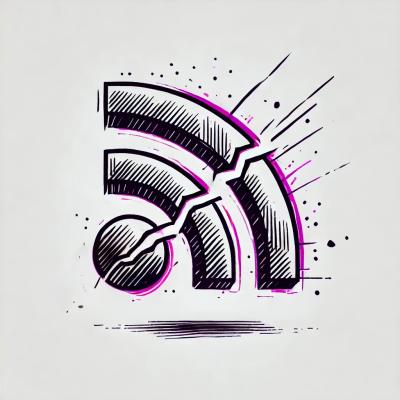
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
A simplified wrapper around Clarifai's NSFW detection.
var NSFAI = require('nsfai');
var nsfai = new NSFAI("YOUR_CLARIFAI_API_KEY_HERE");
function handleResult(result) {
if (result.sfw) {
console.log(`This image is A-OK with a confidence of ${result.confidence}.`);
} else {
console.log(`This image is NSFW with a confidence of ${result.confidence}.`);
}
}
function handleError(error) {
console.error(error);
}
nsfai.predict("https://example.com/example.png").then(handleResult).catch(handleError); // URL
// or //
nsfai.predict("data:image/png;base64,dGhpc2lzbm90YW5pbWFnZQ==").then(handleResult).catch(handleError); // Data URL
// or //
nsfai.predict("dGhpc2lzbm90YW5pbWFnZQ==", handleResult).then(handleResult).catch(handleError); // Base64
After you've created and logged in your account, hover on your name in the top bar, and click on Applications.
Click on Create New Application, give your app a name, and set the base workflow to NSFW (optional). The default language doesn't matter.
After clicking on Create App, head over to the API Keys page in the sidebar.
You can already see an API key, and you could use that, but you should create a new API key with limited permissions, so if your key gets leaked, you can just revoke it, and less damage could be done.
Click on Create new API Key, and select your app in the Apps dropdown. Give your key a name, and select the Predict on Public and Custom Models scope. That's all you need.
Click on Save Changes, and copy your new and shiny API key.
First, you'll need to install NSFAI via NPM. Use the -s
flag, so it gets saved into your package.json
file.
npm install -s nsfai
First, you'll need to require and initialize NSFAI in your code. Use require('nsfai')
, and then, you'll need to create a new NSFAI instance. Here's where your API key comes in.
For security reasons, you shouldn't hardcode your API key into your code, because if you upload it to GitHub, or someone gets the code, they can just read out the key. What we recommend, is storing it in your environment variables. You know, that place where PATH
, and stuff like that is.
Go ahead and save your API key in an environment variable. Now, when you push the code to GitHub, or upload is somewhere, people can't see it.
After you've securely saved your API key into an environment variable, we can create the NSFAI instance. Use new NSFAI(process.env.YOUR_ENVIRONMENT_VARIABLE_NAME_HERE)
for that.
var NSFAI = require('nsfai');
var nsfai = new NSFAI(process.env.MYAPP_CLARIFAI_KEY);
Congratulations! Now you can predict the NSFWness of images. Let's see an example of how to do that!
var NSFAI = require('nsfai');
var nsfai = new NSFAI(process.env.MYAPP_CLARIFAI_KEY);
nsfai.predict("https://example.com/image.png").then(function(result) {
if (result.sfw) { // If the result is safe for work:
console.log(`This image is safe for work, with a confidence of ${result.confidence}!`);
} else { // If the result is not safe for work:
console.log(`This image is not safe for work, with a confidence of ${result.confidence}!`);
}
}).catch(function(error) {
console.error(error); // Print the error to the console.
});
FAQs
A simplified wrapper around Clarifai's NSFW detection.
The npm package nsfai receives a total of 12 weekly downloads. As such, nsfai popularity was classified as not popular.
We found that nsfai demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.