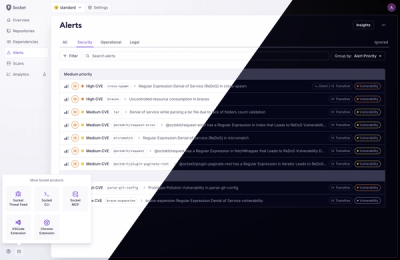
Product
A Fresh Look for the Socket Dashboard
We’ve redesigned the Socket dashboard with simpler navigation, less visual clutter, and a cleaner UI that highlights what really matters.
node-easyocr
Advanced tools
A Node.js wrapper for the Python EasyOCR library
node-easyocr is a Node.js wrapper for the popular Python EasyOCR library, allowing you to perform Optical Character Recognition (OCR) in your Node.js applications. This package provides a simple interface to use EasyOCR's functionality within your JavaScript/TypeScript projects.
npm install node-easyocr
Note: This package requires Python 3.6+ and pip to be installed on your system. The necessary Python dependencies will be installed automatically during the npm installation process.
node-easyocr supports both ES6 and CommonJS module systems. Here are examples for both:
import { EasyOCR } from 'node-easyocr';
const ocr = new EasyOCR();
async function main() {
try {
// Initialize the OCR reader with desired languages
await ocr.init(['en', 'fr']);
console.log('OCR initialized successfully');
const imagePaths = ['path/to/image1.png', 'path/to/image2.png'];
for (const imagePath of imagePaths) {
console.log(`Processing image: ${imagePath}`);
console.time('OCR Process');
const result = await ocr.readText(imagePath);
console.timeEnd('OCR Process');
console.log('OCR Result:');
result.forEach((item, index) => {
console.log(`Line ${index + 1}:`);
console.log(` Text: ${item.text}`);
console.log(` Confidence: ${(item.confidence * 100).toFixed(2)}%`);
console.log(` Bounding Box: ${JSON.stringify(item.bbox)}`);
console.log('---');
});
}
} catch (error) {
console.error('OCR Error:', error.message);
} finally {
await ocr.close();
}
}
main();
const { EasyOCR } = require('node-easyocr');
const ocr = new EasyOCR();
async function main() {
try {
// Initialize the OCR reader with desired languages
await ocr.init(['en', 'fr']);
console.log('OCR initialized successfully');
const imagePaths = ['path/to/image1.png', 'path/to/image2.png'];
for (const imagePath of imagePaths) {
console.log(`Processing image: ${imagePath}`);
console.time('OCR Process');
const result = await ocr.readText(imagePath);
console.timeEnd('OCR Process');
console.log('OCR Result:');
result.forEach((item, index) => {
console.log(`Line ${index + 1}:`);
console.log(` Text: ${item.text}`);
console.log(` Confidence: ${(item.confidence * 100).toFixed(2)}%`);
console.log(` Bounding Box: ${JSON.stringify(item.bbox)}`);
console.log('---');
});
}
} catch (error) {
console.error('OCR Error:', error.message);
} finally {
await ocr.close();
}
}
main();
For more examples, please check the examples/basic
directory in the project repository.
EasyOCR
The main class for interacting with EasyOCR.
init(languages: string[] = ['en']): Promise<void>
Initializes the OCR reader with the specified languages.
languages
: Array of language codes to use for OCR. Defaults to ['en']
(English).readText(imagePath: string): Promise<OCRResult[]>
Performs OCR on the specified image.
imagePath
: Path to the image file.Returns a Promise that resolves to an array of OCRResult
objects:
interface OCRResult {
bbox: number[][];
text: string;
confidence: number;
}
close(): Promise<void>
Closes the OCR reader and releases resources.
This project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! Please feel free to submit a Pull Request.
You can reach out to the maintainer on X (Twitter): @saidbyvj
If you find a bug or have a suggestion, please file an issue on the GitHub repository.
FAQs
A Node.js wrapper for the Python EasyOCR library
The npm package node-easyocr receives a total of 955 weekly downloads. As such, node-easyocr popularity was classified as not popular.
We found that node-easyocr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We’ve redesigned the Socket dashboard with simpler navigation, less visual clutter, and a cleaner UI that highlights what really matters.
Industry Insights
Terry O’Daniel, Head of Security at Amplitude, shares insights on building high-impact security teams, aligning with engineering, and why AI gives defenders a fighting chance.
Security News
MCP spec updated with structured tool output, stronger OAuth 2.1 security, resource indicators, and protocol cleanups for safer, more reliable AI workflows.