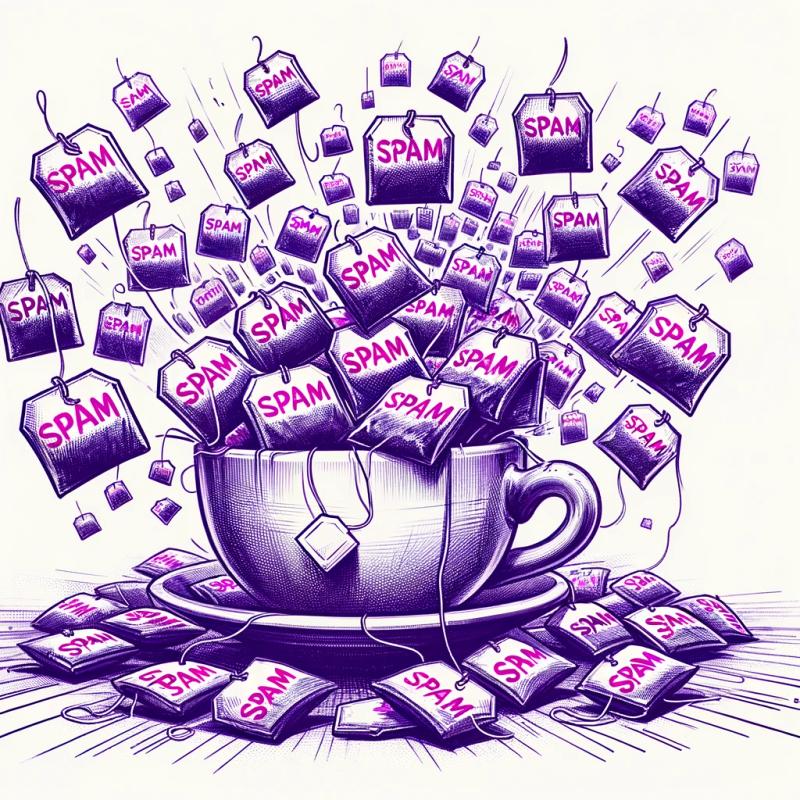
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
node-http-xhr
Advanced tools
Readme
node-http-xhr
An implementation of XMLHttpRequest
for node.js
using
the http.request
API.
This package was written to provide the XMLHttpRequest
API to test browser
code that is being tested in a node.js
environment.
npm install --save node-http-xhr
// Standalone usage
var XMLHttpRequest = require('node-http-xhr');
// Usage as global XHR constructor
global.XMLHttpRequest = require('node-http-xhr');
var req = new XMLHttpRequest();
// Event handlers via .on${event} properties:
req.onreadystatechange = function() {
console.log('readyState: ' + req.readyState);
};
// or using .addEventListener(event, handler):
req.addEventListener('load', function() {
console.log('response: ' + req.response);
});
req.open('GET', 'https://github.com/aspyrx', true);
req.send();
If you use a bundler like browserify
or webpack
that follows the browser
field in package.json
, the module will simply export window.XMLHttpRequest
.
This is provided for sake of compatibility.
To generate documentation:
npm run doc
The html
documentation will be placed in doc/
.
npm test
Currently, some features are lacking:
loadstart
, loadend
, progress
)responseType
values other than ''
or 'text'
and corresponding parsing
overrideMimeType()
isn't very usefulsetRequestHeader()
doesn't check for forbidden headers.withCredentials
is defined as an instance property, but doesn't do anything
since there's no use case for CORS-like requests in node.js
right now.FAQs
Node.js XMLHttpRequest implementation using http.request()
The npm package node-http-xhr receives a total of 6,998 weekly downloads. As such, node-http-xhr popularity was classified as popular.
We found that node-http-xhr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.