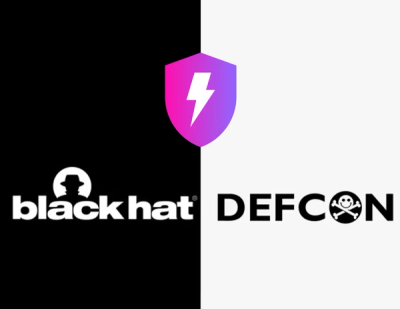
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
node-red-ts
Advanced tools
This project was born because I hate the overly complex and nested structure of the Node-RED API. I wanted something easy and clean and typescript-based.
This is how a simply node looks like:
import { AbstractNode } from "@redts/api/AbstractNode";
import { NodeAPI, NodeMessage } from "node-red";
class DummyNode extends AbstractNode<NodeMessage> {
public constructor(RED: NodeAPI) {
super(RED);
}
public override async onInput(msg?: NodeMessage): Promise<NodeMessage[]> {
console.debug("input");
this.status = "";
return [{ payload: "dummy test" }];
}
public override async onClose(removed: boolean): Promise<void> {
console.debug('input');
this.status = '';
}
}
module.exports = (RED: NodeAPI) => AbstractNode.createNode(RED, DummyNode);
The corresponding HTML file looks a little bit more complex though:
<!-- definition -->
<script type="text/javascript">
RED.nodes.registerType("DummyNode", {
category: "HHLA",
color: "#1eb3fd",
icon: "font-awesome/fa-cogs",
defaults: {
name: { value: "" },
},
inputs: 1,
outputs: 1,
outputLabels: [
"summary",
],
label: function () {
return this.name || "Dummy"
},
paletteLabel: "Dummy",
oneditprepare: () => {
}
});
</script>
<!-- edit dialog -->
<script type="text/html" data-template-name="DummyNode">
<!-- name -->
<div class="form-row">
<label for="node-input-name"><i class="fa fa-tag"></i> Name</label>
<input type="text" id="node-input-name" placeholder="Name" />
</div>
</script>
<!-- help text -->
<script type="text/html" data-help-name="DummyNode">
<p>
</p>
</script>
The HTML structure is based on the Node-RED API. Ideally this would be generated from a type-safe representation, maybe a TS class or another well defined structural language.
The node files are in the "src/nodes" folder of this project.
The project itself is runnable in node-red and fully debuggable in vscode (launch config and all is included).
It looks like this:
A quick-starter template can be found here: https://github.com/mojo2012/node-red-ts-template. Just check it out and run it.
The AbstractNode
has a bunch of methods that can be used or overriden in your node, for example:
onInput
onClose
setProperty
/getProperty
setStatus
context
flowContext
globalContext
The onInput
method is triggered when a message arrives. It returns an array of messages (in the most cases just one). Each message in the array is passed to one output. The number of outputs has to be configured in the HTML file though.
Create new nodes using this command node node_modules/node-red-ts/tools/create-node.js
. This command will create a new node (html and ts file) in the src/nodes
folder and also register the node in the package.json
.
You can then build and launch node-red and start coding on your new node.
AbstractNode
FAQs
This is a typescript API for developing node-red packages
The npm package node-red-ts receives a total of 0 weekly downloads. As such, node-red-ts popularity was classified as not popular.
We found that node-red-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.