next-redux-saga
Advanced tools
Comparing version 2.0.1 to 3.0.0-beta.1
@@ -36,4 +36,5 @@ import _regeneratorRuntime from 'babel-runtime/regenerator'; | ||
value: function () { | ||
var _ref = _asyncToGenerator(_regeneratorRuntime.mark(function _callee(ctx) { | ||
var isServer, store, props; | ||
var _ref = _asyncToGenerator(_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
@@ -43,4 +44,4 @@ while (1) { | ||
case 0: | ||
isServer = ctx.isServer, store = ctx.store; | ||
props = void 0; | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
@@ -53,6 +54,6 @@ if (!BaseComponent.getInitialProps) { | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(ctx); | ||
return BaseComponent.getInitialProps(props); | ||
case 5: | ||
props = _context.sent; | ||
pageProps = _context.sent; | ||
@@ -65,3 +66,3 @@ case 6: | ||
return _context.abrupt('return', props); | ||
return _context.abrupt('return', pageProps); | ||
@@ -82,3 +83,3 @@ case 8: | ||
return _context.abrupt('return', props); | ||
return _context.abrupt('return', pageProps); | ||
@@ -85,0 +86,0 @@ case 13: |
@@ -41,4 +41,5 @@ (function (global, factory) { | ||
value: function () { | ||
var _ref = _asyncToGenerator(_regeneratorRuntime.mark(function _callee(ctx) { | ||
var isServer, store, props; | ||
var _ref = _asyncToGenerator(_regeneratorRuntime.mark(function _callee(props) { | ||
var _props$ctx, isServer, store, pageProps; | ||
return _regeneratorRuntime.wrap(function _callee$(_context) { | ||
@@ -48,4 +49,4 @@ while (1) { | ||
case 0: | ||
isServer = ctx.isServer, store = ctx.store; | ||
props = void 0; | ||
_props$ctx = props.ctx, isServer = _props$ctx.isServer, store = _props$ctx.store; | ||
pageProps = {}; | ||
@@ -58,6 +59,6 @@ if (!BaseComponent.getInitialProps) { | ||
_context.next = 5; | ||
return BaseComponent.getInitialProps(ctx); | ||
return BaseComponent.getInitialProps(props); | ||
case 5: | ||
props = _context.sent; | ||
pageProps = _context.sent; | ||
@@ -70,3 +71,3 @@ case 6: | ||
return _context.abrupt('return', props); | ||
return _context.abrupt('return', pageProps); | ||
@@ -87,3 +88,3 @@ case 8: | ||
return _context.abrupt('return', props); | ||
return _context.abrupt('return', pageProps); | ||
@@ -90,0 +91,0 @@ case 13: |
{ | ||
"name": "next-redux-saga", | ||
"version": "2.0.1", | ||
"version": "3.0.0-beta.1", | ||
"description": "redux-saga HOC for Next.js", | ||
@@ -75,4 +75,4 @@ "repository": "https://github.com/bmealhouse/next-redux-saga.git", | ||
"lint-staged": "6.1.0", | ||
"next": "4.2.3", | ||
"next-redux-wrapper": "1.3.5", | ||
"next": "6.0.0", | ||
"next-redux-wrapper": "2.0.0-beta.4", | ||
"prettier": "1.10.2", | ||
@@ -79,0 +79,0 @@ "prop-types": "15.6.0", |
@@ -9,2 +9,4 @@ # next-redux-saga | ||
:warning: This will work only with NextJS 6+ :warning: | ||
## Installation | ||
@@ -29,3 +31,3 @@ | ||
`next-redux-saga` uses the redux store created by [next-redux-wrapper](https://github.com/kirill-konshin/next-redux-wrapper). Please refer to their documentation for more information. | ||
`next-redux-saga` uses the redux store created by [next-redux-wrapper](https://github.com/kirill-konshin/next-redux-wrapper). Please refer to their documentation for more information. | ||
@@ -48,9 +50,9 @@ ### Configure Store | ||
) | ||
/** | ||
* next-redux-saga depends on `runSagaTask` and `sagaTask` being attached to the store. | ||
* | ||
* | ||
* `runSagaTask` is used to rerun the rootSaga on the client when in sync mode (default) | ||
* `sagaTask` is used to await the rootSaga task before sending results to the client | ||
* | ||
* | ||
*/ | ||
@@ -70,10 +72,44 @@ | ||
### Wrap Page Component | ||
### Configure Custom App Component | ||
```js | ||
import React, {Component} from 'react' | ||
