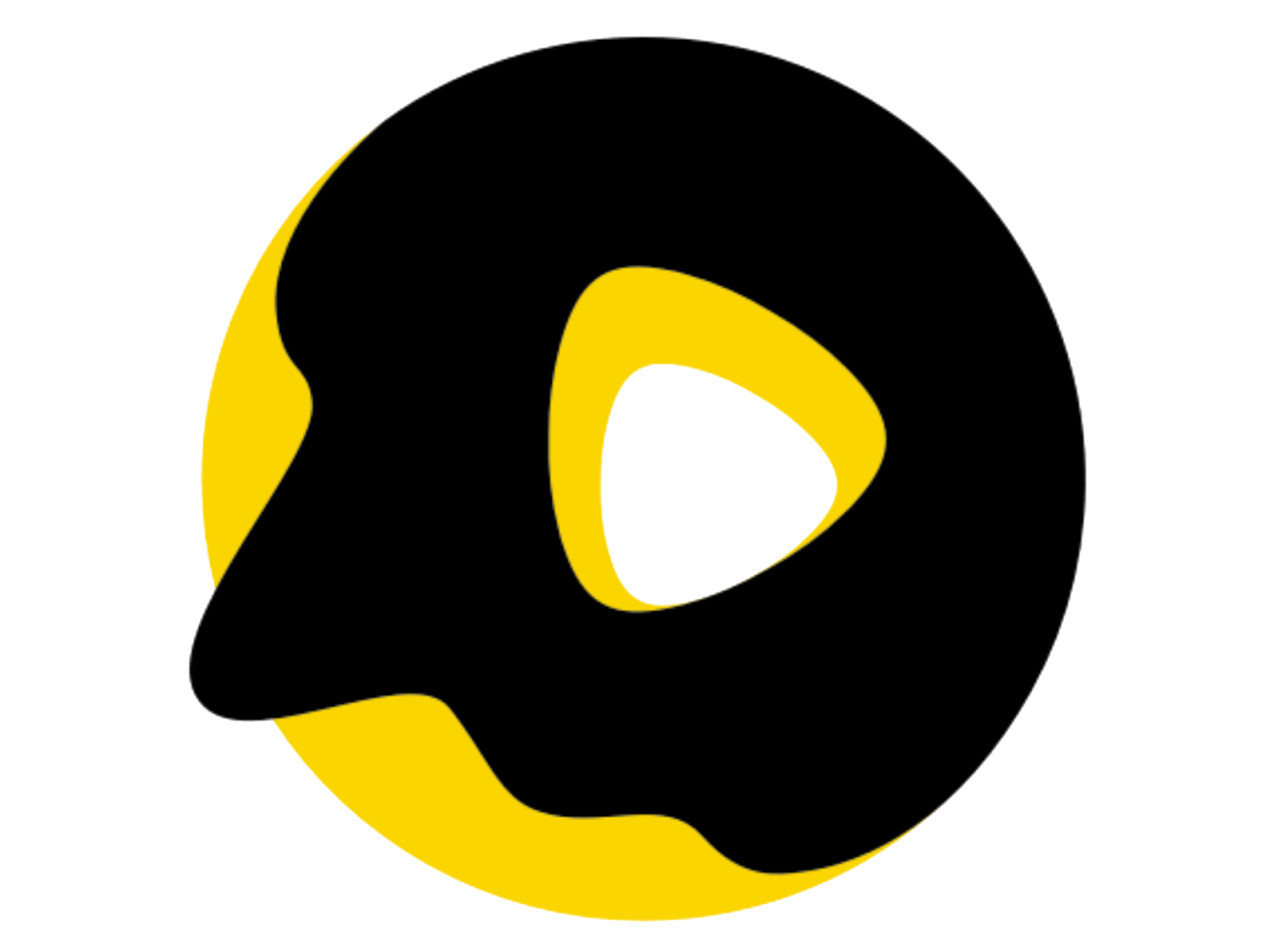
Snack
|

Pinterest
|

Bilibili
|
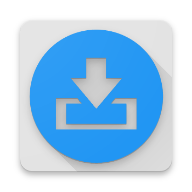
Sfile
|
Required (OPTIONAL)
Youtube requires Python 3.7 or above available in your system as python3. Otherwise, the youtube downloader get disable.
Usage
Installation:
npm i nexo-aio-downloader
Example
For more example will added in future.
const nexo = require("nexo-aio-downloader");
const listUrl = {
twitter: "https://twitter.com/x_cast_x/status/1809598797603041498?t=tXO1JdAR1Avm2BY5wNQX-w&s=19",
instagram: "https://www.instagram.com/reel/C9cFHKIySEu/?igsh=NnhmdmppdHo3dm9o",
facebook: "https://www.facebook.com/share/r/WsMBxDEAWcMVXCf9/?mibextid=D5vuiz",
tiktok: "https://vm.tiktok.com/ZSYnnbXW7",
"google-drive": "https://drive.google.com/file/d/1E8fOgl4nu4onGR756Lw2ZAVv6NgP1H74/view?usp=drive_link",
sfile: "https://sfile.mobi/5g9STNCU525"
}
(async () => {
const result = await nexo.aio('any_url')
console.log(result)
const insta = await nexo.instagram(listUrl.instagram)
console.log(insta)
const fb = await nexo.facebook(listUrl.facebook)
console.log(insta)
})()
Youtube Example
const nexo = require("nexo-aio-downloader");
const youtubeUrl = 'https://www.youtube.com/watch?v=oOIztBXox60'
const playlistUrl = 'https://www.youtube.com/playlist?list=PL8mG-RkN2uTzbbUgvbn2YzBLLU3wktwo0'
const quality = 3
const bitrateList = 9
const dirPath = './youtube'
(async () => {
const youtube = await nexo.youtube(youtubeUrl, quality)
console.log(youtube)
const youtubePlaylist = await nexo.youtubePlaylist(playlistUrl, quality, dirPath)
console.log(youtubePlaylist)
const bitList = await nexo.youtube(youtubeUrl, bitrateList)
const customAudio = await nexo.youtube(youtubeUrl, 160)
console.log(customAudio)
})()
Pixiv Example
For R-18 works require cookie
const nexo = require("nexo-aio-downloader");
const pixivUrl = 'https://www.pixiv.net/en/artworks/120829610'
const userUrl = 'https://www.pixiv.net/en/users/25030629'
const type = 'illust'
const cookie = '55511249_rVrZ0ygXjti1WfuDahh4yCDE4Qo5UUqNK'
(async () => {
const pixiv = await nexo.pixiv(pixivUrl)
console.log(pixiv)
const pixiv18 = await nexo.pixiv(pixivUrl, cookie)
console.log(pixiv18)
