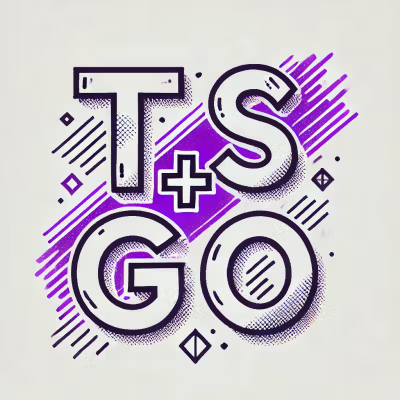
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
npf2html
A simple zero-dependency package for converting Tumblr's Neue Post Format to standard HTML.
This is recommended for use along with tumblr.js, which can interact with the Tumblr API to get the NPF for Tumblr posts.
This module exports a primary entrypoint function, npf2html
, which takes a
list of content blocks as provided by the Tumblr API's post.content
and
returns it as HTML. It's also recommended you pass in post.layout
and
post.asking_avatar
as options to accurately reproduce the structure of the
post.
import npf2html from 'npf2html';
// or for CJS:
// const npf2html = require('npf2html');
import * as tumblr from "tumblr.js";
const client = new tumblr.Client({
consumer_key: "<your key goes here>",
});
const response = await client.blogPosts("the-lonelyshepherd", {
id: "751572408377507840",
npf: true
});
const post = response.posts[0];
console.log(npf2html(post.content, {
layout: post.layout,
askingAvatar: post.asking_avatar,
}));
This package's goal is not to produce the same HTML as the Tumblr website itself. Instead, it aims to produce reasonably clean, semantic, easy-to-style HTML while following the general presentation guidelines for Tumblr API clients.
In many situations, the output will include npf-...
classes to make it easier
to style different blocks. However, in cases where the meaning of a given
element is always clear from the structure of the HTML, classes may be omitted
for cleaner output.
Various components may be omitted, depending on what's sent down by the server.
Most audio blocks look like:
<figure class="npf-block-audio">
<audio controls src="..."></audio>
<figcaption>
<img src="..."> <!-- the "poster" for the audio track -->
<span class="npf-block-audio-title">...</span> -
<span class="npf-block-audio-artist">...</span> on
<span class="npf-block-audio-album">...</span>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
However, audio may also be represented as a plain link:
<figure class="npf-block-audio">
<a href="...">
<img src="..."> <!-- the "poster" for the audio track -->
<span class="npf-block-audio-title">...</span> -
<span class="npf-block-audio-artist">...</span> on
<span class="npf-block-audio-album">...</span>
</a>
<figcaption>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
Or as an iframe embed:
<figure class="npf-block-audio">
<iframe src="...."></iframe>
<figcaption>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
Image blocks look like:
<figure class="npf-block-image">
<a href="...">
<img src="...">
</a>
<figcaption>
<span class="npf-block-image-caption">...</span>
<a class="npf-attribution" href="...">blogname</a>
</figcaption>
</figure>
Link blocks look like:
<a class="npf-block-link" href="...">
<img src="..."> <!-- the "poster" for the link -->
<h2>...</h2> <!-- the link title, or URL if title is unavailable -->
<p class="npf-block-link-site">...</p>
<p class="npf-block-link-author">...</p>
<p class="npf-block-link-description">...</p>
</a>
Paywall blocks look like:
<a class="npf-block-paywall ..." href="...">
<h2>...</h2> <!-- paywall title -->
<p>...</p> <!-- paywall description or label -->
</a>
There are three types of paywall:
An unpaid paywall has the class npf-block-paywall-cta
, and has both a title
and description.
A disabled paywall has the class npf-block-paywall-disabled
, and has both a
title and description.
A paywall for which the viewer has paid has the class
npf-block-paywall-divider
, and has only text, no title. It may also have a
--npf-paywall-color
CSS variable set with the divider color.
Poll blocks look like:
<div class="npf-block-poll">
<h2>...</h2> <!-- poll question -->
<ul>
<li>...</li> <!-- poll option -->
...
</ul>
</div>
Polls can't be interacted with outside the Tumblr web interface, so this doesn't include any interactive elements.
Text blocks are rendered either as <h1>
s, <h2>
s, <blockquote>
s, <ul>
s,
<li>
s, or <p>
s. The latter may have one of the following classes:
npf-block-text-quirky
indicates text that's rendered in a large cursive
font.
npf-block-text-quote
indicates text that's rendered in a large serif font.
npf-block-text-chat
indicates text that's rendered in a monospace font.
Text blocks also support the following inline formatting:
Plain <strong>
, <em>
, <small>
, <s>
, and <a>
tags.
<a class="npf-inline-mention">
tags, which indicate mentions of other blogs.
<span style="color: ...">
for colored text.
Note: Tumblr assumes all text is rendered with whitespace preserved but text
wrapped, equivalent to white-space: pre-wrap
in CSS. If you don't use this
style, it's likely that some posts won't render as the authors intended.
Most video blocks look like:
<figure class="npf-block-video">
<video src="..." poster="..."></video>
<figcaption>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
However, video may also be represented as a plain link:
<figure class="npf-block-video">
<a href="...">...</a>
<figcaption>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
Or as an iframe embed:
<figure class="npf-block-video">
<iframe src="...."></iframe>
<figcaption>
<a class="npf-attribution" href="...">...</a>
</figcaption>
</figure>
Audio, video, and image blocks can all have attributions attached which indicate
where they were sourced. These always appear in <figcaption>
s beneath the
media itself. They're always wrapped in <a class="npf-attribution">
, which can
have the following additional classes:
npf-attribution-post
indicates that the media came from a specific Tumblr
post. The link's text is the blog's name.
npf-attribution-blog
indicates that the media came from a Tumblr blog, but
not a specific post. The link's text is the blog's name.
npf-attribution-link
indicates that the media came from an arbitrary URL.
The link text is the URL itself.
npf-attribution-app
indicates that the media came from a third-party app.
The link may contain an image representing the app's logo, as well as a
text description from the application.
There are a number of layouts that can wrap multiple content blocks.
An ask looks like:
<div class="npf-layout-ask">
<a href="..."><img></a> <!-- asker avatar -->
<figure>
<figcaption>
<a href="..."><strong>...</strong> asked:</a>
</figcaption>
... <!-- content blocks -->
</figure>
</div>
An anonymous ask looks like:
<div class="npf-layout-ask">
<a><img></a> <!-- standard anonymous avatar -->
<figure>
<figcaption>
<strong>Anonyous</strong> asked:
</figcaption>
... <!-- content blocks -->
</figure>
</div>
Note that the avatar still has an <a>
tag despite not having an href
, to
make the styling more consistent with non-anonymous asks.
A row with multiple images looks like:
<div class="npf-layout-row">
... <!-- content blocks -->
</div>
Rows are guaranteed to contain only image blocks. They may have the additional
class npf-layout-row-carousel
if they're expected to display in carousel
display mode.
A truncation, also known as a "read more", looks like:
<details class="npf-layout-truncate">
<summary>Keep reading</summary>
... <!-- content blocks -->
</details>
1.1.6
FAQs
Converts Tumblr's Neue Post Format to plain HTML
The npm package npf2html receives a total of 10 weekly downloads. As such, npf2html popularity was classified as not popular.
We found that npf2html demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.