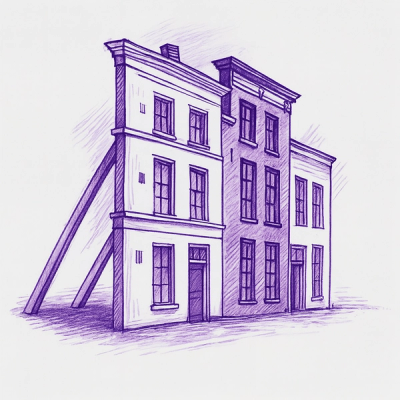
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
ngx-file-drop
Advanced tools
Angular ngx-file-drop - Simple desktop file and folder drag and drop
An Angular module for simple desktop file and folder drag and drop. This library does not need rxjs-compat.
For previous Angular support please use older versions.
This library relies on HTML 5 File API thus IE is not supported
You can check the DEMO of the library
npm install ngx-file-drop --save
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
import { NgxFileDropModule } from 'ngx-file-drop';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule,
NgxFileDropModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
import { Component } from '@angular/core';
import { NgxFileDropEntry, FileSystemFileEntry, FileSystemDirectoryEntry } from 'ngx-file-drop';
@Component({
selector: 'demo-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
public files: NgxFileDropEntry[] = [];
public dropped(files: NgxFileDropEntry[]) {
this.files = files;
for (const droppedFile of files) {
// Is it a file?
if (droppedFile.fileEntry.isFile) {
const fileEntry = droppedFile.fileEntry as FileSystemFileEntry;
fileEntry.file((file: File) => {
// Here you can access the real file
console.log(droppedFile.relativePath, file);
/**
// You could upload it like this:
const formData = new FormData()
formData.append('logo', file, relativePath)
// Headers
const headers = new HttpHeaders({
'security-token': 'mytoken'
})
this.http.post('https://mybackend.com/api/upload/sanitize-and-save-logo', formData, { headers: headers, responseType: 'blob' })
.subscribe(data => {
// Sanitized logo returned from backend
})
**/
});
} else {
// It was a directory (empty directories are added, otherwise only files)
const fileEntry = droppedFile.fileEntry as FileSystemDirectoryEntry;
console.log(droppedFile.relativePath, fileEntry);
}
}
}
public fileOver(event){
console.log(event);
}
public fileLeave(event){
console.log(event);
}
}
<div class="center">
<ngx-file-drop dropZoneLabel="Drop files here" (onFileDrop)="dropped($event)"
(onFileOver)="fileOver($event)" (onFileLeave)="fileLeave($event)">
<ng-template ngx-file-drop-content-tmp let-openFileSelector="openFileSelector">
Optional custom content that replaces the the entire default content.
<button type="button" (click)="openFileSelector()">Browse Files</button>
</ng-template>
</ngx-file-drop>
<div class="upload-table">
<table class="table">
<thead>
<tr>
<th>Name</th>
</tr>
</thead>
<tbody class="upload-name-style">
<tr *ngFor="let item of files; let i=index">
<td><strong>{{ item.relativePath }}</strong></td>
</tr>
</tbody>
</table>
</div>
</div>
Name | Description | Example |
---|---|---|
(onFileDrop) | On drop function called after the files are read | (onFileDrop)="dropped($event)" |
(onFileOver) | On drop over function | (onFileOver)="fileOver($event)" |
(onFileLeave) | On drop leave function | (onFileLeave)="fileLeave($event)" |
accept | String of accepted formats | accept=".png" |
directory | Whether directories are accepted | directory="true" |
dropZoneLabel | Text to be displayed inside the drop box | dropZoneLabel="Drop files here" |
dropZoneClassName | Custom style class name(s) to be used on the "drop-zone" area | dropZoneClassName="my-style" |
contentClassName | Custom style class name(s) to be used for the content area | contentClassName="my-style" |
[disabled] | Conditionally disable the dropzone | [disabled]="condition" |
[showBrowseBtn] | Whether browse file button should be shown | [showBrowseBtn]="true" |
browseBtnClassName | Custom style class name(s) to be used for the button | browseBtnClassName="my-style" |
browseBtnLabel | The label of the browse file button | browseBtnLabel="Browse files" |
multiple | Whether multiple or single files are accepted | multiple="true" |
useDragEnter | Use dragenter event instead of dragover | useDragEnter="true" |
16.0.0 (2023-06-19)
FAQs
Angular ngx-file-drop - Simple desktop file and folder drag and drop
The npm package ngx-file-drop receives a total of 51,627 weekly downloads. As such, ngx-file-drop popularity was classified as popular.
We found that ngx-file-drop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.