const pixivbatch = await nexo.pixivBatch(pixivUrl, cookie, type)
console.log(batch)
})()
How To Get Cookie:
- Login to pixiv using your account
- Open the developer tools (F12, Ctrl+Shift+I, or Cmd+J)
- Go to the
Application
tab
- Go to the
Storage
section and click on Cookies
- Look for the
PHPSESSID
- Open the object, right click on value and copy your session token.

Bilibili Example
const nexo = require("nexo-aio-downloader");
const fs = require("fs")
const biliUrl = 'https://www.bilibili.tv/id/video/2042507617'
const quality = '32';
const cookie = 'SESSDATA=xxxxx'
(async () => {
const result = await bilibiliDownloader(biliUrl, {
download: true,
quality,
cookie,
});
if (result.status) {
console.log('Title:', result.data.title);
console.log('Available quality:');
result.data.mediaList.videoList.forEach(v => {
console.log(`- ${v.quality} (${v.quality_id})`);
});
if (result.data.result) {
fs.writeFileSync(`${result.data.title}.mp4`, result.data.result);
console.log('✅ Video saved succesfully!');
} else {
console.log('📄 Mode metadata-only, no downloading video.');
}
} else {
console.error('❌ Gagal:', result.message);
}
})();
PINTEREST Example
const nexo = require("nexo-aio-downloader");
const pinUrl = 'https://id.pinterest.com/pin/774124930087393/'
(async () => {
const pinDownload = await nexo.pinterest.download(pinUrl)
console.log(pinDownload)
const pinSearch = await nexo.pinterest.search('cat', 10)
console.log(pinSearch)
const pinProfile = await nexo.pinterest.profile('anon')
console.log(pinProfile)
})()
Example Using Proxy
For like instagram that have ip limit
const nexo = require("nexo-aio-downloader");
const { HttpsProxyAgent } = require('https-proxy-agent');
const username = 'example_user'
const password = 'example'
const hostname = 'ex.ample.com'
const port = 6060
const httpsProxyAgent = new HttpsProxyAgent(`http://${username}:${password}@${hostname}:${port}`);
const url = "https://www.instagram.com/reel/C9cFHKIySEu/?igsh=NnhmdmppdHo3dm9o"
(async () => {
const result = await nexo.aio(url, httpsProxyAgent)
console.log(result)
})()
License
nexo-aio-downloader is licensed under the MIT License. Please refer to the LICENSE file for more information.