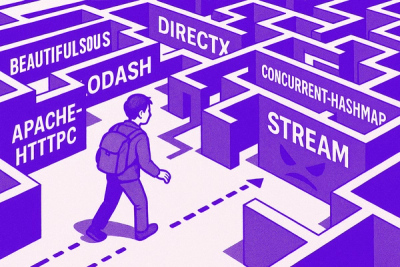
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
ngx-plaid-link
Advanced tools
A wrapper component to make using Plaid Link easy in Angular 6+.
This has been tested to work in at least 1 Angular 5 app as well
$ npm install ngx-plaid-link
$ yarn add ngx-plaid-link
import { BrowserModule } from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { AppComponent } from "./app.component";
import { NgxPlaidLinkModule } from "ngx-plaid-link";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, NgxPlaidLinkModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
ngxPlaidLink
Directives<button ngxPlaidLink
env="sandbox"
publicKey="YOURPUBLICKEY"
institution=""
[countryCodes]="['US', 'CA', 'GB']"
(Success)="onPlaidSuccess($event)"
(Exit)="onPlaidExit($event)"
(Load)="onPlaidLoad($event)"
(Event)="onPlaidEvent($event)"
(Click)="onPlaidClick($event)"
>Link Your Bank Account</button>
<mr-ngx-plaid-link-button
env="sandbox"
publicKey="YOURPUBLICKEY"
institution=""
[countryCodes]="['US', 'CA', 'GB']"
(Success)="onPlaidSuccess($event)"
(Exit)="onPlaidExit($event)"
(Load)="onPlaidLoad($event)"
(Event)="onPlaidEvent($event)"
className="launch-plaid-link-button"
buttonText="Link Your Bank Account"
(Click)="onPlaidClick($event)"
></mr-ngx-plaid-link-button>
Since most of the functionality is through the service you can imlpement this yourself to customize to your needs further.
import { Component, AfterViewInit } from "@angular/core";
import {
PlaidErrorMetadata,
PlaidErrorObject,
PlaidEventMetadata,
PlaidOnEventArgs,
PlaidOnExitArgs,
PlaidOnSuccessArgs,
PlaidSuccessMetadata,
PlaidConfig,
NgxPlaidLinkService,
PlaidLinkHandler
} from "ngx-plaid-link";
export class ComponentThatImplementsPlaidLink implements AfterViewInit {
private plaidLinkHandler: PlaidLinkHandler;
private config: PlaidConfig = {
apiVersion: "v2",
env: "sandbox",
institution: null,
selectAccount: false,
token: null,
webhook: "",
product: ["auth"],
countryCodes: ['US', 'CA', 'GB']
key: "YOURPUBLICKEY"
};
constructor(private plaidLinkService: NgxPlaidLinkService) {}
// Create and open programatically once the library is loaded.
ngAfterViewInit() {
this.plaidLinkService
.createPlaid(
Object.assign({}, config, {
onSuccess: (token, metadata) => this.onSuccess(token, metadata),
onExit: (error, metadata) => this.onExit(error, metadata),
onEvent: (eventName, metadata) => this.onEvent(eventName, metadata)
})
)
.then((handler: PlaidLinkHandler) => {
this.plaidLinkHandler = handler;
this.open();
});
}
open() {
this.plaidLinkHandler.open();
}
exit() {
this.plaidLinkHandler.exit();
}
onSuccess(token, metadata) {
console.log("We got a token:", token);
console.log("We got metadata:", metadata);
}
onEvent(eventName, metadata) {
console.log("We got an event:", eventName);
console.log("We got metadata:", metadata);
}
onExit(error, metadata) {
console.log("We exited:", error);
console.log("We got metadata:", metadata);
}
}
The following chart can be used to determine what version of ngx-plaid-link
should be used within your angular project.
angular@12
support within the chart; this is because there has never been a version of ngx-plaid-link
published that officially supports angular@12
. ngx-plaid-link@1.0.3
should work fine with angular@12
(albeit with peer dependency warnings during installation) but official advice is to upgrade your project to angular@13
or later.angular version | ngx-plaid-link version |
---|---|
6 | 1.0.3 |
7 | 1.0.3 |
8 | 1.0.3 |
9 | 1.0.3 |
10 | 1.0.3 |
11 | 1.0.3 |
13 | 13.0.0 |
This is all there in the types, but here they are for convenience.
Attribute/prop | input/output | optional/required | Type | Default | Description |
---|---|---|---|---|---|
apiVersion | input | optional | string | v2 | The version of the Plaid Link api to use |
buttonText | input | optional | string | Log In To Your Bank Account | You can customize the text on the button by providing text here. |
className | input | optional | string | null | A class or classes to apply to the button inside the component |
clientName | input | required | string | null | The name of your application, gets used in the Plaid Link UI. |
countryCodes | input | optional | string[] | ['US'] | An array of strings of Plaid supported country codes |
env | input | optional | string | sandbox | Can be one of available plaid environments: sandbox , development , or production |
institution | input | optional | string | null | If you want to launch a specific institution |
product | input | optional | string[] | ['auth'] | An array of the names of the products you'd like to authorize. Available options are transactions , auth , and identity . |
publicKey | input | conditional | string | null | The key (publiKey) parameter in PlaidLink create is deprecated. The public key from your Plaid account Make sure it's the public key and not the private key |
token | input | conditional | string | null | If you're using link_tokens this field is required and will be the link_token. If you're still using the public key (legacy), then this field is optional and is for if you are re-authenticating or updating an item that has previously been linked. |
style | input | optional | object | An object of styles | An ngStyle object that can be used to apply styles and customize the plaid link button to match your app. |
selectAccount | input | optional | boolean | false | Setting this to TRUE will allow the user to select their bank account from a list through the plaid modal. FALSE does not show the account list prompt. |
webhook | input | optional | string | null | You can provide a webhook for each item that Plaid will send events to. |
receivedRedirectUri | input | optional | string | null | A receivedRedirectUri is required to support OAuth authentication flows when re-launching Link on a mobile device and using one or more European country codes. In addition to configuring the URI here, you will also need to enable the URI on the developer dashboard. |
isWebview | input | optional | boolean | false | Set to true if launching Link within a WebView. |
Exit | output | required | function | n/a | Passes the result from the onExit function to your component |
Success | output | required | function | n/a | Passes the result from the onSuccess function to your component |
Click | output | optional | function | n/a | Lets you act on the event when the button is clicked |
Event | output | optional | function | n/a | Passes the result from the onEvent function to your component |
Load | output | optional | function | n/a | Lets you act on the event when the Plaid Link stuff is all loaded |
Coming soon...
14.0.0
I tried to make as few breaking changes as possible, but there is one scenario where you could need to update your code.
FAQs
Angular Plaid Link Directive & Button Component
The npm package ngx-plaid-link receives a total of 4,907 weekly downloads. As such, ngx-plaid-link popularity was classified as popular.
We found that ngx-plaid-link demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.