import React from 'react' | ||
import App, {Container} from 'next/app' | ||
import {Provider} from 'react-redux' | ||
import withRedux from 'next-redux-wrapper' | ||
import withReduxSaga from 'next-redux-saga' | ||
import nextReduxSaga from 'next-redux-saga' | ||
import configureStore from './configure-store' | ||
class ExampleApp extends App { | ||
static async getInitialProps({Component, ctx}) { | ||
let pageProps = {} | ||
if (Component.getInitialProps) { | ||
pageProps = await Component.getInitialProps(ctx) | ||
} | ||
return {pageProps} | ||
} | ||
render() { | ||
const {Component, pageProps, store} = this.props | ||
return ( | ||
<Container> | ||
<Provider store={store}> | ||
<Component {...pageProps} /> | ||
</Provider> | ||
</Container> | ||
) | ||
} | ||
} | ||
export default withRedux(configureStore)(withReduxSaga(ExampleApp)) | ||
``` | ||
### Connect Page Components | ||
```js | ||
import React, {Component} from 'react' | ||
import {connect} from 'react-redux' | ||
class ExamplePage extends Component { | ||
@@ -90,5 +126,3 @@ static async getInitialProps({store}) { | ||
export default withRedux(configureStore, state => state)( | ||
withReduxSaga(ExamplePage) | ||
) | ||
export default connect(state => state)(ExamplePage) | ||
``` | ||
@@ -98,5 +132,5 @@ | ||
To be consistent with how Next.js works, `next-redux-saga` defaults to **sync mode** in version 2.x. When you trigger a route change on the client, your browser **WILL NOT** navigate to the new page until `getInitialProps()` has completed running all it's asynchronous tasks. | ||
To be consistent with how Next.js works, `next-redux-saga` defaults to **sync mode** in version 2.x. When you trigger a route change on the client, your browser **WILL NOT** navigate to the new page until `getInitialProps()` has completed running all it's asynchronous tasks. | ||
For backwards compatibility with 1.x, **async mode** is still supported, however it is no longer the default behavior. When you trigger a route change on the client in async mode, your browser **WILL** navigate to the new page immediately and continue to carry out the asynchronous tasks from `getInitialProps()`. When the asynchronous tasks have completed, React will rerender the components necessary to display the async data. | ||
For backwards compatibility with 1.x, **async mode** is still supported, however it is no longer the default behavior. When you trigger a route change on the client in async mode, your browser **WILL** navigate to the new page immediately and continue to carry out the asynchronous tasks from `getInitialProps()`. When the asynchronous tasks have completed, React will rerender the components necessary to display the async data. | ||
@@ -113,5 +147,5 @@ ```js | ||
[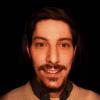](https://github.com/bmealhouse) | [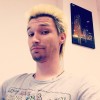](https://github.com/JerryCauser) | ||
---|--- | ||
[Brent Mealhouse](https://github.com/bmealhouse) | [Artem Abzanov](https://github.com/JerryCauser) | ||
| [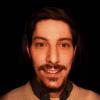](https://github.com/bmealhouse) | [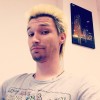](https://github.com/JerryCauser) | [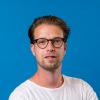](https://github.com/RobbinHabermehl) | | ||
| ----------------------------------------------------------------------------------------------- | ----------------------------------------------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- | | ||
| [Brent Mealhouse](https://github.com/bmealhouse) | [Artem Abzanov](https://github.com/JerryCauser) | [Robbin Habermehl](https://github.com/RobbinHabermehl) | | ||
@@ -118,0 +152,0 @@ ## Contributing |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
24522
156
1