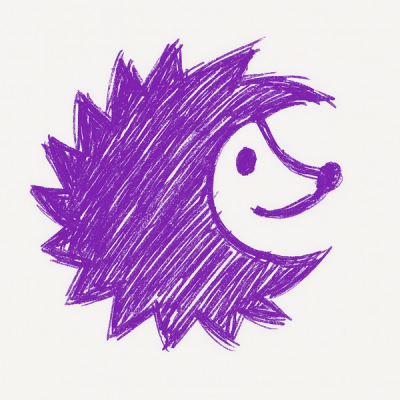
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
node-fetch-commonjs
Advanced tools
The node-fetch-commonjs package is a CommonJS version of the popular node-fetch library, which allows you to make HTTP requests in a Node.js environment. It provides a simple and familiar API for making network requests, similar to the Fetch API available in browsers.
Basic GET Request
This feature allows you to make a basic GET request to a specified URL and handle the response. The example fetches a post from a placeholder API and logs the response data.
const fetch = require('node-fetch-commonjs');
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
POST Request with JSON Body
This feature allows you to make a POST request with a JSON body. The example sends a new post to a placeholder API and logs the response data.
const fetch = require('node-fetch-commonjs');
const data = { title: 'foo', body: 'bar', userId: 1 };
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Handling Headers
This feature allows you to include custom headers in your requests. The example fetches a post from a placeholder API with an Authorization header.
const fetch = require('node-fetch-commonjs');
fetch('https://jsonplaceholder.typicode.com/posts/1', {
headers: { 'Authorization': 'Bearer token' }
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more feature-rich API compared to node-fetch-commonjs, including support for request and response interceptors, automatic JSON data transformation, and more.
Got is a human-friendly and powerful HTTP request library for Node.js. It offers a more modern and flexible API compared to node-fetch-commonjs, with features like retry mechanisms, advanced error handling, and support for streams.
Superagent is a small, progressive client-side HTTP request library, and Node.js module with a simple API. It provides a more flexible and chainable API compared to node-fetch-commonjs, making it easier to build complex requests.
node-fetch but in CommonJS format. This module is built from node-fetch
directly.
require("node-fetch-commonjs")
directly.ExperimentalWarning: stream/web is an experimental feature
warning.node:
prefix.yarn
./build.js # Output to `lib` folder
yarn add node-fetch-commonjs
FAQs
A light-weight module that brings Fetch API to node.js
The npm package node-fetch-commonjs receives a total of 509,867 weekly downloads. As such, node-fetch-commonjs popularity was classified as popular.
We found that node-fetch-commonjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.