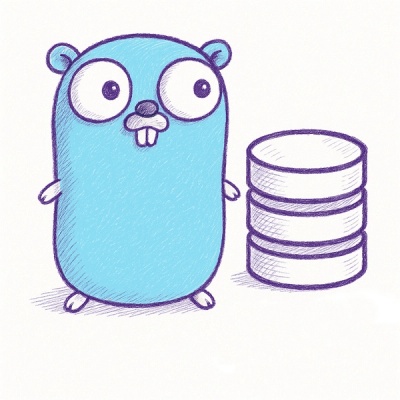
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
next-webworker-pool
Advanced tools
A NPM package that enables developers to build Web Worker pools that can be used in (but not limited to) Next.js applications
A no-dependency package that enables developers to build Web Worker pools for Next.js applications.
You can find an example Next.js project using next-webworker-pool
here.
npm install next-webworker-pool
This is the file that will be run inside the Web Worker.
// my-worker.ts
import type { WebWorkerTask, WebWorkerResult } from 'next-webworker-pool';
type MyInput = number; // can be anything that the client will send
type MyOutput = number; // can be anything that the client will receive
self.onmessage = function (e: MessageEvent<WebWorkerTask<MyInput, MyOutput>>) {
self.postMessage(runTask(e.data));
};
function runTask(
task: WebWorkerTask<MyInput, MyOutput>,
): WebWorkerResult<MyInput> {
const result = task.data + 1; // do something with the input
return {
id: task.id,
data: result,
};
}
WebWorkerPool
class or use the factory functionThis is a class that is responsible for creating Web Workers from a specific source, and running tasks on them.
This pattern is used, because Next.js scans the source code for new Worker(new URL(...))
calls, and replaces
them with the Next.js custom bundling implementation.
// my-worker-pool.ts
import { createWebWorkerPool } from 'next-webworker-pool';
import type { MyInput, MyOutput } from './my-worker';
export const myWorkerPool = createWebWorkerPool<MyInput, MyOutput>(
new URL('./my-worker.ts', import.meta.url),
{ maxWorkers: 4 },
);
The worker pool can then be used directly in your Next.js application:
// pages/index.tsx
import { myWorkerPool } from '../my-worker-pool';
export default function Home() {
const [result, setResult] = useState<number | null>(null);
useEffect(() => {
const task = myWorkerPool.executeTask(1); // run the task with input 1
// wait for the task to finish and use the result
task.promise
.then((result) => {
setResult(result);
})
.catch((error) => {
console.error(error);
});
return () => {
// terminate the Web Worker pool when the component is unmounted
myWorkerPool.terminate();
};
}, []);
return <div>{result}</div>;
}
WebWorkerPool
class// my-worker-pool.ts
import { WebWorkerPool } from 'next-webworker-pool';
import type { MyInput, MyOutput } from './my-worker';
export class MyWorkerPool extends WebWorkerPool<MyInput, MyOutput> {
_createWorker(): Worker {
return new Worker(new URL('./my-worker.ts', import.meta.url));
}
}
To use the Web Worker pool, you need to create an instance of it, and call the run
method with the input data.
// pages/index.tsx
import { MyWorkerPool } from '../my-worker-pool';
export default function Home() {
const [result, setResult] = useState<number | null>(null);
useEffect(() => {
// create a new instance of the Web Worker pool
const pool = new MyWorkerPool();
const task = pool.executeTask(1); // run the task with input 1
// wait for the task to finish and use the result
task.promise
.then((result) => {
setResult(result);
})
.catch((error) => {
console.error(error);
});
return () => {
// terminate the Web Worker pool when the component is unmounted
pool.terminate();
};
}, []);
return <div>{result}</div>;
}
maxWorkers
The maximum number of Web Workers that can be created by the pool. Defaults to navigator.hardwareConcurrency
or 4 if hardwareConcurrency
is not supported.
// my-worker-pool.ts
export class MyWorkerPool extends WebWorkerPool<MyInput, MyOutput> {
constructor() {
super({
maxWorkers: 4,
});
}
createWorker(): Worker {
return new Worker(new URL('./my-worker.ts', import.meta.url));
}
}
FAQs
A NPM package that enables developers to build Web Worker pools that can be used in (but not limited to) Next.js applications
The npm package next-webworker-pool receives a total of 72 weekly downloads. As such, next-webworker-pool popularity was classified as not popular.
We found that next-webworker-pool demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.