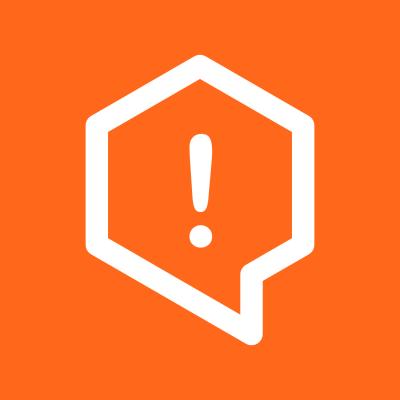
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
ngx-scanner-qrcode
Advanced tools
This library is built to provide a solution scanner QR code. This library takes in raw images and will locate, extract and parse any QR code found within.
This library is built to provide a solution scanner QR code.
This library takes in raw images and will locate, extract and parse any QR code found within.
This demo Github, Stackblitz.
Supported Barcode Types | |||
---|---|---|---|
QR Code | Code-39 | Code-93 | Code-128 |
Codabar | Databar/Expanded | EAN/GTIN-5/8/13 | ISBN-10/13 |
ISBN-13+2 | ISBN-13+5 | ITF (Interleaved 2 of 5) | UPC-A/E. |
Install ngx-scanner-qrcode
from npm
:
npm install ngx-scanner-qrcode@<version> --save
Add wanted package to NgModule imports:
import { NgxScannerQrcodeModule, LOAD_WASM } from 'ngx-scanner-qrcode';
// Necessary to solve the problem of losing internet connection
LOAD_WASM('assets/wasm/ngx-scanner-qrcode.wasm').subscribe();
@NgModule({
imports: [
NgxScannerQrcodeModule
]
})
angular.json
{
"architect": {
"build": {
"options": {
"assets": [
/* Necessary to solve the problem of losing internet connection */
{
"glob": "**/*",
"input": "node_modules/ngx-scanner-qrcode/wasm/",
"output": "./assets/wasm/"
}
]
}
}
}
}
In the Component:
<!-- For camera -->
<ngx-scanner-qrcode #action="scanner"></ngx-scanner-qrcode>
<!-- data -->
<span>{{ action.data.value | json }}</span><!-- value -->
<span>{{ action.data | async | json }}</span><!-- async -->
<!-- Loading -->
<p *ngIf="action.isLoading">⌛ Loading...</p>
<!-- start -->
<button (click)="action.isStart ? action.stop() : action.start()">{{action.isStart ? 'Stop' : 'Start'}}</button>
<!-- For image src -->
<ngx-scanner-qrcode #action="scanner" [src]="'https://domain.com/test.png'"></ngx-scanner-qrcode>
<span>{{ action.data.value | json }}</span><!-- value -->
<span>{{ action.data | async | json }}</span><!-- async -->
<!-- For select files -->
<input #file type="file" (change)="onSelects(file.files)" [multiple]="'multiple'" [accept]="'.jpg, .png, .gif, .jpeg'"/>
<div *ngFor="let item of qrCodeResult">
<ngx-scanner-qrcode #actionFile="scanner" [src]="item.url" [config]="config"></ngx-scanner-qrcode>
<p>{{ actionFile.data.value | json }}</p><!-- value -->
<p>{{ actionFile.data | async | json }}</p><!-- async -->
</div>
import { Component } from '@angular/core';
import { NgxScannerQrcodeService, ScannerQRCodeSelectedFiles } from 'ngx-scanner-qrcode';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public qrCodeResult: ScannerQRCodeSelectedFiles[] = [];
public config: ScannerQRCodeConfig = {
constraints: {
video: {
width: window.innerWidth
}
}
};
constructor(private qrcode: NgxScannerQrcodeService) { }
public onSelects(files: any) {
this.qrcode.loadFiles(files).subscribe((res: ScannerQRCodeSelectedFiles[]) => {
this.qrCodeResult = res;
});
}
}
<!-- For select files -->
<input #file type="file" (change)="onSelects(file.files)" [multiple]="'multiple'" [accept]="'.jpg, .png, .gif, .jpeg'"/>
<div *ngFor="let item of qrCodeResult">
<img [src]="item.url | safe: 'url'" [alt]="item.name" style="max-width: 100%"><!-- Need bypassSecurityTrustUrl -->
<p>{{ item.data | json }}</p>
</div>
import { Component } from '@angular/core';
