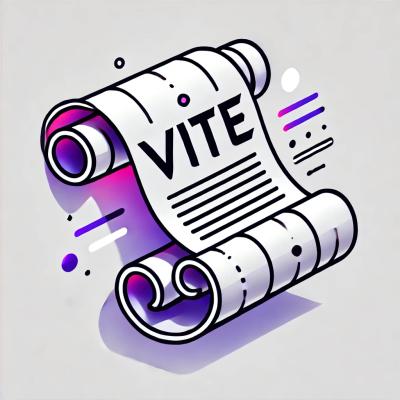
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
The 'once' npm package is a utility that allows you to ensure a function can only be called once. It is useful for preventing duplicate initialization, handling setup tasks that should only run a single time, or ensuring a callback is only executed once in response to an event or the resolution of a Promise.
Ensuring a function is only called once
This feature is used to wrap a function so that it can only be executed once. Subsequent calls to the function will have no effect, and the original return value will be returned.
const once = require('once');
const myFunction = once(() => {
console.log('This will only be logged once.');
});
myFunction(); // logs 'This will only be logged once.'
myFunction(); // does nothing
This is a function from the Lodash library that ensures a given function can only be called once. It is similar to 'once' but comes as part of the larger Lodash utility library, which includes a wide range of functions for different purposes.
Memoizee is a library for memoizing functions, which can also be used to ensure a function is only called once by caching the result of the first call. It is more complex and feature-rich than 'once', offering fine-grained control over cache management and function memoization.
Only call a function once.
var once = require('once')
function load (file, cb) {
cb = once(cb)
loader.load('file')
loader.once('load', cb)
loader.once('error', cb)
}
Or add to the Function.prototype in a responsible way:
// only has to be done once
require('once').proto()
function load (file, cb) {
cb = cb.once()
loader.load('file')
loader.once('load', cb)
loader.once('error', cb)
}
Ironically, the prototype feature makes this module twice as complicated as necessary.
To check whether you function has been called, use fn.called
. Once the
function is called for the first time the return value of the original
function is saved in fn.value
and subsequent calls will continue to
return this value.
var once = require('once')
function load (cb) {
cb = once(cb)
var stream = createStream()
stream.once('data', cb)
stream.once('end', function () {
if (!cb.called) cb(new Error('not found'))
})
}
once.strict(func)
Throw an error if the function is called twice.
Some functions are expected to be called only once. Using once
for them would
potentially hide logical errors.
In the example below, the greet
function has to call the callback only once:
function greet (name, cb) {
// return is missing from the if statement
// when no name is passed, the callback is called twice
if (!name) cb('Hello anonymous')
cb('Hello ' + name)
}
function log (msg) {
console.log(msg)
}
// this will print 'Hello anonymous' but the logical error will be missed
greet(null, once(msg))
// once.strict will print 'Hello anonymous' and throw an error when the callback will be called the second time
greet(null, once.strict(msg))
FAQs
Run a function exactly one time
The npm package once receives a total of 58,130,041 weekly downloads. As such, once popularity was classified as popular.
We found that once demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.