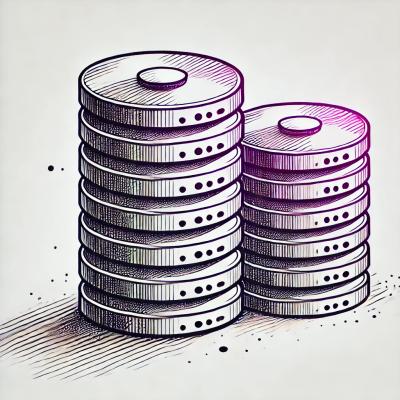
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Orison is a Node.js framework for building server-side rendered, dynamic webapps with React. It is heavily inspired by Next.js but has several key features and differences that make it stand out from the crowd.
It is highly recommended to use yarn
add your package manager. Use npm install --global yarn
to get Yarn, and run yarn install orisonjs
to install Orison.
When getting props for a page, the getServerSideProps
function is called only on the server and it is ignored by Webpack and not sent to the client. This means that you can have information like SQL passwords, queries, server-side only NPM modules, and other sensitive and client-unavailable information in your page file and it's only compiled for the server.
// src/pages/PageWithData.tsx
import { OrisonPage } from 'orisonjs';
interface PageWithDataProps {
elements: {
x: string;
y: string;
}[];
}
const PageWithData: OrisonPage<PageWithDataProps> = ({ elements }) => (
<div>
{elements.map(elem => (
<>
<span>x = {elem.x}</span>
<span>y = {elem.y}</span>
</>
))}
</div>
);
PageWithData.getServerSideProps = async req => {
const sql = await import('../common/sql');
return {
elements: await sql.query('SELECT x, y FROM elements')
};
};
export default PageWithData;
Each user session on an Orison server is assigned a cookie to keep track of their session, and each session has an object you can specify key-value pairs for that are only available server-side, very similar to ASP.NET.
// src/pages/AuthenticatedPageWithData.tsx
import { OrisonPage } from 'orisonjs';
interface AuthenticatedPageWithDataProps {
elements: {
x: string;
y: string;
}[];
}
const AuthenticatedPageWithData: OrisonPage<AuthenticatedPageWithDataProps> = ({ elements }) => (
<div>
{elements.map(elem => (
<>
<span>x = {elem.x}</span>
<span>y = {elem.y}</span>
</>
))}
</div>
);
AuthenticatedPageWithData.getServerSideProps = async (req, res) => {
// req.session field is added by Orison with the data for each user's session
// values can be get and set from both pages and REST endpoints
if(!req.session.isAuthenticated) {
res.redirect('/login'); // if the user isn't logged in, send them to the log in page, skipping the SSR of this page
} else {
const sql = await import('../singletons/sql');
return {
elements: await sql.query('SELECT x, y FROM elements')
};
}
};
export default AuthenticatedPageWithData;
Instead of using the built-in node http
module, Orison uses express
to utilize its powerful middleware functionality. Orison servers are required to have an entrypoint at src/orison.ts
, where you can get direct access to the Express request handler.
// src/orison.ts
import { OrisonServer } from 'orison';
export default async function main(server: OrisonServer) {
const express = server.getRequestListener();
express.use(...); // custom middleware function
await server.configure({
// function to generate a session state object for each new session
sessionStateGenerator: req => ({
isAuthenticated: false
})
});
server.start(3000);
}
FAQs
Full-stack stateful React SSR framework
The npm package orisonjs receives a total of 5 weekly downloads. As such, orisonjs popularity was classified as not popular.
We found that orisonjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.