import { NgxScannerQrcodeService, ScannerQRCodeSelectedFiles } from 'ngx-scanner-qrcode';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public qrCodeResult: ScannerQRCodeSelectedFiles[] = [];
public config: ScannerQRCodeConfig = {
constraints: {
video: {
width: window.innerWidth
}
}
};
constructor(private qrcode: NgxScannerQrcodeService) { }
public onSelects(files: any) {
this.qrcode.loadFilesToScan(files).subscribe((res: ScannerQRCodeSelectedFiles[]) => {
this.qrCodeResult = res;
});
}
}
Field | Description | Type | Default |
---|---|---|---|
[src] | image url | string | - |
[fps] | fps/ms | number | 30 |
[vibrate] | vibrate for mobile | number | 300 |
[decode] | decode value | string | utf-8 |
[isBeep] | beep | boolean | true |
[isMasked] | masked | boolean | true |
[unScan] | scan | boolean | false |
[loadWasmUrl] | wasm local url | string | blank |
[symbolType] | type | ScannerQRCodeSymbolType[] | [ScannerQRCode_NONE] |
[constraints] | setting video | MediaStreamConstraints | {audio:false,video:true} |
[canvasStyles] | setting canvas | CanvasRenderingContext2D[] | [{ lineWidth: 1, strokeStyle: 'green', fillStyle: '#55f02880' },{ font: '15px serif', strokeStyle: '#fff0', fillStyle: '#ff0000' }] |
[config] | config | ScannerQRCodeConfig | {src:..,fps..,vibrate..,decode:..,isBeep:..,config:..,constraints:..,canvasStyles:..} |
Field | Description | Type | Default |
---|---|---|---|
(event) | data response | BehaviorSubject<ScannerQRCodeResult[]> | [] |
Field | Description | Type | Default |
---|---|---|---|
isStart | status | boolean | false |
isLoading | status | boolean | false |
isTorch | torch | boolean | false |
isPause | status | boolean | - |
isReady | status wasm | AsyncSubject | - |
data | data response | BehaviorSubject<ScannerQRCodeResult[]> | [] |
devices | data devices | BehaviorSubject<ScannerQRCodeDevice[]> | [] |
deviceIndexActive | device index | number | 0 |
--- | --- | --- | --- |
(start) | start camera | AsyncSubject | - |
(stop) | stop camera | AsyncSubject | - |
(play) | play video | AsyncSubject | - |
(pause) | pause video | AsyncSubject | - |
(torcher) | toggle on/off flashlight | AsyncSubject | - |
(applyConstraints) | set media constraints | AsyncSubject | - |
(getConstraints) | get media constraints | AsyncSubject | - |
(playDevice) | play deviceId | AsyncSubject | - |
(loadImage) | load image from src | AsyncSubject | - |
(download) | download image | AsyncSubject<ScannerQRCodeSelectedFiles[]> | - |
Field | Description | Type | Default |
---|---|---|---|
(loadFiles) | Convert files | AsyncSubject<ScannerQRCodeSelectedFiles[]> | [] |
(loadFilesToScan) | Scanner files | AsyncSubject<ScannerQRCodeSelectedFiles[]> | [] |
export interface ScannerQRCodeConfig {
src?: string;
fps?: number;
vibrate?: number; /* support mobile */
decode?: string;
unScan?: boolean;
isBeep?: boolean;
isMasked?: boolean;
loadWasmUrl?: string; /* eg. assets/wasm/ngx-scanner-qrcode.wasm */
symbolType?: ScannerQRCodeSymbolType[];
constraints?: MediaStreamConstraints;
canvasStyles?: CanvasRenderingContext2D[] | any[];
}
interface ScannerQRCodeDevice {
kind: string;
label: string;
groupId: string;
deviceId: string;
}
class ScannerQRCodeResult {
type: ScannerQRCodeSymbolType;
typeName: string;
data: Int8Array;
points: Array<ScannerQRCodePoint>;
