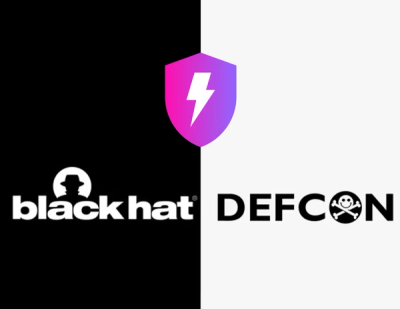
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
next-api-builder
Advanced tools
An easy way to build API routes in Next.js with the right defaults.
An easy way to build API routes in Next.js with the right defaults.
Features:
400: Bad Request
response when a Zod schema is supplied and the input data fails validationOPTIONS
response based on defined HTTP methodsHEAD
response based on your defined GET
handler405: Method Not Found
response for requests to methods not handledInstall with NPM:
npm i next-api-builder
Or with Yarn:
yarn add next-api-builder
import { apiRoute } from 'next-api-builder';
import type { NextApiRequest, NextApiResponse } from 'next/types';
export default apiRoute()
.get(async (req: NextApiRequest, res: NextApiResponse) => {
return { foo: 'bar!' };
})
.post(async (req: NextApiRequest, res: NextApiResponse) => {
// Insert data into some DB...
return req.body || { id: 0, title: 'Sample record' };
});
Next API builder uses Zod for runtime validation of a request body and/or query string parameters.
You can use a second optional object literal parameter when defining routes to supply a Zod schema.
validateBody
- Parse the body as an object (from JSON, etc.) and run validations on itvalidateQuery
- Parse the query string and run validations on itimport { apiRoute } from 'next-api-builder';
import type { NextApiRequest, NextApiResponse } from 'next/types';
const schema = z.object({
name: z.string(),
email: z.string().email(),
});
type TSchema = z.infer<typeof schema>;
export default apiRoute().post(
async (req: NextApiRequest, res: NextApiResponse, data: TSchema) => {
// Insert data into some DB...
return data || { id: 0, title: 'Sample record' };
},
{ validateBody: schema }, // Use 'validateBody' or 'validateQuery' with a Zod schema object
);
If the incoming HTTP Request fails validation, a 400: Bad Request
will be returned to the user with the field level
errors and messages returned from Zod, formatted to JSON.
FAQs
An easy way to build API routes in Next.js with the right defaults.
The npm package next-api-builder receives a total of 0 weekly downloads. As such, next-api-builder popularity was classified as not popular.
We found that next-api-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.