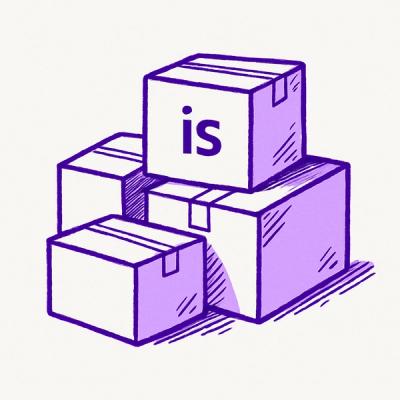
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Nookies is a simple utility for handling cookies in Next.js applications. It provides a straightforward API for setting, getting, and destroying cookies on both the client and server sides.
Set a Cookie
This feature allows you to set a cookie in a Next.js application. The code sample demonstrates how to set a cookie named 'cookieName' with a value of 'cookieValue' that expires in 30 days.
const nookies = require('nookies');
// In a Next.js API route or getServerSideProps
export function handler(req, res) {
nookies.set({ res }, 'cookieName', 'cookieValue', {
maxAge: 30 * 24 * 60 * 60,
path: '/',
});
res.end('Cookie set');
}
Get a Cookie
This feature allows you to retrieve a cookie in a Next.js application. The code sample demonstrates how to get the value of a cookie named 'cookieName'.
const nookies = require('nookies');
// In a Next.js API route or getServerSideProps
export function handler(req, res) {
const cookies = nookies.get({ req });
res.end(`Cookie value: ${cookies.cookieName}`);
}
Destroy a Cookie
This feature allows you to destroy a cookie in a Next.js application. The code sample demonstrates how to destroy a cookie named 'cookieName'.
const nookies = require('nookies');
// In a Next.js API route or getServerSideProps
export function handler(req, res) {
nookies.destroy({ res }, 'cookieName');
res.end('Cookie destroyed');
}
The 'cookie' package is a simple, lightweight library for parsing and serializing cookies. It is more general-purpose and can be used in any Node.js application, not just Next.js. However, it does not provide the same level of integration with Next.js as nookies.
The 'js-cookie' package is a popular library for handling cookies in the browser. It provides a simple API for setting, getting, and removing cookies on the client side. Unlike nookies, it does not support server-side operations, making it less suitable for Next.js applications that require server-side cookie handling.
The 'universal-cookie' package is designed to work in both browser and Node.js environments. It provides a consistent API for handling cookies on both the client and server sides. While it offers similar functionality to nookies, it is not specifically tailored for Next.js applications.
A collection of cookie helpers for Next.js
Setting and destroying cookies also works on server-side.
yarn add nookies
You can play with the example code here.
import nookies from 'nookies'
export default function Me() {
return <div>My profile</div>
}
export async function getServerSideProps(ctx) {
// Parse
const cookies = nookies.get(ctx)
// Set
nookies.set(ctx, 'fromGetInitialProps', 'value', {
maxAge: 30 * 24 * 60 * 60,
path: '/',
})
// Destroy
// nookies.destroy(ctx, 'cookieName')
return { cookies }
}
import { parseCookies, setCookie, destroyCookie } from 'nookies'
function handleClick() {
// Simply omit context parameter.
// Parse
const cookies = parseCookies()
console.log({ cookies })
// Set
setCookie(null, 'fromClient', 'value', {
maxAge: 30 * 24 * 60 * 60,
path: '/',
})
// Destroy
// destroyCookie(null, 'cookieName')
}
export default function Me() {
return <button onClick={handleClick}>Set Cookie</button>
}
const express = require('express');
const dev = process.env.NODE_ENV !== 'production';
const app = next({ dev });
const handle = app.getRequestHandler();
const { parseCookies, setCookie, destroyCookie } = require('nookies');
app.prepare()
.then(() => {
const server = express();
server.get('/page', (req, res) => {
// Notice how the request object is passed
const parsedCookies = parseCookies({ req });
// Notice how the response object is passed
setCookie({ res }, 'fromServer', 'value', {
maxAge: 30 * 24 * 60 * 60,
path: '/page',
});
// destroyCookie({ res }, 'fromServer');
return handle(req, res);
});
);
For client side usage, omit the
ctx
parameter. You can do so by setting it to an empty object ({}
),null
orundefined
.
parseCookies(ctx, options)
or nookies.get(ctx, options)
Next.js context
|| (Express request object)
a custom resolver function (default: decodeURIComponent)
setCookie(ctx, name, value, options)
or nookies.set(ctx, name, value, options)
Don't forget to end your response on the server with
res.send()
.
(Next.js context)
|| (Express request object)
destroyCookie(ctx, name, options)
or nookies.destroy(ctx, 'token', options)
Don't forget to end your response on the server with
res.send()
. This might be the reason your cookie isn't removed.
(Next.js context)
|| (Express response object)
MIT
FAQs
A set of cookie helpers for Next.js
The npm package nookies receives a total of 132,107 weekly downloads. As such, nookies popularity was classified as popular.
We found that nookies demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.