orientation: ScannerQRCodeOrientation;
time: number;
cacheCount: number;
quality: number;
value: string;
}
enum ScannerQRCodeSymbolType {
ScannerQRCode_NONE = 0, /**< no symbol decoded */
ScannerQRCode_PARTIAL = 1, /**< intermediate status */
ScannerQRCode_EAN2 = 2, /**< GS1 2-digit add-on */
ScannerQRCode_EAN5 = 5, /**< GS1 5-digit add-on */
ScannerQRCode_EAN8 = 8, /**< EAN-8 */
ScannerQRCode_UPCE = 9, /**< UPC-E */
ScannerQRCode_ISBN10 = 10, /**< ISBN-10 (from EAN-13). @since 0.4 */
ScannerQRCode_UPCA = 12, /**< UPC-A */
ScannerQRCode_EAN13 = 13, /**< EAN-13 */
ScannerQRCode_ISBN13 = 14, /**< ISBN-13 (from EAN-13). @since 0.4 */
ScannerQRCode_COMPOSITE = 15, /**< EAN/UPC composite */
ScannerQRCode_I25 = 25, /**< Interleaved 2 of 5. @since 0.4 */
ScannerQRCode_DATABAR = 34, /**< GS1 DataBar (RSS). @since 0.11 */
ScannerQRCode_DATABAR_EXP = 35, /**< GS1 DataBar Expanded. @since 0.11 */
ScannerQRCode_CODABAR = 38, /**< Codabar. @since 0.11 */
ScannerQRCode_CODE39 = 39, /**< Code 39. @since 0.4 */
ScannerQRCode_PDF417 = 57, /**< PDF417. @since 0.6 */
ScannerQRCode_QRCODE = 64, /**< QR Code. @since 0.10 */
ScannerQRCode_SQCODE = 80, /**< SQ Code. @since 0.20.1 */
ScannerQRCode_CODE93 = 93, /**< Code 93. @since 0.11 */
ScannerQRCode_CODE128 = 128, /**< Code 128 */
/*
* Please see _ScannerQRCode_get_symbol_hash() if adding
* anything after 128
*/
/** mask for base symbol type.
* @deprecated in 0.11, remove this from existing code
*/
ScannerQRCode_SYMBOL = 0x00ff,
/** 2-digit add-on flag.
* @deprecated in 0.11, a ::ScannerQRCode_EAN2 component is used for
* 2-digit GS1 add-ons
*/
ScannerQRCode_ADDON2 = 0x0200,
/** 5-digit add-on flag.
* @deprecated in 0.11, a ::ScannerQRCode_EAN5 component is used for
* 5-digit GS1 add-ons
*/
ScannerQRCode_ADDON5 = 0x0500,
/** add-on flag mask.
* @deprecated in 0.11, GS1 add-ons are represented using composite
* symbols of type ::ScannerQRCode_COMPOSITE; add-on components use ::ScannerQRCode_EAN2
* or ::ScannerQRCode_EAN5
*/
ScannerQRCode_ADDON = 0x0700,
}
interface ScannerQRCodePoint {
x: number;
y: number;
}
enum ScannerQRCodeOrientation {
ScannerQRCode_ORIENT_UNKNOWN = -1, /**< unable to determine orientation */
ScannerQRCode_ORIENT_UP, /**< upright, read left to right */
ScannerQRCode_ORIENT_RIGHT, /**< sideways, read top to bottom */
ScannerQRCode_ORIENT_DOWN, /**< upside-down, read right to left */
ScannerQRCode_ORIENT_LEFT, /**< sideways, read bottom to top */
}
interface ScannerQRCodeSelectedFiles {
url: string;
name: string;
file: File;
data?: ScannerQRCodeResult[];
canvas?: HTMLCanvasElement;
}
Support versions | |
---|---|
Angular 16+ | 1.7.3 |
Angular 6+ | 1.7.2 |
Author Information | |
---|---|
Author | DaiDH |
Phone | +84845882882 |
Country | Vietnam |
Bitcoin | Paypal | MbBank |
---|---|---|
![]() | ![]() | ![]() |
LGPL-2.1+ License. Copyright (C) 1991, 1999 Free Software Foundation, Inc.
FAQs
This library is built to provide a solution scanner QR code. This library takes in raw images and will locate, extract and parse any QR code found within.
The npm package ngx-scanner-qrcode receives a total of 5,054 weekly downloads. As such, ngx-scanner-qrcode popularity was classified as popular.
We found that ngx-scanner-qrcode demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.